Modify this class by: 1. Suppose the bank wants to keep track of how many accounts exist. A. Do you need a static variable or a regular variable to save how many accounts have been created? Declare it and name the variable as numAccounts. It should be initialized to 0 (since no account exists initially). B. Update this variable in the constructor. (Ask the instructor if you do not know how) C. Add a method getNumAccounts that returns the total number of accounts. Decide if this method should be static – is the information related to a single account or is it for the entire class? Does this method manipulate a static variable? Complete this method. 2. Use the driver class TestAccounts1.java to test your modified Account class. Complete the code as instructed. 3. Add a method void close() to your Account class. This method should close the current account by appending “CLOSED” to the account name and setting the balance to 0. Also, decrement the total number of accounts. Should this method be static or non-static? 4. Modify the driver program to test this method. Choose one of the existing accounts to close. Then print this account as well as the total number of accounts existing, which should be one less after closing the account.
Modify this class by:
1. Suppose the bank wants to keep track of how many accounts exist.
A. Do you need a static variable or a regular variable to save how many accounts have been created? Declare it and name the variable as numAccounts. It should be initialized to 0 (since no account exists initially).
B. Update this variable in the constructor. (Ask the instructor if you do not know how)
C. Add a method getNumAccounts that returns the total number of accounts. Decide if this method should be static – is the information related to a single account or is it for the entire class? Does this method manipulate a static variable? Complete this method.
2. Use the driver class TestAccounts1.java to test your modified Account class. Complete the code as instructed.
3. Add a method void close() to your Account class. This method should close the current account by appending “CLOSED” to the account name and setting the balance to 0. Also, decrement the total number of accounts. Should this method be static or non-static?
4. Modify the driver program to test this method. Choose one of the existing accounts to close. Then print this account as well as the total number of accounts existing, which should be one less after closing the account.
Code:
//*******************************************************
// Account.java
//
// A bank account class with methods to deposit, withdraw,
// and check the balance.
//*******************************************************
public class Account
{
private double balance;
private String name;
private long acctNum;
//----------------------------------------------
//Constructor -- initializes balance, owner, and account number
//----------------------------------------------
public Account(double initBal, String owner, long number)
{
balance = initBal;
name = owner;
acctNum = number;
}
//----------------------------------------------
// Checks to see if balance is sufficient for withdrawal.
// If so decrements balance by amount; if not, prints message.
//----------------------------------------------
public void withdraw(double amount)
{
if (balance >= amount)
balance -= amount;
else
System.out.println("Insufficient funds");
}
//----------------------------------------------
// Adds deposit amount to balance.
//----------------------------------------------
public void deposit(double amount)
{
balance += amount;
}
//----------------------------------------------
// Returns balance.
//----------------------------------------------
public double getBalance()
{
return balance;
}
//----------------------------------------------
// Returns account number
//----------------------------------------------
public double getAcctNumber()
{
return acctNum;
}
//----------------------------------------------
// Returns a string containing the name, acct number, and balance.
//----------------------------------------------
public String toString()
{
return "Name: " + name +
"\nAcct #: " + acctNum +
"\nBalance: " + balance;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

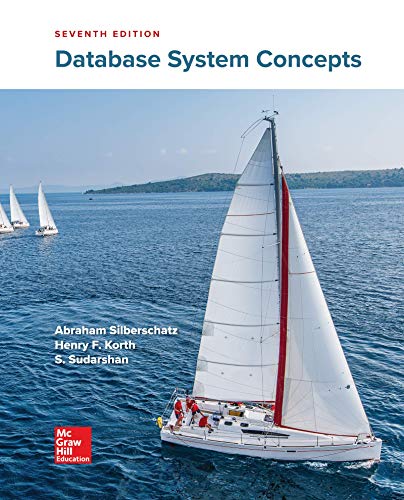
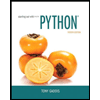
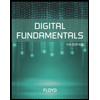
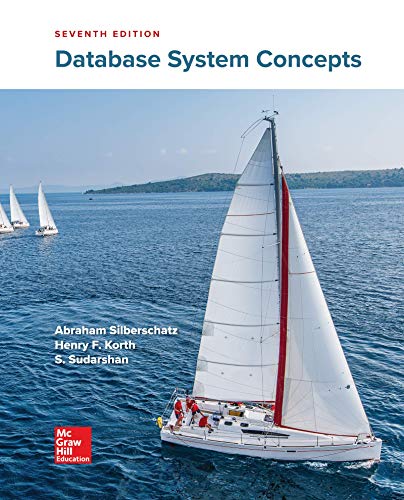
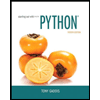
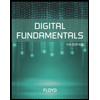
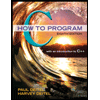
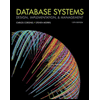
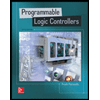