list. entry will be the list created for the second dictionary, and so on.) eated for the first dictionary; the second entry Each entry in the returned list will contain a list of values from that dictionary. The second parameter's entries specify the keys whose values should be added to this list. The order of these values must parallel the order of the keys in the second parameter. (So first will be the value associated with the second parameter's first entry, second will be the value associated with the second parameter's second entry, and so on.) You SHOULD assume that every dictionary will contain all of the keys from the first parameter. Python creates a new list only when a variable is assigned a list literal. You MUST include the statement initializing the variable to which the dictionary's values are added INSIDE the for-loop iterating through the first parameter. As an example, if this were a function call: list_gen ([{'Used Key': 'Data', 'Unused Keys': 'More Data'}], ['Used Key']) the expected return value would be: [ ['Data']] As an second example, if this were a function call: list_gen ([{'Hint': 'Length 2 Not Required', 'Num': 8675309), {'Num': 1, 'Hint': 'Use 1st param order'}], ['Hint', 'Num']) the expected return value would be: [['Length 2 Not Required', 8675309], ['Use 1st param order', 1] I Page 3/ +
list. entry will be the list created for the second dictionary, and so on.) eated for the first dictionary; the second entry Each entry in the returned list will contain a list of values from that dictionary. The second parameter's entries specify the keys whose values should be added to this list. The order of these values must parallel the order of the keys in the second parameter. (So first will be the value associated with the second parameter's first entry, second will be the value associated with the second parameter's second entry, and so on.) You SHOULD assume that every dictionary will contain all of the keys from the first parameter. Python creates a new list only when a variable is assigned a list literal. You MUST include the statement initializing the variable to which the dictionary's values are added INSIDE the for-loop iterating through the first parameter. As an example, if this were a function call: list_gen ([{'Used Key': 'Data', 'Unused Keys': 'More Data'}], ['Used Key']) the expected return value would be: [ ['Data']] As an second example, if this were a function call: list_gen ([{'Hint': 'Length 2 Not Required', 'Num': 8675309), {'Num': 1, 'Hint': 'Use 1st param order'}], ['Hint', 'Num']) the expected return value would be: [['Length 2 Not Required', 8675309], ['Use 1st param order', 1] I Page 3/ +
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter7: Arrays
Section7.5: Case Studies
Problem 3E
Related questions
Question
100%
so im having an issue writing this code. the question, plus the errors are shared in screenshots. please, please, if an expert can help me correct this, id immensely appreciate it
def list_gen(d, l):
result = []
for k in d:
x = []
sorted_dict = dict()
sorted_list = list((i, k[i]) for i in l)
for i in sorted_list:
sorted_dict.setdefault(i[0], i[1])
for key, value in sorted_dict.items():
if key in l:
x.append(value)
result.append(x)
return result
![ERROR when running test_list_gen_empty: Python crashed running list_gen because:
local variable 'sorted_list' referenced before assignment
PASSED
test_list_gen_extra_keys:
I called list_gen ([{"case_number": "22-1660390", "incident_datetime": "2022-06-15T10:51:48.
expecting the result to be [["10"]],
and it was!
FAILED test_list_gen_extra_keys:
I called list_gen ([{"case_number": "22-1650418", "incident_datetime": "2022-06-14T11:45:48.
expecting the result to be [["2022-06-14T11:45:48.000", "Tuesday"], ["2022-06-19T14:06:00.0
but your function returned [["2022-06-15T10:51:48.000", "Wednesday"], ["2022-06-15T10:51:48
FAILED test_list_gen_multi_entries:
I called list_gen ([{"hour_of_day": "23"}, {"hour_of_day": "8"}], ["hour_of_day"])
expecting the result to be [["23"], ["8"]],
but your function returned [["8"]].
FAILED test_list_gen_random_key_order:
I called list_gen([{"incident_datetime": "2022-08-03T13:30:00.000", "day_of_week": "Wednesd
expecting the result to be [["2022-08-03T13:30:00.000", "Buffalo Police are investigating t
but your function returned [["2022-08-03T13:30:00.000", "Buffalo Police are investigating t
PASSED test_list_gen_single_entry:
I called list_gen([{"incident_description": "Buffalo Police are investigating this report o
expecting the result to be [["Buffalo Police are investigating this report of a crime. It
and it was!
FAILED test_list_gen_single_entry:
I called list gen([{"day of week": "Friday", "incident datetime": "2022-04-22T01:31:35.000"](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F89b7b4a3-edc3-4856-b3b8-970ee403a9a8%2F3265cf23-c6fb-4c6a-b9be-c0446bd50d89%2Fl3ezsv_processed.png&w=3840&q=75)
Transcribed Image Text:ERROR when running test_list_gen_empty: Python crashed running list_gen because:
local variable 'sorted_list' referenced before assignment
PASSED
test_list_gen_extra_keys:
I called list_gen ([{"case_number": "22-1660390", "incident_datetime": "2022-06-15T10:51:48.
expecting the result to be [["10"]],
and it was!
FAILED test_list_gen_extra_keys:
I called list_gen ([{"case_number": "22-1650418", "incident_datetime": "2022-06-14T11:45:48.
expecting the result to be [["2022-06-14T11:45:48.000", "Tuesday"], ["2022-06-19T14:06:00.0
but your function returned [["2022-06-15T10:51:48.000", "Wednesday"], ["2022-06-15T10:51:48
FAILED test_list_gen_multi_entries:
I called list_gen ([{"hour_of_day": "23"}, {"hour_of_day": "8"}], ["hour_of_day"])
expecting the result to be [["23"], ["8"]],
but your function returned [["8"]].
FAILED test_list_gen_random_key_order:
I called list_gen([{"incident_datetime": "2022-08-03T13:30:00.000", "day_of_week": "Wednesd
expecting the result to be [["2022-08-03T13:30:00.000", "Buffalo Police are investigating t
but your function returned [["2022-08-03T13:30:00.000", "Buffalo Police are investigating t
PASSED test_list_gen_single_entry:
I called list_gen([{"incident_description": "Buffalo Police are investigating this report o
expecting the result to be [["Buffalo Police are investigating this report of a crime. It
and it was!
FAILED test_list_gen_single_entry:
I called list gen([{"day of week": "Friday", "incident datetime": "2022-04-22T01:31:35.000"
![list_gen
Define a function named is open with Google Paraineters. The first parameter will be a list of
dictionaries. The second parameter will be a list of strings (dictionary keys)..
The function needs to return a list of lists. The length of the returned list must be identical to the
length of the first parameter. The order of these dictionaries will be paralleled in the entries in
the returned list. (So the first entry will be the list created for the first dictionary; the second entry
will be the list created for the second dictionary, and so on.)
Each entry in the returned list will contain a list of values from that dictionary. The second
parameter's entries specify the keys whose values should be added to this list. The order of
these values must parallel the order of the keys in the second parameter. (So first will be the
value associated with the second parameter's first entry, second will be the value associated
with the second parameter's second entry, and so on.) You SHOULD assume that every
dictionary will contain all of the keys from the first parameter.
Python creates a new list only when a variable is assigned a list literal. You MUST
include the statement initializing the variable to which the dictionary's values are added
INSIDE the for-loop iterating through the first parameter.
As an example, if this were a function call:
list_gen ([{'Used Key': 'Data', 'Unused Keys' : 'More Data' }],
['Used Key'])
the expected return value would be:
[ ['Data']]
As an second example, if this were a function call:
list_gen ([{'Hint': 'Length 2 Not Required', 'Num' : 8675309),
{'Num': 1, 'Hint': 'Use 1st param order'}],
['Hint', 'Num'])
the expected return value would be:
[ ['Length 2 Not Required', 8675309],
['Use 1st param order', 1] ]
Page 3 / 4
+](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F89b7b4a3-edc3-4856-b3b8-970ee403a9a8%2F3265cf23-c6fb-4c6a-b9be-c0446bd50d89%2Fcc5nwhg_processed.png&w=3840&q=75)
Transcribed Image Text:list_gen
Define a function named is open with Google Paraineters. The first parameter will be a list of
dictionaries. The second parameter will be a list of strings (dictionary keys)..
The function needs to return a list of lists. The length of the returned list must be identical to the
length of the first parameter. The order of these dictionaries will be paralleled in the entries in
the returned list. (So the first entry will be the list created for the first dictionary; the second entry
will be the list created for the second dictionary, and so on.)
Each entry in the returned list will contain a list of values from that dictionary. The second
parameter's entries specify the keys whose values should be added to this list. The order of
these values must parallel the order of the keys in the second parameter. (So first will be the
value associated with the second parameter's first entry, second will be the value associated
with the second parameter's second entry, and so on.) You SHOULD assume that every
dictionary will contain all of the keys from the first parameter.
Python creates a new list only when a variable is assigned a list literal. You MUST
include the statement initializing the variable to which the dictionary's values are added
INSIDE the for-loop iterating through the first parameter.
As an example, if this were a function call:
list_gen ([{'Used Key': 'Data', 'Unused Keys' : 'More Data' }],
['Used Key'])
the expected return value would be:
[ ['Data']]
As an second example, if this were a function call:
list_gen ([{'Hint': 'Length 2 Not Required', 'Num' : 8675309),
{'Num': 1, 'Hint': 'Use 1st param order'}],
['Hint', 'Num'])
the expected return value would be:
[ ['Length 2 Not Required', 8675309],
['Use 1st param order', 1] ]
Page 3 / 4
+
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
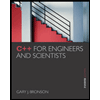
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
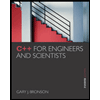
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr