/java Part I: Code an application using the BigDecimalclass to calculate the return of an investment. Use the same formula found in FutureValueand FutureValueApp2in Ch5 from the downloaded source code examples for the calculation. You must format the currency and percent (keeping 3 positions to the right of the decimal point in percent) and handle any exceptions (letter and out of range entries) that may occur from the input data. The valid range of investment amounts is from $1.00 to $1,000,000.00. The valid return rate is from 0.1% to 3.5%. The valid period of investment years is from 1 to 100. Your program must continue to run until the user enters “n” to terminate the execution. You must validate the user's entry so that only “y” and “n” are accepted. Save your code as fileFutureValueBigDeci malApp.java. (Suggestions: Modify Validator so it will add a new validateDoubleWithRange(Scanner sc, String prompt, double min, double max) method and a newvalidateIntWithRange(Scanner, sc, String prompt, int min, int max) method. Modify three instance data monthlyInvestment, yearlyReturnRate, and investYearsas BigDecimal objects and modify FutureValueaccordingly to handle BigDecimalobjects for practice purposes in this lab). Part II: Modify Part I so it will use methods in JOptionPane to handle all input and output data including all data validations described in Part I above. Save your driver code as FutureValueJOptionPaneApp.java. ==================================================== public class FutureValue { private String name; //User name private int years; //investment in years private double monthlyInvest, //monthly investment amount yearlyRate, //annual return rate futureValue = 0.0; //return value //following are setXx() and getXxx() for instance variables public void setName(String userName) { name = userName; } public String getName() { return name; } public void setMonthlyInvest(double monthlyInvestment) { monthlyInvest = monthlyInvestment; } public double getMonthlyInvest() { return monthlyInvest; } public void setYearlyRate(double yearlyReturnRate) { yearlyRate = yearlyReturnRate; } public double getYealyRate() { return yearlyRate; } public void setYears(int investYears){ years = investYears; } public int getYears() { return years; } public double getFutureValue() { return futureValue; } public void futureValueComputing() { //method to compute double monthlyRate = yearlyRate/12/100; int months = years * 12; //convert to months int i = 1; //loop control variable while(i <= months) { futureValue = (futureValue + monthlyInvest) * (1 + monthlyRate); i++; //month increased by 1 } //end of while } //end of method futureValueComputing public void outputResult() { String message = "Your future value report: " + "Name: " + name + "\nMonthly investment: " //build output message + FormattedOutput.currencyOutput(monthlyInvest) + "\nYearly interest rate: " + FormattedOutput.percentOutput(yearlyRate, 2) + "\nInvest years: " + years + "\nFuture return: " + FormattedOutput.currencyOutput(futureValue); System.out.println(message); } } //end of FutureValue class ===================================================== mport java.util.Scanner; public class FutureValueApp2 { public static void main(String[] args) { String choice = "y", //loop control initial value userName; while(choice.equalsIgnoreCase("y")) { FutureValue futureValue = new FutureValue(); //create an object of FutureValue Scanner sc = new Scanner(System.in); System.out.println("Welcome to future value application!\n\n"); System.out.print("Please enter your name: "); userName = sc.next(); //get name futureValue.setName(userName); //set user name System.out.println(); double investAmount = Validator.validateDoubleWithRange(sc, "enter your monthly invest: ", 0, 1000000); //verify data futureValue.setMonthlyInvest(investAmount); //set monthly invest double interestRate = Validator.validateDoubleWithRange(sc, "enter your yearly interest rate: ", 0, 35); //verify data futureValue.setYearlyRate(interestRate); //set yearly rate int years = Validator.validateIntWithRange(sc, "enter number of years: ", 0, 130); //verify data futureValue.setYears(years); futureValue.futureValueComputing(); //calculate futureValue.outputResult(); //formatted output choice = Validator.validateYN(sc, "continue? (y/n): "); //ask for continue or no
//java
Part I: Code an application using the BigDecimalclass to calculate the return of an investment. Use the same formula found in FutureValueand FutureValueApp2in Ch5 from the downloaded source code examples for the calculation. You must format the currency and percent (keeping 3 positions to the right of the decimal point in percent) and handle any exceptions (letter and out of range entries) that may occur from the input data. The valid range of investment amounts is from $1.00 to $1,000,000.00. The valid return rate is from 0.1% to 3.5%. The valid period of investment years is from 1 to 100. Your program must continue to run until the user enters “n” to terminate the execution. You must validate the user's entry so that only “y” and “n” are accepted. Save your code as fileFutureValueBigDeci malApp.java. (Suggestions: Modify Validator so it will add a new validateDoubleWithRange(Scanner sc, String prompt, double min, double max) method and a newvalidateIntWithRange(Scanner, sc, String prompt, int min, int max) method. Modify three instance data monthlyInvestment, yearlyReturnRate, and investYearsas BigDecimal objects and modify FutureValueaccordingly to handle BigDecimalobjects for practice purposes in this lab).
Part II: Modify Part I so it will use methods in JOptionPane to handle all input and output data including all data validations described in Part I above. Save your driver code as FutureValueJOptionPaneApp.java.
====================================================
public class FutureValue {
private String name; //User name
private int years; //investment in years
private double monthlyInvest, //monthly investment amount
yearlyRate, //annual return rate
futureValue = 0.0; //return value
//following are setXx() and getXxx() for instance variables
public void setName(String userName) {
name = userName;
}
public String getName() {
return name;
}
public void setMonthlyInvest(double monthlyInvestment) {
monthlyInvest = monthlyInvestment;
}
public double getMonthlyInvest() {
return monthlyInvest;
}
public void setYearlyRate(double yearlyReturnRate) {
yearlyRate = yearlyReturnRate;
}
public double getYealyRate() {
return yearlyRate;
}
public void setYears(int investYears){
years = investYears;
}
public int getYears() {
return years;
}
public double getFutureValue() {
return futureValue;
}
public void futureValueComputing() { //method to compute
double monthlyRate = yearlyRate/12/100;
int months = years * 12; //convert to months
int i = 1; //loop control variable
while(i <= months) {
futureValue = (futureValue + monthlyInvest) *
(1 + monthlyRate);
i++; //month increased by 1
} //end of while
} //end of method futureValueComputing
public void outputResult() {
String message = "Your future value report: "
+ "Name: " + name + "\nMonthly investment: " //build output message
+ FormattedOutput.currencyOutput(monthlyInvest)
+ "\nYearly interest rate: "
+ FormattedOutput.percentOutput(yearlyRate, 2)
+ "\nInvest years: " + years
+ "\nFuture return: " + FormattedOutput.currencyOutput(futureValue);
System.out.println(message);
}
} //end of FutureValue class
=====================================================
mport java.util.Scanner;
public class FutureValueApp2 {
public static void main(String[] args) {
String choice = "y", //loop control initial value
userName;
while(choice.equalsIgnoreCase("y")) {
FutureValue futureValue = new FutureValue(); //create an object of FutureValue
Scanner sc = new Scanner(System.in);
System.out.println("Welcome to future value application!\n\n");
System.out.print("Please enter your name: ");
userName = sc.next(); //get name
futureValue.setName(userName); //set user name
System.out.println();
double investAmount = Validator.validateDoubleWithRange(sc, "enter your monthly invest: ", 0, 1000000); //verify data
futureValue.setMonthlyInvest(investAmount); //set monthly invest
double interestRate = Validator.validateDoubleWithRange(sc, "enter your yearly interest rate: ", 0, 35); //verify data
futureValue.setYearlyRate(interestRate); //set yearly rate
int years = Validator.validateIntWithRange(sc, "enter number of years: ", 0, 130); //verify data
futureValue.setYears(years);
futureValue.futureValueComputing(); //calculate
futureValue.outputResult(); //formatted output
choice = Validator.validateYN(sc, "continue? (y/n): "); //ask for continue or no
} //end of while
System.out.println("\nThank you for using future value application.");
} //end of main()
} //end of TestJOptionPaneApp

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

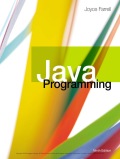
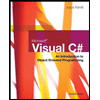
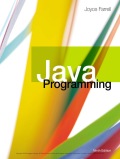
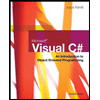