JAVA PROGRAM Chapter 7. PC #1. Rainfall Class Write a RainFall class that stores the total rainfall for each of 12 months into an array of doubles. The program should have methods that return the following: • the total rainfall for the year • the average monthly rainfall • the month with the most rain • the month with the least rain Demonstrate the class in a complete program. Main class name: RainFall (no package name) HERE IS A WORKING CODE, PLEASE MODIFY THIS CODE SO THE PUBLIC CLASS IS MAIN AND WERE EVER THERE IS MAIN IN THE CHANGE IT SO IT RUNS. ALSO, MAKE SURE WHEN I UPLOAD THE CODE TO HYPERGRADE IT PASSES ALL THE TEST CASES. THANK YOU
JAVA PROGRAM
import java.util.Scanner;
public class Main {
private double[] rainfall = new double[12];
public Main(double[] rainfall) {
this.rainfall = rainfall;
}
public double getTotalRainfall() {
double total = 0.0;
for(double rain : rainfall) {
total += rain;
}
return total;
}
public double getAverageMonthlyRainfall() {
return getTotalRainfall() / 12;
}
public int getMonthWithMostRain() {
int month = 0;
double max = rainfall[0];
for(int i = 1; i < rainfall.length; i++) {
if(rainfall[i] > max) {
max = rainfall[i];
month = i;
}
}
return month + 1;
}
public int getMonthWithLeastRain() {
int month = 0;
double min = rainfall[0];
for(int i = 1; i < rainfall.length; i++) {
if(rainfall[i] < min) {
min = rainfall[i];
month = i;
}
}
return month + 1;
}
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
double[] monthlyRainfall = new double[12];
for(int i = 0; i < 12; i++) {
System.out.print("Enter the rainfall amount for month " + (i + 1) + ":\n");
monthlyRainfall[i] = input.nextDouble();
}
Main rf = new Main(monthlyRainfall);
System.out.println("Maximum rainfall: " + monthlyRainfall[rf.getMonthWithMostRain() - 1]);
System.out.println("Minimum rainfall: " + monthlyRainfall[rf.getMonthWithLeastRain() - 1]);
System.out.println("Total rainfall: " + rf.getTotalRainfall());
System.out.printf("Average rainfall: %.1f\n", rf.getAverageMonthlyRainfall());
}
}
Test Case 1
1.2ENTER
Enter the rainfall amount for month 2:\n
2.3ENTER
Enter the rainfall amount for month 3:\n
3.4ENTER
Enter the rainfall amount for month 4:\n
5.1ENTER
Enter the rainfall amount for month 5:\n
1.7ENTER
Enter the rainfall amount for month 6:\n
6.5ENTER
Enter the rainfall amount for month 7:\n
2.5ENTER
Enter the rainfall amount for month 8:\n
3.3ENTER
Enter the rainfall amount for month 9:\n
1.1ENTER
Enter the rainfall amount for month 10:\n
5.5ENTER
Enter the rainfall amount for month 11:\n
6.6ENTER
Enter the rainfall amount for month 12:\n
6.0ENTER
Maximum rainfall: 6.6\n
Minimum rainfall: 1.1\n
Total rainfall: 45.2\n
Average rainfall: 3.8\n

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

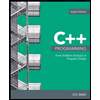
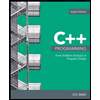