LLNode: public class LLNode { protected T info; protectedLLNodelink; public LLNode(Tinfo){ this.info=info; link=null; } publicvoidsetInfo(Tinfo){ this.info=info; } publicTgetInfo(){ return info; } publicvoidsetLink(LLNodelink){ this.link=link; } publicLLNodegetLink(){ return link; } @Override public String toString(){ return "LLNode [info="+info+", link="+link+"]"; } } MyLLNode: public class MyLLNode { LLNodehead; public MyLLNode(){ this.head=null; } public MyLLNode(String first){ this.head=newLLNode(first); } //------------------------------------------------------------- public void addAtEnd(String newstr){ LLNodenode=head; LLNodenewNode=newLLNode(newstr); if(this.head==null){ this.head=newNode; } else{ while(node.getLink()!=null){ node=node.getLink(); } node.setLink(newNode); } } //------------------------------------------------------------- public void addAtFront(String newstr){ LLNodenewNode=newLLNode(newstr); if(this.head==null){ this.head=newNode; } else{ newNode.setLink(this.head); this.head=newNode; } } //------------------------------------------------------------- public boolean contains(String item){ LLNodetemp=head; while(temp!=null){ if(temp.getInfo().equalsIgnoreCase(item)){ return true; } temp=temp.getLink(); } return false; } //------------------------------------------------------------- public String print(){ String result=""; LLNodetemp=head; while(temp!=null){ result+=temp.getInfo()+"-->"; temp=temp.getLink(); } return result; }
LLNode:
public class LLNode<T> {
protected T info;
protectedLLNode<T>link;
public LLNode(Tinfo){
this.info=info;
link=null;
}
publicvoidsetInfo(Tinfo){
this.info=info;
}
publicTgetInfo(){
return info;
}
publicvoidsetLink(LLNode<T>link){
this.link=link;
}
publicLLNode<T>getLink(){
return link;
}
@Override
public String toString(){
return "LLNode [info="+info+", link="+link+"]";
}
}
MyLLNode:
public class MyLLNode {
LLNode<String>head;
public MyLLNode(){
this.head=null;
}
public MyLLNode(String first){
this.head=newLLNode<String>(first);
}
//-------------------------------------------------------------
public void addAtEnd(String newstr){
LLNode<String>node=head;
LLNode<String>newNode=newLLNode<String>(newstr);
if(this.head==null){
this.head=newNode;
}
else{
while(node.getLink()!=null){
node=node.getLink();
}
node.setLink(newNode);
}
}
//-------------------------------------------------------------
public void addAtFront(String newstr){
LLNode<String>newNode=newLLNode<String>(newstr);
if(this.head==null){
this.head=newNode;
}
else{
newNode.setLink(this.head);
this.head=newNode;
}
}
//-------------------------------------------------------------
public boolean contains(String item){
LLNode<String>temp=head;
while(temp!=null){
if(temp.getInfo().equalsIgnoreCase(item)){
return true;
}
temp=temp.getLink();
}
return false;
}
//-------------------------------------------------------------
public String print(){
String result="";
LLNode<String>temp=head;
while(temp!=null){
result+=temp.getInfo()+"-->";
temp=temp.getLink();
}
return result;
}
}


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

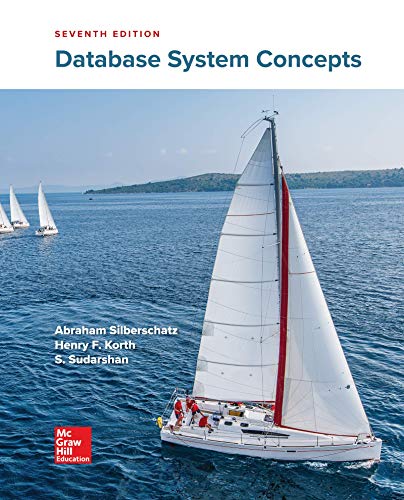
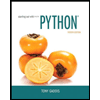
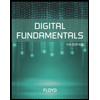
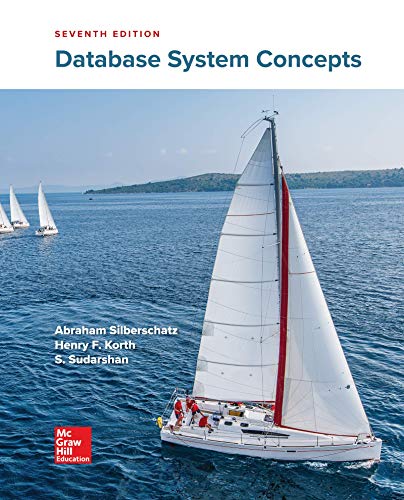
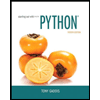
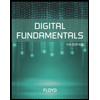
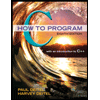
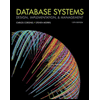
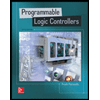