ItemToPurchase.h - Class declaration ItemToPurchase.cpp - Class definition main.cpp - main() function Build the ItemToPurchase class with the following specifications: Default constructor Public class functions (mutators & accessors) setName() & name() setPrice() & price() setQuantity() & quantity() Private data members string _name - Initialized in default constructor to "none" int _price - Initialized in default constructor to 0 int _quantity - Initialized in default constructor to 0
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Create three files:
- ItemToPurchase.h - Class declaration
- ItemToPurchase.cpp - Class definition
- main.cpp - main() function
Build the ItemToPurchase class with the following specifications:
- Default constructor
- Public class functions (mutators & accessors)
- setName() & name()
- setPrice() & price()
- setQuantity() & quantity()
- Private data members
- string _name - Initialized in default constructor to "none"
- int _price - Initialized in default constructor to 0
- int _quantity - Initialized in default constructor to 0
(Step 2) Extend the ItemToPurchase class per the following specifications:
- Parameterized constructor to assign item name, item description, item price, and item quantity (default values of 0).
- Public member functions
- setDescription() mutator & description() accessor
- printItemCost() - Outputs the item name followed by the quantity, price, and subtotal
- printItemDescription() - Outputs the item name and description
- Private data members
- string _description - Initialized in default constructor to "none"
Ex. of printItemCost() output:
Bottled Water 10 @ $1 = $10
Ex of printItemDescription() output:
Bottled Water: Deer Park, 12 oz.
Create two new files:
- ShoppingCart.h - Class declaration
- ShoppingCart.cpp - Class definition
Build the ShoppingCart class with the following specifications. Note: Some can be function stubs (empty functions) initially, to be completed in later steps.
- Default constructor
- Parameterized constructor which takes the customer name and date as parameters
- Private data members
- string _customerName - Initialized in default constructor to "none"
- string _currentDate - Initialized in default constructor to "January 1, 2016"
vector < ItemToPurchase > _cartItems
- Public member functions
- customerName() accessor
- date() accessor
- addItem()
- Adds an item to cart items vector. Has a parameter of type ItemToPurchase. Does not return anything.
- removeItem()
- Removes item from cart items vector. Has a parameter of type string (an item's name). Does not return anything.
- If item name cannot be found, output this message: Item not found in cart. Nothing removed.
- modifyItem()
- Modifies an item's description, price, and/or quantity. Has a parameter of type ItemToPurchase. Does not return anything.
- If item can be found (by name) in cart, check if parameter variable has default values for description, price, and/or quantity. For each of the non-default values in parameter, set the item in the cart to the non-default value. For example, if the parameter has non-default values for quantity and price but a default value for the description, then just set the quantity and price in the cart item to the parameter's values for quantity and price and leave the cart item's description alone.
- If item cannot be found (by name) in cart, output this message: Item not found in cart. Nothing modified.
- numItemsInCart()
- Returns quantity of all items in cart. Has no parameters.
- costOfCart()
- Determines and returns the total cost of items in cart. Has no parameters.
- printTotal()
- Outputs total of objects in cart.
- If cart is empty, output this message: SHOPPING CART IS EMPTY
- printDescriptions()
- Outputs each item's description.
Ex. of printTotal() output:
John Doe's Shopping Cart - February 1, 2016 Number of Items: 8 Nike Romaleos 2 @ $189 = $378 Chocolate Chips 5 @ $3 = $15 Powerbeats 2 Headphones 1 @ $128 = $128 Total: $521
Ex. of printDescriptions() output:
John Doe's Shopping Cart - February 1, 2016 Item Descriptions Nike Romaleos: Volt color, Weightlifting shoes Chocolate Chips: Semi-sweet Powerbeats 2 Headphones: Bluetooth headphones
(Step 4) In main(), prompt the user for a customer's name and today's date. Output the name and date. Create an object of type ShoppingCart.
Ex.
Enter customer's name: John Doe Enter today's date: February 1, 2016 Customer name: John Doe Today's date: February 1, 2016
(Step 5) Implement the printMenu() function as global function (non-member function). printMenu() has a ShoppingCart parameter, and outputs a menu of options to manipulate the shopping cart. Each option is represented by a single character. Build and output the menu within the function.
If the an invalid character is entered, continue to prompt for a valid choice.
Ex:
MENU a - Add item to cart d - Remove item from cart c - Change item quantity i - Output items' descriptions o - Output shopping cart q - Quit Choose an option:
(Step 6) Implement Output shopping cart menu option.
Ex:
OUTPUT SHOPPING CART
John Doe's Shopping Cart - February 1, 2016
Number of Items: 8
Nike Romaleos 2 @ $189 = $378
Chocolate Chips 5 @ $3 = $15
Powerbeats 2 Headphones 1 @ $128 = $128
Total: $521
(Step 7) Implement Output item's description menu option.
Ex.
OUTPUT ITEMS' DESCRIPTIONS
John Doe's Shopping Cart - February 1, 2016
Item Descriptions Nike Romaleos: Volt color, Weightlifting shoes
Chocolate Chips: Semi-sweet
Powerbeats 2 Headphones: Bluetooth headphones
(Step 8) Implement Add item to cart menu option.
(Step 9 ) implement remove item menu option
ex:
REMOVE ITEM FROM CART
Enter name of item to remove:
Chocolate Chips

Step by step
Solved in 3 steps with 1 images

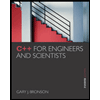
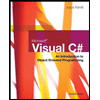
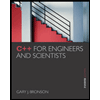
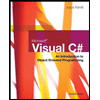