Overview Construct a hierarchy of classes representing savings and checking accounts as well as a means to ‘filter’ account objects. Account Classes Your solution will consist of 4 account classes as described below. It is your responsibility to define the exact relationship among those classes and translate that relationship into source code structure. There is a correct structure; the skeleton must be modified to reflect the correct relationships. Account – represents a general account with basic operations such as deposit, withdraw, etc. Savings – represents a savings account that must maintain a minimum balance. Checking – represents a checking account that has no minimum balance (this means a zero balance). CappedChecking – represents a checking account that disallows a balance above a particular maximum value. Linking Accounts
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Overview
Construct a hierarchy of classes representing savings and checking accounts as well as a means to ‘filter’
account objects.
Account Classes
Your solution will consist of 4 account classes as described below. It is your responsibility to define the
exact relationship among those classes and translate that relationship into source code structure. There is a
correct structure; the skeleton must be modified to reflect the correct relationships.
Account – represents a general account with basic operations such as deposit, withdraw, etc.
Savings – represents a savings account that must maintain a minimum balance.
Checking – represents a checking account that has no minimum balance (this means a zero
balance).
CappedChecking – represents a checking account that disallows a balance above a particular
maximum value.
Linking Accounts
A bank or credit typically allows a user the ability to link accounts together. That is, I might wish to link
my checking account with my savings account. If I overdraw from my savings account, then the appropriate
amount of money is transferred from my savings account into my checking account to prevent against the
overdraw. In this program we wish to simulate this concept with the following requirements:
A checking account may only be linked to a savings account (and vice versa).
An account may only be linked to one other account.
An account may not be linked to itself.
An account may exist without a link to another account.
In this program, we consider a link to be bidirectional; that is, if account A is linked to account B, then B
is only linked to account A. If the user wishes to link account C to A, then the bidirectional link from A to
B must be unlinked before A is then linked to C.
Depositing / Withdrawing Funds and Exceptions
In this program we will use the following rule for deposits: if a deposit operation is unsuccessful a
LinkAccountException will be thrown. For example, if a deposit exceeds the maximum amount
allowed in the account and the account is not linked, an exception will be thrown. If the account is linked,
the remaining monies will be transferred to the linked account.
If a withdrawal operation is unsuccessful an InsufficientFundsException will be thrown. For
example, if a withdrawal exceeds the minimum of an account (and its linked account), an exception shall
be thrown. In the case that a withdrawal results in an account dropping below the minimum, an appropriate
amount of funds shall be transferred from the linked account to the current account in order to attain the
minimum balance.
Filtering
In
project, each account will be labeled as Filterable. That is, you must appropriately define an abstract
In java



Trending now
This is a popular solution!
Step by step
Solved in 2 steps

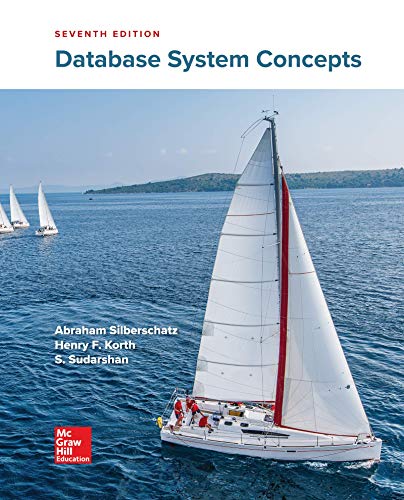
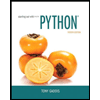
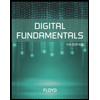
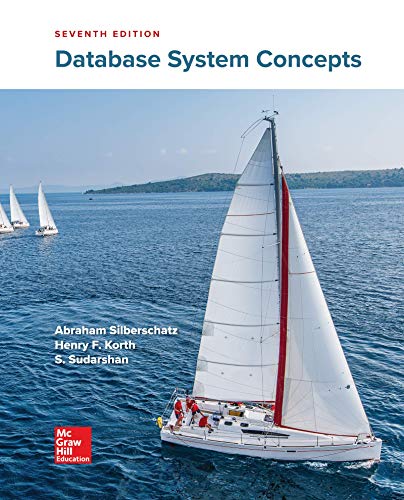
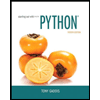
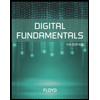
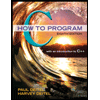
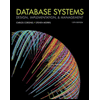
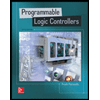