Introduction In this lab, you are writing a function get_written_date(date_list) that takes as a parameter a list of strings in the [MM, DD, YYYY] format and returns the resulting date (in the US format) as a string. Note: we are using the US format for strings: //. For example, 01/02/2022 can be represented as ['01', '02', *2022'], which represents January 2nd, 2022. Test Your Code assert get_written_date(["01", "01", "1970"]) == "January 1, 1970" assert get_written_date(["02", "03", "2000"]) == "February 3, 2000" assert get_written_date(["10", "15", "2022"]) == "October 15, 2022" assert get_written_date(["12", "31", "2021"]) == "December 31, 2021" Instructions Use the dictionary month_names that maps months to their English names. month_names = { 1: "January", 2: "February", 3: "March" } Note: the month key in the dictionary is stored as an integer, however, in the input list it is stored as a string in the MM format, e.g., "01". Also, pay attention how the day is represented in the list and how it is supposed to be output. • You may assume that the dates in date_list is a valid date. Troubleshooting If you are getting a KeyError: '01' for "01" pay attention to the type of the keys in the dictionary. (See the Note above :-)) If you are having trouble converting a "01" into just 1, think how you would turn just "1" into an integer. ;-) What if you use the same mechanism with "01"?
Please Use Python
def get_written_date(date_list):
"""
The function ...
"""
month_names = {
1: "January",
2: "February",
3: "March",
4: "April",
5: "May",
6: "June",
7: "July",
8: "August",
9: "September",
10: "October",
11: "November",
12: "December",
}
# Finish the function
# Return the date string in written format
if __name__ == "__main__":
assert get_written_date(["01", "02", "2022"]) == 'January 2, 2022'
assert get_written_date(["01", "12", "1970"]) == 'January 12, 1970'
assert get_written_date(["04", "14", "2020"]) == 'April 14, 2020'
assert get_written_date(["06", "19", "2000"]) == 'June 19, 2000'
![Introduction
In this lab, you are writing a function get_written_date(date_list) that takes as a parameter a list of strings in the [MM, DD, YYYY]
format and returns the resulting date (in the US format) as a string.
Note: we are using the US format for strings: <MM>/<DD>/<YEAR>. For example, 01/02/2022 can be represented as ['01', '02',
*2022' ], which represents January 2nd, 2022.
Test Your Code
assert get_written_date(["02",
assert get_written_date(["01", "01", "1970"]) == "January 1, 1970"
"03", "2000"]) == "February 3, 2000"
assert get_written_date(["10", "15", "2022"]) "October 15, 2022"
assert get_written_date(["12", "31", "2021"]) == "December 31, 2021"
Instructions
Use the dictionary month_names that maps months to their English names.
month_names = {
1: "January",
2: "February",
3: "March"
...
==
Note: the month key in the dictionary is stored as an integer, however, in the input list it is stored as a string in the MM format, e.g., "01".
Also, pay attention how the day is represented in the list and how it is supposed to be output.
You may assume that the dates in date_list is a valid date.
Troubleshooting
If you are getting a KeyError: '01' for "01" pay attention to the type of the keys in the dictionary. (See the Note above :-))
If you are having trouble converting a "01" into just 1, think how you would turn just "1" into an integer. ;-) What if you use the same
mechanism with "01"?](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fccac55ee-732b-4607-a38e-f5bd0a8aacb2%2F1f8d84f3-ee31-4142-a74c-8d899e5d1f33%2Fr7hfwxb_processed.png&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

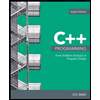
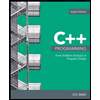