Math 130 Java Programming Does my code pass the requirements? May I have more explanation? Does my code compile correctly? Is my code readable? Is my code well commented? How may I better organize my code? Are there whitespaces to appropriate help separate distinct parts? My code: import java.util.Scanner; public class TicketSale { public static void main(String[] args) { Scanner keyboard = new Scanner(System.in); //Prompt user to input the number of Adult tickets to purchase System.out.print("Please enter number of adults:"); //Prompt user to input the number of Adult tickets to purchase int adults = keyboard.nextInt(); //Prompt user to input the number of Children tickets to purchase System.out.print("Please enter number of children:"); //Prompt user to input the number of Children tickets to purchase int children = keyboard.nextInt(); //Calculate and display the total cost for adults and children System.out.print("Your total cost for " + adults + getadulttxt(adults) + " and " + children + getChildtxt(children) + " is: $"); double cost = ticketCost(adults, children); //invoke method System.out.printf("%.2f", cost); keyboard.close(); } //Returns the appropriate text for adult(s) based on the count public static String getadulttxt(int adults) { if(adults > 1) { return " adults"; } else return " adult"; } //Returns the appropriate text for child/children based on the count public static String getChildtxt(int children) { if(children > 1) return " children"; else return " child"; } //Calculate the total cost of tickets based on the number of adults and children public static double ticketCost(int adults, int children) { //Calculate ticket prices double priceperadults = 16.00; double priceperchild = 8.50; double pricePeraccompaniedChild = 5.50; //Calculate the number of accompanied children int accompaniedChildren = adults * 2; //Adjust the count of children and accompanied children based on availability if(children>=accompaniedChildren) { children-=accompaniedChildren ; } else { accompaniedChildren = children; children = 0; } //Calculate the total cost double total = (priceperchild*children) + (priceperadults *adults) + (pricePeraccompaniedChild * accompaniedChildren); //Apply discounts based on the number of adults if(adults >= 20) { total *= .90; // Ten percent discount } else if(adults>= 10) { total*=.94; // Six percent discount } return total; } } Thank you
Math 130 Java Programming Does my code pass the requirements? May I have more explanation? Does my code compile correctly? Is my code readable? Is my code well commented? How may I better organize my code? Are there whitespaces to appropriate help separate distinct parts? My code: import java.util.Scanner; public class TicketSale { public static void main(String[] args) { Scanner keyboard = new Scanner(System.in); //Prompt user to input the number of Adult tickets to purchase System.out.print("Please enter number of adults:"); //Prompt user to input the number of Adult tickets to purchase int adults = keyboard.nextInt(); //Prompt user to input the number of Children tickets to purchase System.out.print("Please enter number of children:"); //Prompt user to input the number of Children tickets to purchase int children = keyboard.nextInt(); //Calculate and display the total cost for adults and children System.out.print("Your total cost for " + adults + getadulttxt(adults) + " and " + children + getChildtxt(children) + " is: $"); double cost = ticketCost(adults, children); //invoke method System.out.printf("%.2f", cost); keyboard.close(); } //Returns the appropriate text for adult(s) based on the count public static String getadulttxt(int adults) { if(adults > 1) { return " adults"; } else return " adult"; } //Returns the appropriate text for child/children based on the count public static String getChildtxt(int children) { if(children > 1) return " children"; else return " child"; } //Calculate the total cost of tickets based on the number of adults and children public static double ticketCost(int adults, int children) { //Calculate ticket prices double priceperadults = 16.00; double priceperchild = 8.50; double pricePeraccompaniedChild = 5.50; //Calculate the number of accompanied children int accompaniedChildren = adults * 2; //Adjust the count of children and accompanied children based on availability if(children>=accompaniedChildren) { children-=accompaniedChildren ; } else { accompaniedChildren = children; children = 0; } //Calculate the total cost double total = (priceperchild*children) + (priceperadults *adults) + (pricePeraccompaniedChild * accompaniedChildren); //Apply discounts based on the number of adults if(adults >= 20) { total *= .90; // Ten percent discount } else if(adults>= 10) { total*=.94; // Six percent discount } return total; } } Thank you
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Math 130 Java Programming
Does my code pass the requirements? May I have more explanation? Does my code compile correctly? Is my code readable? Is my code well commented? How may I better organize my code? Are there whitespaces to appropriate help separate distinct parts?
My code:
import java.util.Scanner;
public class TicketSale
{
public static void main(String[] args)
{
Scanner keyboard = new Scanner(System.in);
//Prompt user to input the number of Adult tickets to purchase
System.out.print("Please enter number of adults:"); //Prompt user to input the number of Adult tickets to purchase
int adults = keyboard.nextInt();
//Prompt user to input the number of Children tickets to purchase
System.out.print("Please enter number of children:"); //Prompt user to input the number of Children tickets to purchase
int children = keyboard.nextInt();
//Calculate and display the total cost for adults and children
System.out.print("Your total cost for " + adults + getadulttxt(adults) + " and " + children + getChildtxt(children) + " is: $");
double cost = ticketCost(adults, children); //invoke method
System.out.printf("%.2f", cost);
keyboard.close();
}
//Returns the appropriate text for adult(s) based on the count
public static String getadulttxt(int adults)
{
if(adults > 1)
{
return " adults";
}
else
return " adult";
}
//Returns the appropriate text for child/children based on the count
public static String getChildtxt(int children)
{
if(children > 1)
return " children";
else
return " child";
}
//Calculate the total cost of tickets based on the number of adults and children
public static double ticketCost(int adults, int children)
{
//Calculate ticket prices
double priceperadults = 16.00;
double priceperchild = 8.50;
double pricePeraccompaniedChild = 5.50;
//Calculate the number of accompanied children
int accompaniedChildren = adults * 2;
//Adjust the count of children and accompanied children based on availability
if(children>=accompaniedChildren)
{
children-=accompaniedChildren ;
}
else
{
accompaniedChildren = children;
children = 0;
}
//Calculate the total cost
double total = (priceperchild*children) + (priceperadults *adults) + (pricePeraccompaniedChild * accompaniedChildren);
//Apply discounts based on the number of adults
if(adults >= 20)
{
total *= .90; // Ten percent discount
}
else if(adults>= 10)
{
total*=.94; // Six percent discount
}
return total;
}
}
Thank you
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
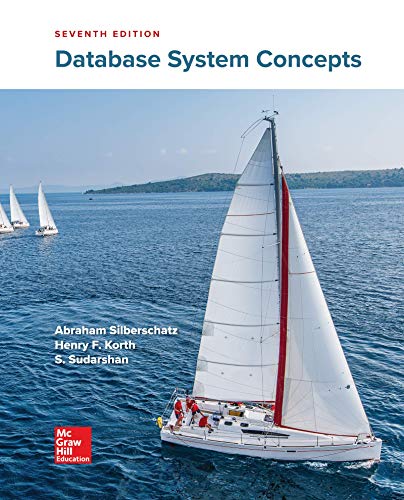
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
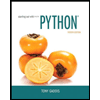
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
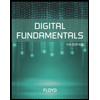
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
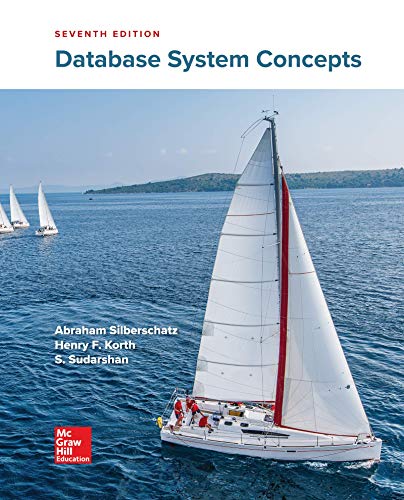
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
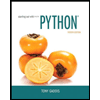
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
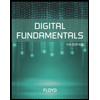
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
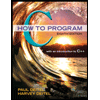
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
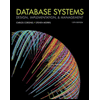
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
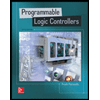
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education