ng Language Given the code below 1.Change all of the low-level arrays in your program to use the appropriate C# collection class instead. Be sure to implement Deck as a Stack 2. Make sure that you use the correct type-safe generic designations throughout your program. 3.Write code that watches for that incorrect data and throws an appropriate exception if bad data is found. 4. Write Properties instead of Getter and Setter methods. no lamdas, no delegates, no LINQ, no events Go Fish using System; namespace GoFish { public enum Suit { Clubs, Diamonds, Hearts, Spades };
C# Programing Language
Given the code below
1.Change all of the low-level arrays in your program to use the appropriate C# collection class instead. Be sure to implement Deck as a Stack
2. Make sure that you use the correct type-safe generic designations throughout your
3.Write code that watches for that incorrect data and throws an appropriate exception if bad data is found.
4. Write Properties instead of Getter and Setter methods.
no lamdas, no delegates, no LINQ, no events
Go Fish
using System;
namespace GoFish
{
public enum Suit { Clubs, Diamonds, Hearts, Spades };
public enum Rank { Ace, Two, Three, Four, Five, Six, Seven, Eight, Nine, Ten, Jack, Queen, King };
// -----------------------------------------------------------------------------------------------------
public class Card
{
public Rank Rank { get; private set; }
public Suit Suit { get; private set; }
public Card(Suit suit, Rank rank)
{
this.Suit = suit;
this.Rank = rank;
}
public override string ToString()
{
return ("[" + Rank + " of " + Suit + "]");
}
}
// -----------------------------------------------------------------------------------------------------
public class Deck
{
private Card[] cards;
private static Random rng = new Random();
public Deck()
{
Array suits = Enum.GetValues(typeof(Suit));
Array ranks = Enum.GetValues(typeof(Rank));
int size = suits.Length * ranks.Length;
cards = new Card[size];
int i = 0;
foreach (Suit suit in suits)
{
foreach (Rank rank in ranks)
{
Card card = new Card(suit, rank);
cards[i++] = card;
}
}
}
public int Size()
{
return cards.Length;
}
public void Shuffle()
{
if (Size() == 0) return;
// Fisher-Yates Shuffle (modern
// - http://en.wikipedia.org/wiki/Fisher%E2%80%93Yates_shuffle
for (int i = 0; i < Size(); i++)
{
int j = rng.Next(i, Size());
Card c = cards[i];
cards[i] = cards[j];
cards[j] = c;
}
}
public void Cut()
{
if (Size() == 0) return;
int cutPoint = rng.Next(1, Size());
Card[] newDeck = new Card[Size()];
int i;
int j = 0;
// Copy the cards at or below the cutpoint into the top of the new deck
for (i = cutPoint; i < Size(); i++)
{
newDeck[j++] = cards[i];
}
for (i = 0; i < cutPoint; i++)
{
newDeck[j++] = cards[i];
}
cards = newDeck;
}
public Card DealCard()
{
if (Size() == 0) return null;
Card card = cards[Size() - 1]; // Deal from bottom of deck (makes Resizing easier)
Array.Resize(ref cards, Size() - 1);
return card;
}
public override string ToString()
{
string s = "[";
string comma = "";
foreach (Card c in cards)
{
s += comma + c.ToString();
comma = ", ";
}
s += "]";
s += "\n " + Size() + " cards in deck.\n";
return s;
}
}
----------------------------------------------------------------------------------
public class Hand
{
private Card[] cards;
public Hand()
{
cards = new Card[0]; // Empty hand
}
public int Size()
{
return cards.Length;
}
public Card[] GetCards()
{
Card[] cardsCopy = new Card[cards.Length];
Array.Copy(cards, cardsCopy, cards.Length);
return cards;
}
public void AddCard(Card card)
{
Array.Resize(ref cards, Size() + 1);
cards[Size() - 1] = card;
}
public Card RemoveCard(Card card)
{
Card[] newCards = new Card[cards.Length - 1];
int i = 0;
foreach (Card c in cards)
{
if (c != card)
newCards[i++] = c;
}
cards = newCards;
return card;
}
public override string ToString()
{
string s = "[";
string comma = "";
foreach (Card c in cards)
{
s += comma + c.ToString();
comma = ", ";
}
s += "]";
return s;
}
}
}
public class Hand
{
private Card[] cards;
public Hand()
{
cards = new Card[0]; // Empty hand
}
public int Size()
{
return cards.Length;
}
public Card[] GetCards()
{
Card[] cardsCopy = new Card[cards.Length];
Array.Copy(cards, cardsCopy, cards.Length);
return cards;
}
public void AddCard(Card card)
{
Array.Resize(ref cards, Size() + 1);
cards[Size() - 1] = card;
}
public Card RemoveCard(Card card)
{
Card[] newCards = new Card[cards.Length - 1];
int i = 0;
foreach (Card c in cards)
{
if (c != card)
newCards[i++] = c;
}
cards = newCards;
return card;
}
public override string ToString()
{
string s = "[";
string comma = "";
foreach (Card c in cards)
{
s += comma + c.ToString();
comma = ", ";
}
s += "]";
return s;
}
}
}
public override string ToString()
{
string s = Name + "'s Hand: ";
s += Hand.ToString();
return s;
}
}
//----------------------------------------------------------------
using System;
namespace GoFish
{
class GoFishApplication
{
private static Deck theDeck;
private static Player[] players;
private static int numTurns = 0;
private static int numBooksPlayed = 0;
public static void Main()
{
Console.WriteLine("Go Fish Simulation (Random players)");
Console.WriteLine("===================================");
theDeck = new Deck();
theDeck.Shuffle();
theDeck.Cut();
players = new Player[4];
players[0] = new RandomPlayer("Paul");
players[1] = new RandomPlayer("Tom");
players[2] = new RandomPlayer("Pat");
players[3] = new RandomPlayer("Susan");
for (int i = 0; i < 5; i++)
{
foreach (Player player in players)
player.AddCardToHand(theDeck.DealCard());
}
foreach (Player player in players)
Console.WriteLine(player);

Step by step
Solved in 2 steps

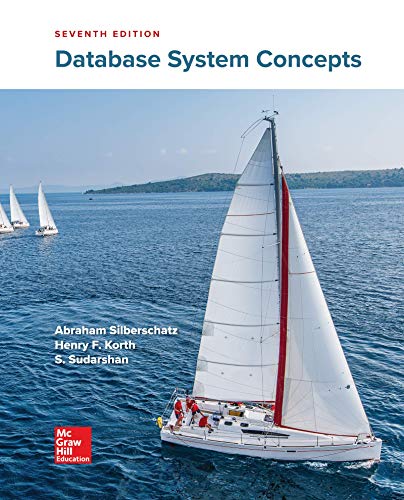
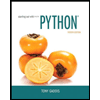
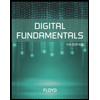
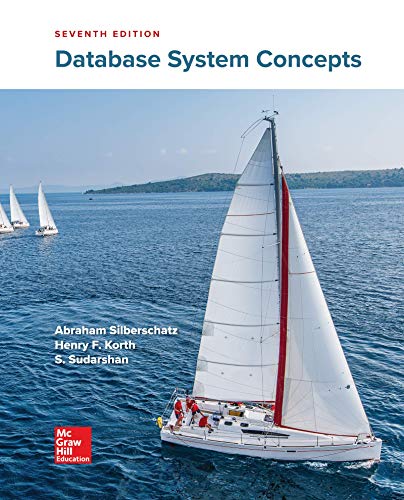
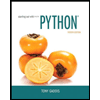
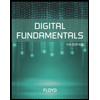
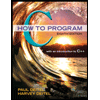
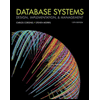
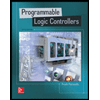