Need help describing these: #define NAMELEN 2048 #define BUFSIZE 1024 #include <sys/types.h> #include <sys/stat.h> #include <time.h> #include <stdio.h> #include <stdlib.h> char *strmode(mode_t mode); // nasty function below this one int main(int argc, char *argv[]) { if (argc != 2) { fprintf(stderr, "Usage: %s <pathname>\n", argv[0]); exit(EXIT_FAILURE); } if(access(argv[1], F_OK)!=0){ // check file exists perror("Couldn't access file"); exit(EXIT_FAILURE); } struct stat sb; if (stat(argv[1], &sb) == -1) { // call to stat(), fills sb, distinguishes symbolic links perror("stat"); exit(EXIT_FAILURE); } printf("Filename: %s\n",argv[1]); printf("File type: "); if(0){} // checks to determine file type else if S_ISBLK (sb.st_mode) { printf("block device\n"); } else if S_ISCHR (sb.st_mode) { printf("character device\n"); } else if S_ISDIR (sb.st_mode) { printf("directory\n"); } else if S_ISFIFO(sb.st_mode) { printf("FIFO/pipe\n"); } else if S_ISLNK (sb.st_mode) { printf("symlink\n"); } else if S_ISREG (sb.st_mode) { printf("regular file\n"); } else if S_ISSOCK(sb.st_mode) { printf("socket\n"); } else{ printf("unknown?\n"); } // Use various fields to display information printf("I-node number: %ld\n" , (long) sb.st_ino); printf("Permissions: %lo (octal)\n" , (unsigned long) sb.st_mode); printf("Link count: %ld\n" , (long) sb.st_nlink); printf("Ownership: UID=%ld GID=%ld\n" , (long) sb.st_uid, (long) sb.st_gid); printf("Preferred I/O block size: %ld bytes\n" , (long) sb.st_blksize); printf("File size: %lld bytes\n" , (long long) sb.st_size); printf("Blocks allocated: %lld\n" , (long long) sb.st_blocks); // Print times of last access printf("Last status change: %s", ctime(&sb.st_ctime)); // formats time as a string printf("Last file access: %s", ctime(&sb.st_atime)); // fields like st_atime are of printf("Last file modification: %s", ctime(&sb.st_mtime)); // type time_t taken by ctime() char *str_mode = strmode(sb.st_mode); // use strmode() form strmode.c to construct permissions string printf("Permissions: %s\n", str_mode); // Optionally report device number // printf("ID of containing device: [%lx,%lx]\n", (long) major(sb.st_dev), (long) minor(sb.st_dev)); exit(EXIT_SUCCESS); } A) What's the best way to describe how the 'access()' system call is used? B) How does `stat()' report the Size of a file? C) `stat()()' report the "kind" of file being queried. How is this information used in `stat_demo.c'? D) Which one of the following is NOT a file "kind" reported by `stat() / lstat()' - ( ) Directory - ( ) Binary - ( ) Socket - ( ) Symbolic Link (symlink) E) What's the difference between ctime and mtime reported by stat()?
Need help describing these: #define NAMELEN 2048 #define BUFSIZE 1024 #include <sys/types.h> #include <sys/stat.h> #include <time.h> #include <stdio.h> #include <stdlib.h> char *strmode(mode_t mode); // nasty function below this one int main(int argc, char *argv[]) { if (argc != 2) { fprintf(stderr, "Usage: %s <pathname>\n", argv[0]); exit(EXIT_FAILURE); } if(access(argv[1], F_OK)!=0){ // check file exists perror("Couldn't access file"); exit(EXIT_FAILURE); } struct stat sb; if (stat(argv[1], &sb) == -1) { // call to stat(), fills sb, distinguishes symbolic links perror("stat"); exit(EXIT_FAILURE); } printf("Filename: %s\n",argv[1]); printf("File type: "); if(0){} // checks to determine file type else if S_ISBLK (sb.st_mode) { printf("block device\n"); } else if S_ISCHR (sb.st_mode) { printf("character device\n"); } else if S_ISDIR (sb.st_mode) { printf("directory\n"); } else if S_ISFIFO(sb.st_mode) { printf("FIFO/pipe\n"); } else if S_ISLNK (sb.st_mode) { printf("symlink\n"); } else if S_ISREG (sb.st_mode) { printf("regular file\n"); } else if S_ISSOCK(sb.st_mode) { printf("socket\n"); } else{ printf("unknown?\n"); } // Use various fields to display information printf("I-node number: %ld\n" , (long) sb.st_ino); printf("Permissions: %lo (octal)\n" , (unsigned long) sb.st_mode); printf("Link count: %ld\n" , (long) sb.st_nlink); printf("Ownership: UID=%ld GID=%ld\n" , (long) sb.st_uid, (long) sb.st_gid); printf("Preferred I/O block size: %ld bytes\n" , (long) sb.st_blksize); printf("File size: %lld bytes\n" , (long long) sb.st_size); printf("Blocks allocated: %lld\n" , (long long) sb.st_blocks); // Print times of last access printf("Last status change: %s", ctime(&sb.st_ctime)); // formats time as a string printf("Last file access: %s", ctime(&sb.st_atime)); // fields like st_atime are of printf("Last file modification: %s", ctime(&sb.st_mtime)); // type time_t taken by ctime() char *str_mode = strmode(sb.st_mode); // use strmode() form strmode.c to construct permissions string printf("Permissions: %s\n", str_mode); // Optionally report device number // printf("ID of containing device: [%lx,%lx]\n", (long) major(sb.st_dev), (long) minor(sb.st_dev)); exit(EXIT_SUCCESS); } A) What's the best way to describe how the 'access()' system call is used? B) How does `stat()' report the Size of a file? C) `stat()()' report the "kind" of file being queried. How is this information used in `stat_demo.c'? D) Which one of the following is NOT a file "kind" reported by `stat() / lstat()' - ( ) Directory - ( ) Binary - ( ) Socket - ( ) Symbolic Link (symlink) E) What's the difference between ctime and mtime reported by stat()?
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Topic Video
Question
Need help describing these:
#define NAMELEN 2048
#define BUFSIZE 1024
#include <sys/types.h>
#include <sys/stat.h>
#include <time.h>
#include <stdio.h>
#include <stdlib.h>
char *strmode(mode_t mode); // nasty function below this one
int main(int argc, char *argv[]) {
if (argc != 2) {
fprintf(stderr, "Usage: %s <pathname>\n", argv[0]);
exit(EXIT_FAILURE);
}
if(access(argv[1], F_OK)!=0){ // check file exists
perror("Couldn't access file");
exit(EXIT_FAILURE);
}
struct stat sb;
if (stat(argv[1], &sb) == -1) { // call to stat(), fills sb, distinguishes symbolic links
perror("stat");
exit(EXIT_FAILURE);
}
printf("Filename: %s\n",argv[1]);
printf("File type: ");
if(0){} // checks to determine file type
else if S_ISBLK (sb.st_mode) { printf("block device\n"); }
else if S_ISCHR (sb.st_mode) { printf("character device\n"); }
else if S_ISDIR (sb.st_mode) { printf("directory\n"); }
else if S_ISFIFO(sb.st_mode) { printf("FIFO/pipe\n"); }
else if S_ISLNK (sb.st_mode) { printf("symlink\n"); }
else if S_ISREG (sb.st_mode) { printf("regular file\n"); }
else if S_ISSOCK(sb.st_mode) { printf("socket\n"); }
else{ printf("unknown?\n"); }
// Use various fields to display information
printf("I-node number: %ld\n" , (long) sb.st_ino);
printf("Permissions: %lo (octal)\n" , (unsigned long) sb.st_mode);
printf("Link count: %ld\n" , (long) sb.st_nlink);
printf("Ownership: UID=%ld GID=%ld\n" , (long) sb.st_uid, (long) sb.st_gid);
printf("Preferred I/O block size: %ld bytes\n" , (long) sb.st_blksize);
printf("File size: %lld bytes\n" , (long long) sb.st_size);
printf("Blocks allocated: %lld\n" , (long long) sb.st_blocks);
// Print times of last access
printf("Last status change: %s", ctime(&sb.st_ctime)); // formats time as a string
printf("Last file access: %s", ctime(&sb.st_atime)); // fields like st_atime are of
printf("Last file modification: %s", ctime(&sb.st_mtime)); // type time_t taken by ctime()
char *str_mode = strmode(sb.st_mode); // use strmode() form strmode.c to construct permissions string
printf("Permissions: %s\n", str_mode);
// Optionally report device number
// printf("ID of containing device: [%lx,%lx]\n", (long) major(sb.st_dev), (long) minor(sb.st_dev));
exit(EXIT_SUCCESS);
}
A) What's the best way to describe how the 'access()' system call is
used?
B) How does `stat()' report the Size of a file?
C) `stat()()' report the "kind" of file being queried. How is this
information used in `stat_demo.c'?
D) Which one of the following is NOT a file "kind" reported by `stat() /
lstat()'
- ( ) Directory
- ( ) Binary
- ( ) Socket
- ( ) Symbolic Link (symlink)
E) What's the difference between ctime and mtime reported by stat()?
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
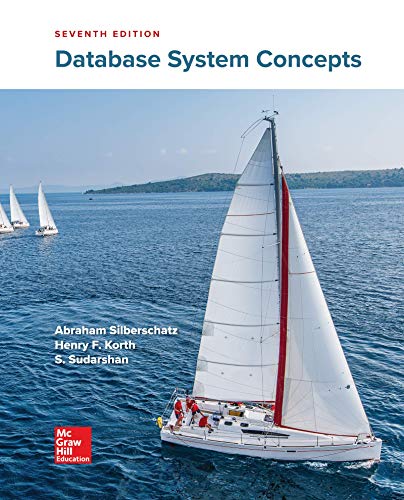
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
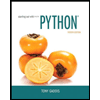
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
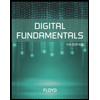
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
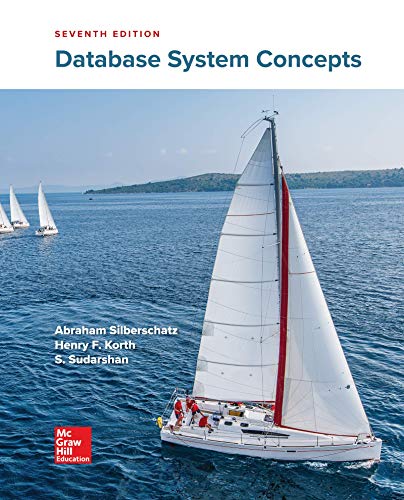
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
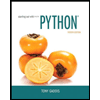
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
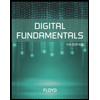
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
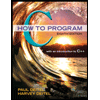
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
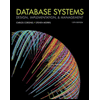
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
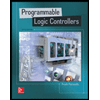
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education