Please answer in C++ and give explanation LAB 17.1-B Linked List Read the internal documentation for class ItemList carefully. Fill in the missing code in the implementation file, being careful to adhere to the preconditions and postconditions. Compile your program. ------------------------------------------------------------------------------------------------------------------------------- class ItemList { private: struct ListNode { int value; ListNode * next; }; ListNode * head; public: ItemList(); // Post: List is the empty list. bool IsThere(int item) const; // Post: If item is in the list IsThere is // True; False, otherwise. void Insert(int item); // Pre: item is not already in the list. // Post: item is in the list. void Delete(int item); // Pre: item is in the list. // Post: item is no longer in the list. void Print() const; // Post: Items on the list are printed on the screen. int GetLength() const; // Post: Length is equal to the number of items in the // list. ~ItemList(); // Post: List has been destroyed. }; ------------------------------------------------------------------------------------------------------------------------------- Exercise 1: Write the definitions for the member functions. Save the code in file ItemList.cpp. Exercise 2: Write a driver program that reads values from file int.dat, inserts them into the list, print the length of the final list, and prints the items. Be sure that your driver checks for the preconditions on the member functions of class ItemList before calling them. How many items are in the list? If your answer is not 11, your driver did not adhere to the preconditions. Correct your driver and return your program. Exercise 3: Add code to your driver to test the remaining member functions. Delete -47, 1926, and 2000 and print the list to confirm that they are gone.
Please answer in C++ and give explanation LAB 17.1-B Linked List Read the internal documentation for class ItemList carefully. Fill in the missing code in the implementation file, being careful to adhere to the preconditions and postconditions. Compile your program. ------------------------------------------------------------------------------------------------------------------------------- class ItemList { private: struct ListNode { int value; ListNode * next; }; ListNode * head; public: ItemList(); // Post: List is the empty list. bool IsThere(int item) const; // Post: If item is in the list IsThere is // True; False, otherwise. void Insert(int item); // Pre: item is not already in the list. // Post: item is in the list. void Delete(int item); // Pre: item is in the list. // Post: item is no longer in the list. void Print() const; // Post: Items on the list are printed on the screen. int GetLength() const; // Post: Length is equal to the number of items in the // list. ~ItemList(); // Post: List has been destroyed. }; ------------------------------------------------------------------------------------------------------------------------------- Exercise 1: Write the definitions for the member functions. Save the code in file ItemList.cpp. Exercise 2: Write a driver program that reads values from file int.dat, inserts them into the list, print the length of the final list, and prints the items. Be sure that your driver checks for the preconditions on the member functions of class ItemList before calling them. How many items are in the list? If your answer is not 11, your driver did not adhere to the preconditions. Correct your driver and return your program. Exercise 3: Add code to your driver to test the remaining member functions. Delete -47, 1926, and 2000 and print the list to confirm that they are gone.
Programming Logic & Design Comprehensive
9th Edition
ISBN:9781337669405
Author:FARRELL
Publisher:FARRELL
Chapter8: Advanced Data Handling Concepts
Section: Chapter Questions
Problem 10RQ
Related questions
Question
Please answer in C++ and give explanation
LAB 17.1-B Linked List
Read the internal documentation for class ItemList carefully. Fill in the missing code in the implementation file, being careful to adhere to the preconditions and postconditions. Compile your program.
-------------------------------------------------------------------------------------------------------------------------------
class ItemList
{
private:
struct ListNode
{
int value;
ListNode * next;
};
ListNode * head;
public:
ItemList();
// Post: List is the empty list.
bool IsThere(int item) const;
// Post: If item is in the list IsThere is
// True; False, otherwise.
void Insert(int item);
// Pre: item is not already in the list.
// Post: item is in the list.
void Delete(int item);
// Pre: item is in the list.
// Post: item is no longer in the list.
void Print() const;
// Post: Items on the list are printed on the screen.
int GetLength() const;
// Post: Length is equal to the number of items in the
// list.
~ItemList();
// Post: List has been destroyed.
};
-------------------------------------------------------------------------------------------------------------------------------
Exercise 1: Write the definitions for the member functions. Save the code in file ItemList.cpp.
Exercise 2: Write a driver program that reads values from file int.dat, inserts them into the list, print the length of the final list, and prints the items. Be sure that your driver checks for the preconditions on the member functions of class ItemList before calling them. How many items are in the list? If your answer is not 11, your driver did not adhere to the preconditions. Correct your driver and return your program.
Exercise 3: Add code to your driver to test the remaining member functions. Delete -47, 1926, and 2000 and print the list to confirm that they are gone.

Transcribed Image Text:int - Notepad
File Edit Format View Help
1492
1776
1860
-23
1698
1935
1926
1953
1960
1492
1776
1860
-23
1698
2000
1935
1926
1953
1960
-47

Transcribed Image Text:Sample Run:
1492 is not in the list
1776 is not in the list
1860 is not in the list
23 is not in the list
1698 is not in the list
1935 is not in the list
1926 is not in the list
1953 is not in the list
1960 is not in the list
1492 is already in the list
1776 is already in the list
1860 is already in the list
-23 is already in the list
1698 is already in the list
2000 is not in the list
1935 is already in the list
1926 is already in the list
1953 is already in the list
1960 is already in the list
47 is not in the list
There are 11 items in the list.
F47
-23
1492
1698
1776
1860
1926
1935
1953
1960
2000
After deletion, there are 8 items in the list.
23
1492
1698
1776
1860
1935
1953
1960
Press any ke y to continue
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
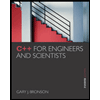
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
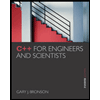
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr