Option: 2 Enter a jersey number: 101 Not in roster Enter a new jersey number: 9 Traceback (most recent call last): File "/Users/jacksonchapin/Project 1.py", line 102, in menu () File "/Users/jacksonchapin/Project 1.py", line 61, in menu del roster[jersey_num] #deletes that jersey number from the dictionary KeyError: 9
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
In python:
Prompt user for 5 inputs from 0-99 for jersey numbers along with 5 input (1-9) for ratings
jersey_num = int(input("Enter a jersey number between 0 and 99: ")) #prompts user for jersey number
rating_num = int(input("Enter a rating between 1 and 9: ")) #prompts user for rating for that jersey number
#Construct while loop to check if prompt is okay (no duplicates and is between 0-99 for jersey number and 1-9 for rating)
roster = {}
count = 1
while count <= 5:
if (jersey_num >= 0) and (jersey_num <= 99) and (rating_num >= 1) and (rating_num <= 9 ):
roster[jersey_num] = rating_num # put it in the dict
count +=1 # update count
jersey_num = int(input("Enter a jersey number between 0 and 99: ")) # get the next entry
rating_num = int(input("Enter a rating between 1 and 9: ")) # get the next entry
if jersey_num in roster: #if statement that checks for duplicates in the roster
print('In roster') #if in roster alrewady, prints this
jersey_num = int(input("Select new jersey number between 0 and 99: ")) #prompts user for a new number)
else:
print('Not in roster') #if if statment fails, then the number is not in the roster
else:
print("Your answer has an incorrect value. Jersey numbers must be between 1 and 99 and ratings must be between 0 and 9. Please try again.")
jersey_num = int(input("Enter a jersey number between 0 and 99: ")) # get the next entry
rating_num = int(input("Enter a rating between 1 and 9: ")) # get the next entry
#Print print the roster
print(roster)
#Create a menu to add a player, remove a player, change a rating, output layers above a certain rating, output roster and exit
def menu():
print("Select an option: \n 1. Add a player \n 2. Remove a player \n 3. Change a player's rating \n 4. Output players above a certain rating \n 5. Output the roster \n 6. Exit")
choice = input("Option: ") #selects which option for menu to run
if choice == "1":
new_jersey = int(input("Enter a new jersey number: ")) #gets new, unique player number
new_rating = int(input("Enter the corresponding rating: ")) #gives the jersey number a corresponding rating
while (new_jersey < 0) or (new_jersey > 99):
print('Out of range')
new_jersey = int(input("Enter a new jersey number: ")) #gets new, unique player number
while (new_rating < 1) or (new_rating > 9):
print('Out of range')
new_rating = int(input("Enter the corresponding rating: ")) #gives the jersey number a corresponding rating
if new_jersey in roster: #find duplicates in roster
print('Already in roster')
new_jersey = int(input("Enter a new jersey number: ")) #prompts user for new jersey number if already in roster
else:
print('Not in roster')
roster[new_jersey] = new_rating #adds player to dictionary if player not already in there
print(roster)
if choice == "2":
jersey_num = int(input("Enter a jersey number: ")) #selects a jersey number from user
if jersey_num in roster: #find duplicates in roster
print('In roster')
else:
print('Not in roster')
jersey_num = int(input("Enter a new jersey number: ")) #prompts user for new jersey number if already in roster
del roster[jersey_num] #deletes that jersey number from the dictionary
print(roster) #prints the updated roster
if choice == "3":
jersey_num = int(input("Enter a new jersey number: ")) #gets new, unique player number
new_rating = int(input("Enter the corresponding rating: ")) #gives the jersey number a corresponding rating
while (jersey_num < 0) or (jersey_num > 99):
print('Out of range')
jersey_num = int(input("Enter a new jersey number: ")) #gets new, unique player number
else:
print("in range")
while (new_rating < 1) or (new_rating > 9):
print('Out of range')
new_rating = int(input("Enter the corresponding rating: ")) #gives the jersey number a corresponding rating
else:
print('In range')
if jersey_num in roster: #find duplicates in roster
print('Already in roster')
jersey_num = int(input("Enter a new jersey number: ")) #prompts user for new jersey number if already in roster
else:
print('Not in roster')
roster[jersey_num] = new_rating #attaches the new rating to the selected jersey number
print(roster) #prints new roster
if choice == '4':
val = int(input("Enter rating to be compared to (1-9): ")) #gets value to compare ratings to
while val > 9 or val < 0:
print ('Out of range')
val = int(input("Enter the new rating between 1 and 9: ")) #prompts user for new rating
else:
print('In range')
output = dict((k,i) for k, i in roster.items() if i > val) #looks at key-value pairings in roster and evaluates if ratings (i) is above a certain rating then stores it into dictionary output
print(output) #prints the key-value pairings found in line 51
if choice == '5':
print(roster) #prints roster
if choice == '6':
quit #stops the menu from running
menu()
Choice 2 in menu is the main question: If the first number is not in the roster, the user is prompted for another jersey number but when that one is also not in the roster, the code fails and there is an error message. How do I fix this code so that no matter how many numbers it prompts for that will keep asking until they are in the dictionary?
![Option: 2
Enter a jersey number: 101
Not in roster
Enter a new jersey number: 9
Traceback (most recent call last):
File "/Users/jacksonchapin/Project 1.py", line 102, in <module>
menu ()
File "/Users/jacksonchapin/Project 1.py", line 61, in menu
del roster[jersey_num] #deletes that jersey number from the dictionary
KeyError: 9](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3b875ac8-882b-4ab8-aa9e-a70d3b1e2dfc%2Fa47e8ad7-1898-4c7b-8868-d7b33bb2c01d%2F1uz2ji.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 8 images

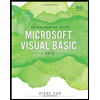
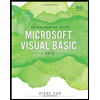