java.io.BufferedReader; import java.io.FileReader;
public List<String> getLikes(String user)
This will take a String representing a user (like “Mike”) and return a unique List containing all of the users that have liked the user “Mike.”
public List<String> getLikedBy(String user)
This will take a String representing a user (like “Tony”) and return a unique List containing each user that “Tony” has liked.
create a Main to test your work.
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
public class FacebookLikeManager {
public List<String> facebookMap;
private Set<String> likesSets;
public FacebookLikeManager() {
facebookMap = new ArrayList<>();
likesSets = new HashSet<>(Arrays.asList("Mike","Kristen","Bill","Sara"));
}
public void buildMap(String filePath) {
try (BufferedReader reader = new BufferedReader(new FileReader(filePath))) {
String line = reader.readLine();
while (line != null) {
String[] lineWords = line.split("[\\s,%$]+");
for (String word : lineWords) {
String cleanWord = word.replaceAll("[^A-Za-z]", "").toUpperCase();
facebookMap.add(cleanWord);
}
line = reader.readLine();
}
} catch (IOException ex) {
System.err.format("IOException: %s%n", ex);
}
}
public List<String> getAllUsers(){
List<String> userList = new ArrayList<>();
Set<String> users = new HashSet<>();
for(String usersName: facebookMap){
users.add(usersName);
}
userList.addAll(users);
return userList;
}
public List<String> getLikes(String user) {
}
public List<String> getLikedBy(String user) {
}
}
public class Main {
public static void main(String[] args) {
FacebookLikeManager main = new FacebookLikeManager();
main.buildMap(args[0]);
System.out.println(main.getAllUsers());
}
}
users.txt
Mike: Kristen, Sara, Nate, Anthony, Randy
Kristen: Mike, John, Steve, Bill
Bill: Sara, Nate
Sara: Nate, Anthony

To implement the getLikes
and getLikedBy
methods, you can use a HashMap
to keep track of the likes of each user. The keys of the HashMap
would be the users, and the values would be a Set
of the users that each user has liked.
Step by step
Solved in 3 steps

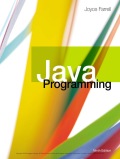
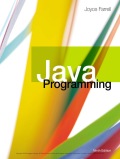