mplement a class CreditCard. A CreditCard is defined by a 16-digit number and an expiration date. A valid credit card number is defined as follows: 1. Form the sum of all digits. 2. Add to the sum from step 1 every other digit, starting with the second digit. 3. Count the number of digits in step 2 that are greater than 5, and add this count to the sum from step 2. If the re is divisible by 3, the credit card is valid. or example, consider the number 4022 8888 8888 1881.
mplement a class CreditCard. A CreditCard is defined by a 16-digit number and an expiration date. A valid credit card number is defined as follows: 1. Form the sum of all digits. 2. Add to the sum from step 1 every other digit, starting with the second digit. 3. Count the number of digits in step 2 that are greater than 5, and add this count to the sum from step 2. If the re is divisible by 3, the credit card is valid. or example, consider the number 4022 8888 8888 1881.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter5: Control Structures Ii (repetition)
Section: Chapter Questions
Problem 20PE: When you borrow money to buy a house, a car, or for some other purpose, you repay the loan by making...
Related questions
Question
Java. Please use the template

Transcribed Image Text:Problem 5: CreditCard
Implement a class CreditCard. A CreditCard is defined by a 16-digit number and an expiration date.
A valid credit card number is defined as follows:
1. Form the sum of all digits.
2. Add to the sum from step 1 every other digit, starting with the second digit.
3. Count the number of digits in step 2 that are greater than 5, and add this count to the sum from step 2. If the result
is divisible by 3, the credit card is valid.
For example, consider the number 4022 8888 8888 1881.
1. The sum of all digits is 90.
2. The sum of the bolded digits is 43. Running sum is now 90 + 43 = 133.
3. There are 5 bolded digits larger than 5, so running sum is now 133 + 5 = 138. 138 is divisible by 3 so the card
number is valid.
A valid expiration date is one that has not yet occurred at the time the program is run (hint: see the Java library class
LocalDate).
A valid credit card is one that has both a valid number and a valid expiration date.
Use this template: CreditCard.java
Examples:
CreditCard ccl
new CreditCard(4022888888881881L, LocalDate.of(2024, 2, 28));
cc1.getNumber(); // returns 4022888888881881L
ccl.getExpirationDate(); // returns 2024-02-28
ccl.getNumberWithSpaces (); // returns "4022 8888 8888 1881"
ccl.isValid(); // returns true
CreditCard cc2
new CreditCard(4022888888881881L, LocalDate.of(2021, 2, 28));
cc2.getNumber(); // returns 4022888888881881L
cc2.getExpirationDate () ; // returns 2021-02-28
cc2.getNumberWithSpaces (); // returns "4022 8888 8888 1881"
cc2.isValid(); // returns false

Transcribed Image Text:3 import java.time.LocalDate;
4
50 /**
Represents a credit card
8.
* /
9 public class CreditCard {
10
11
// ADD YOUR INSTANCE VARIABLES HERE
12
13
140
15
16
17
18
19
20
/**
* Constructs a credit card object with the given number and expiration
* date
Assume the number passed in will always have 16 digits and will not
* start with 0.
*
*
* Assume the date passed in will always be a valid date.
21
22
23
24
25
*
@param number
@param expDate
* /
public CreditCard(long number, LocalDate expDate) {
// FILL IN
}
the credit card number
the credit card expiration date
*
260
27
28
29
30
310
/**
32
33
34
35
360
37
38
39
Returns the credit card number
*
* @return
the credit card number
*/
public long getNumber() {
return -1; // FIX ME
}
40
410
42
43
44
/**
Returns the credit card expiration date
*
@return the credit card expiration date
45.
460
47
* /
public LocalDate getExpirationDate() {
return LocalDate.now(); // FIX ME
48
49
50
510
52
/**
Returns a string containing the card number, with a space inserted
* between every 4 digits
53
54
55
@return
the card number, with a space inserted between every 4 digits
56
570
58
*/
public String getNumberWithSpaces() {
return ""; /| FIX ME
}
59
60
61
620
**
Returns true if both the card number and expiration date are valid,
* false otherwise
63
64
65
66
@return whether card is valid
*/
67
680
public boolean isValid() {
return false; // FIX ME
}
69
70
71 }
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 6 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
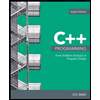
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
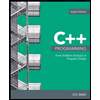
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning