Need help with this beginning Java question " PROGRAMMING PROJECT #2A: Managing Your Courses Using Exceptions & File I/Os Purpose: • Define class using OO approach • Understand how to create and manipulate list of objects • Design and create a well-structure program using basic programming constructs and classes • Utilizing files for input data and output • Throw/Handle Exceptions when necessary. Description: Write a program to help you to manage courses you are taking this semester. The program must read a list of courses from a file “courses.txt” and initialize the list of courses using data from this file. If the file does not exist, the program must throw an exception. The course data file contains one line per course which contains: id, number of units, and title. Here is a sample of data in “courses.txt”: CIS001 2 Computer Literacy CIS002 4 Introduction to Computing CIS045 3 Linux Essential I CIS010 5 Computer Programming Lab Your program provides the following options: 1. List all courses 2. Add a course 3. Search word: show all the courses that has the word in the course title (ask the user for the word) 4. List >= input-unit: show all the courses that has unit >= input-unit (ask the user for input) 5. Exit Explanation of options: 1. The program prints your course list. Initially, it should say “Your list is empty” 2. The program asks the user input for a course including: course ID, number of units and title. 3. The program asks the user to input the search word. Then, display all courses that has that word in the title. The search must be case- insensitive. For example, “computer” and “Computer” are considered a match. 4. List courses that have the number of unit >= the user input 5. Write course data to “courses.txt” before terminating the program. Requirements: A. Here is the minimum list of classes that you must have: 1. “Course.java” class - Manages a course (id, number of units, and title) - Keep track of course count in a static variable. You will need this count in other places. 2. “ManageCourses.java” program: Implement the main program that perform the following tasks: a. Reads in the data file “courses.txt” and initialize the arrayList of Course objects. For each course, create one Course object and store it into the arrayList. b. It processes all the commands to manage the courses in your list. c. It should have a method to search for unit, for example: public static void searchCourseUnit( Course[] courseArray, int unitValue) Or public static void searchCourseUnit( Course[] courseArray, int size, int unitValue) This method must throw an Exception (you decide what Exception class to throw) if the unitValue is <= 0. a. It should have another method to search for word in title, for example: public static void searchCourseTitle( Course[] courseArray, String target) Or public static void searchCourseTitle( Course[] courseArray, int size, String target) This method must throw an Exception (you decide what Exception class to throw) if the target is empty. 3. If the input file “courses.txt” does not exist, handle the exception and exit gracefully. Your program should print and error message. It should not crash. 4. You need to submit all java files and “courses.txt” file. 5. You must have comments in your class and methods to describe what the class provide and what the methods do."
Need help with this beginning Java question " PROGRAMMING PROJECT #2A: Managing Your Courses Using Exceptions & File I/Os Purpose: • Define class using OO approach • Understand how to create and manipulate list of objects • Design and create a well-structure program using basic programming constructs and classes • Utilizing files for input data and output • Throw/Handle Exceptions when necessary. Description: Write a program to help you to manage courses you are taking this semester. The program must read a list of courses from a file “courses.txt” and initialize the list of courses using data from this file. If the file does not exist, the program must throw an exception. The course data file contains one line per course which contains: id, number of units, and title. Here is a sample of data in “courses.txt”: CIS001 2 Computer Literacy CIS002 4 Introduction to Computing CIS045 3 Linux Essential I CIS010 5 Computer Programming Lab Your program provides the following options: 1. List all courses 2. Add a course 3. Search word: show all the courses that has the word in the course title (ask the user for the word) 4. List >= input-unit: show all the courses that has unit >= input-unit (ask the user for input) 5. Exit Explanation of options: 1. The program prints your course list. Initially, it should say “Your list is empty” 2. The program asks the user input for a course including: course ID, number of units and title. 3. The program asks the user to input the search word. Then, display all courses that has that word in the title. The search must be case- insensitive. For example, “computer” and “Computer” are considered a match. 4. List courses that have the number of unit >= the user input 5. Write course data to “courses.txt” before terminating the program. Requirements: A. Here is the minimum list of classes that you must have: 1. “Course.java” class - Manages a course (id, number of units, and title) - Keep track of course count in a static variable. You will need this count in other places. 2. “ManageCourses.java” program: Implement the main program that perform the following tasks: a. Reads in the data file “courses.txt” and initialize the arrayList of Course objects. For each course, create one Course object and store it into the arrayList. b. It processes all the commands to manage the courses in your list. c. It should have a method to search for unit, for example: public static void searchCourseUnit( Course[] courseArray, int unitValue) Or public static void searchCourseUnit( Course[] courseArray, int size, int unitValue) This method must throw an Exception (you decide what Exception class to throw) if the unitValue is <= 0. a. It should have another method to search for word in title, for example: public static void searchCourseTitle( Course[] courseArray, String target) Or public static void searchCourseTitle( Course[] courseArray, int size, String target) This method must throw an Exception (you decide what Exception class to throw) if the target is empty. 3. If the input file “courses.txt” does not exist, handle the exception and exit gracefully. Your program should print and error message. It should not crash. 4. You need to submit all java files and “courses.txt” file. 5. You must have comments in your class and methods to describe what the class provide and what the methods do."
Chapter4: More Object Concepts
Section: Chapter Questions
Problem 5RQ
Related questions
Question
Need help with this beginning Java question "
& File I/Os
Purpose:
• Define class using OO approach
• Understand how to create and manipulate list of objects
• Design and create a well-structure program using basic programming
constructs and classes
• Utilizing files for input data and output
• Throw/Handle Exceptions when necessary.
Description:
Write a program to help you to manage courses you are taking this semester.
The program must read a list of courses from a file “courses.txt” and initialize
the list of courses using data from this file. If the file does not exist, the program
must throw an exception. The course data file contains one line per course
which contains: id, number of units, and title.
Here is a sample of data in “courses.txt”:
CIS001 2 Computer Literacy
CIS002 4 Introduction to Computing
CIS045 3 Linux Essential I
CIS010 5 Computer Programming Lab
Your program provides the following options:
1. List all courses
2. Add a course
3. Search word: show all the courses that has the word in the course
title (ask the user for the word)
4. List >= input-unit: show all the courses that has unit >= input-unit
(ask the user for input)
5. Exit
Explanation of options:
1. The program prints your course list. Initially, it should say “Your list is
empty”
2. The program asks the user input for a course including: course ID, number
of units and title.
3. The program asks the user to input the search word. Then, display all
courses that has that word in the title. The search must be case-
insensitive. For example, “computer” and “Computer” are considered a
match.
4. List courses that have the number of unit >= the user input
5. Write course data to “courses.txt” before terminating the program.
Requirements:
A. Here is the minimum list of classes that you must have:
1. “Course.java” class
- Manages a course (id, number of units, and title)
- Keep track of course count in a static variable. You will need this count
in other places.
2. “ManageCourses.java” program: Implement the main program that
perform the following tasks:
a. Reads in the data file “courses.txt” and initialize the arrayList of
Course objects. For each course, create one Course object and
store it into the arrayList.
b. It processes all the commands to manage the courses in your list.
c. It should have a method to search for unit, for example:
public static void searchCourseUnit(
Course[] courseArray, int unitValue)
Or
public static void searchCourseUnit(
Course[] courseArray, int size, int unitValue)
This method must throw an Exception (you decide what Exception
class to throw) if the unitValue is <= 0.
a. It should have another method to search for word in title, for
example:
public static void searchCourseTitle(
Course[] courseArray, String target)
Or
public static void searchCourseTitle(
Course[] courseArray, int size, String target)
This method must throw an Exception (you decide what Exception
class to throw) if the target is empty.
3. If the input file “courses.txt” does not exist, handle the exception and exit
gracefully. Your program should print and error message. It should not
crash.
4. You need to submit all java files and “courses.txt” file.
5. You must have comments in your class and methods to describe what the
class provide and what the methods do."
5. Write course data to “courses.txt” before terminating the program.
Requirements:
A. Here is the minimum list of classes that you must have:
1. “Course.java” class
- Manages a course (id, number of units, and title)
- Keep track of course count in a static variable. You will need this count
in other places.
2. “ManageCourses.java” program: Implement the main program that
perform the following tasks:
a. Reads in the data file “courses.txt” and initialize the arrayList of
Course objects. For each course, create one Course object and
store it into the arrayList.
b. It processes all the commands to manage the courses in your list.
c. It should have a method to search for unit, for example:
public static void searchCourseUnit(
Course[] courseArray, int unitValue)
Or
public static void searchCourseUnit(
Course[] courseArray, int size, int unitValue)
This method must throw an Exception (you decide what Exception
class to throw) if the unitValue is <= 0.
a. It should have another method to search for word in title, for
example:
public static void searchCourseTitle(
Course[] courseArray, String target)
Or
public static void searchCourseTitle(
Course[] courseArray, int size, String target)
This method must throw an Exception (you decide what Exception
class to throw) if the target is empty.
3. If the input file “courses.txt” does not exist, handle the exception and exit
gracefully. Your program should print and error message. It should not
crash.
4. You need to submit all java files and “courses.txt” file.
5. You must have comments in your class and methods to describe what the
class provide and what the methods do."
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
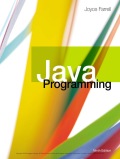
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
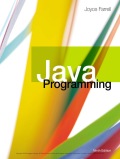
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT