Objectives: to design a good interface to do simple arithmetic, including a running sum to use named constants, rather than hard-coded constants . to learn when to use Static variables to use concatenation to display results Deliverables & Responsibilities: • Hand in a Planning document which shows your plan for all program events (buttons), and the actions you will code for each button. You have been hired by the Calgary Athletic Club to design and program a project to help their members keep track of how many calories they consume in a day, and how much of their diet comes from fat. The Required User Interface The form must have, at a minimum, labels, text boxes, radio buttons and buttons. Do not use MaskedTextBoxes on this assignment. Follow good design guidelines for the user interface as suggested in class and in the text. Even if something is not listed here as a requirement, if it results in a better design, you should do it! Input: The user must input whether she is male or female. The default will be male (since most members of the club are men). The other input is 4 pieces of data for a food item: a brief description, and the number of grams of fat, protein and carbohydrates Output: The recommended maximum total daily calories (for a man this should be 2500, for a woman, 2000), the recommended total calories from fat, and the recommended % of total calories that comes from fat (30%) are displayed. Information displayed about all the food entered so far is the total calories consumed so far, the number of these calories that come from fat, and the % of total calories that comes from fat. A list of all food consumed so far is also displayed (see point 3 below for details) Three buttons are required as described below. The form must have a label in a lower corner with your name(s) The Required Code 1. The Click event procedure for the Male & Female buttons displays the 3 recommended values described above. (Calories, calories from fat and % of total calories) 2. The "New Client" button should clear the form and reset the focus to the first place the user may want to enter data. Male should be the default button. Fat, proteins and carbohydrates should be set to "0" by doing this, if an item contains no protein, there will be no error if the user doesn't enter a value there). Note: you should ensure that these 3 fields have a 0 in them when the form starts, as well. 3. The "Add Item" button will be clicked after all data for a single item of food has been entered. Once it is clicked, the item's calories from fat and total calories will be calculated. (Each gram of fat has 9 calories, each gram of protein has 4 calories, and each gram of carbohydrates has 4 calories). The total calories consumed so far, the number of these calories that come from fat, and the % of total calories that comes from fat will be calculated and displayed. As well, this item's information should be appended to the list of food consumed so far. The format for this list is shown to the right (this example is as it would appear after 5 items have been added. The data entered for line 4 was "enchilada", with grams of fat, carbohydrates and protein as 16, 24 and 20 respectively. Be sure to have spaces and brackets in the line as shown, but don't worry about lining things up nicely). The input data for this item should then be cleared, and focus reset for the next item. The exit button should end the program It must be easy to change all these numeric values (eg. maybe in the future we will want to be more exact, and use decimal places). In other words use named constants. Test your program thoroughly before handing it in. Try it for both men and women. You can run Poorly Designed Calorie Counter.exe to see how it should work, but do not use this as an example of a good design! Cals (Fat) Item 73 (45) potato chips 137 (9) apple 334 (270) avocado 320 (144) enchilada 720 popsicle 4. 5.
Objectives: to design a good interface to do simple arithmetic, including a running sum to use named constants, rather than hard-coded constants . to learn when to use Static variables to use concatenation to display results Deliverables & Responsibilities: • Hand in a Planning document which shows your plan for all program events (buttons), and the actions you will code for each button. You have been hired by the Calgary Athletic Club to design and program a project to help their members keep track of how many calories they consume in a day, and how much of their diet comes from fat. The Required User Interface The form must have, at a minimum, labels, text boxes, radio buttons and buttons. Do not use MaskedTextBoxes on this assignment. Follow good design guidelines for the user interface as suggested in class and in the text. Even if something is not listed here as a requirement, if it results in a better design, you should do it! Input: The user must input whether she is male or female. The default will be male (since most members of the club are men). The other input is 4 pieces of data for a food item: a brief description, and the number of grams of fat, protein and carbohydrates Output: The recommended maximum total daily calories (for a man this should be 2500, for a woman, 2000), the recommended total calories from fat, and the recommended % of total calories that comes from fat (30%) are displayed. Information displayed about all the food entered so far is the total calories consumed so far, the number of these calories that come from fat, and the % of total calories that comes from fat. A list of all food consumed so far is also displayed (see point 3 below for details) Three buttons are required as described below. The form must have a label in a lower corner with your name(s) The Required Code 1. The Click event procedure for the Male & Female buttons displays the 3 recommended values described above. (Calories, calories from fat and % of total calories) 2. The "New Client" button should clear the form and reset the focus to the first place the user may want to enter data. Male should be the default button. Fat, proteins and carbohydrates should be set to "0" by doing this, if an item contains no protein, there will be no error if the user doesn't enter a value there). Note: you should ensure that these 3 fields have a 0 in them when the form starts, as well. 3. The "Add Item" button will be clicked after all data for a single item of food has been entered. Once it is clicked, the item's calories from fat and total calories will be calculated. (Each gram of fat has 9 calories, each gram of protein has 4 calories, and each gram of carbohydrates has 4 calories). The total calories consumed so far, the number of these calories that come from fat, and the % of total calories that comes from fat will be calculated and displayed. As well, this item's information should be appended to the list of food consumed so far. The format for this list is shown to the right (this example is as it would appear after 5 items have been added. The data entered for line 4 was "enchilada", with grams of fat, carbohydrates and protein as 16, 24 and 20 respectively. Be sure to have spaces and brackets in the line as shown, but don't worry about lining things up nicely). The input data for this item should then be cleared, and focus reset for the next item. The exit button should end the program It must be easy to change all these numeric values (eg. maybe in the future we will want to be more exact, and use decimal places). In other words use named constants. Test your program thoroughly before handing it in. Try it for both men and women. You can run Poorly Designed Calorie Counter.exe to see how it should work, but do not use this as an example of a good design! Cals (Fat) Item 73 (45) potato chips 137 (9) apple 334 (270) avocado 320 (144) enchilada 720 popsicle 4. 5.
Programming with Microsoft Visual Basic 2017
8th Edition
ISBN:9781337102124
Author:Diane Zak
Publisher:Diane Zak
Chapter5: The Repetition Structure
Section: Chapter Questions
Problem 14E
Related questions
Question
using Java GUI Interface language

Transcribed Image Text:Objectives:
to design a good interface
to do simple arithmetic, including a running sum
to use named constants, rather than hard-coded constants
to learn when to use Static variables
to use concatenation to display results
Deliverables & Responsibilities:
Hand in a Planning document which shows your plan for all program events (buttons), and the actions you will code for each button.
You have been hired by the Calgary Athletic Club to design and program a project to help their members keep track of how many calories they consume in a day, and how much of their diet comes from fat.
The Required User Interface
The form must have, at a minimum, labels, text boxes, radio buttons and buttons. Do not use MaskedTextBoxes on this assignment. Follow good design guidelines for the user interface as suggested in class and in the text. Even if something is not listed here as a requirement, if it results in a better design, you should do it!
Input: The user must input whether s/he is male or female. The default will be male (since most members of the club are men). The other input is 4 pieces of data for a food item: a brief description, and the number of grams of fat, protein and carbohydrates.
Output: The recommended maximum total daily calories (for a man this should be 2500; for a woman, 2000), the recommended total calories from fat, and the recommended % of total calories that comes from fat (30%) are displayed. Information displayed about all the food entered so far is the total calories consumed so far, the number of
these calories that come from fat, and the % of total calories that comes from fat. A list of all food consumed so far is also displayed (see point 3 below for details)
Three buttons are required as described below. The form must have a label in a lower corner with your name(s).
The Required Code
1. The Click event procedure for the Male & Female buttons displays the 3 recommended values described above. (Calories, calories from fat and % of total calories)
2. The "New Client" button should clear the form and reset the focus to the first place the user may want to enter data. Male should be the default button. Fat, proteins and carbohydrates should be set to "0" by doing this, if an item contains no protein, there will be no error if the user doesn't enter a value there). Note: you should ensure that
these 3 fields have a 0 in them when the form starts, as well.
The “Add Item" button will be clicked after all data for a single item of food has been entered. Once it is clicked, the item's calories from fat and total calories wvill be calculated. (Each gram of fat has 9 calories, each gram of protein has 4 calories, and each gram of carbohydrates has 4 calories). The total
calories consumed so far, the number of these calories that come from fat, and the % of total calories that comes from fat will be calculated and displayed. As well, this item's information should be appended to the list of food consumed so far. The format for this list is shown to the right (this example is as it
would appear after 5 items have been added. The data entered for line 4 was "enchilada", with grams of fat, carbohydrates and protein as 16, 24 and 20 respectively. Be sure to have spaces and brackets in the line as shown, but don't worry about lining things up nicely). The input data for this item should then be
3.
Cals (Fat)
Item
73 (45) potato chips
137 (9) apple
334 (270) avocado
320 (144) enchilada
72 (0) popsicle
cleared, and focus reset for the next item.
The exit button should end the program.
4.
5.
It must be easy to change all these numeric values (e.g. maybe in the future we will want to be more exact, and use decimal places). In other words use named constants.
Test your program thoroughly before handing it in. Try it for both men and women. You can run Poorly Designed Calorie Counter.exe to see how it should work, but do not use this as an example of a good design!
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
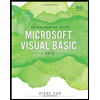
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
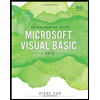
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning