please code in python Please create a class called PlayingCard. This class should have: An attribute, "rank" that takes a value of "2", "3", "4", "5", "6", "7", "8", "9", "10", "J", "Q", "K", or "A" An attribute, "suit" that takes a value of "♠" "♥" "♦" or "♣". (If you don't know how to make these characters you can cut and paste from this block) An init method that: Accepts as parameters
please code in python Please create a class called PlayingCard. This class should have: An attribute, "rank" that takes a value of "2", "3", "4", "5", "6", "7", "8", "9", "10", "J", "Q", "K", or "A" An attribute, "suit" that takes a value of "♠" "♥" "♦" or "♣". (If you don't know how to make these characters you can cut and paste from this block) An init method that: Accepts as parameters
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
please code in python
Please create a class called PlayingCard.
This class should have:
- An attribute, "rank" that takes a value of "2", "3", "4", "5", "6", "7", "8", "9", "10", "J", "Q", "K", or "A"
- An attribute, "suit" that takes a value of "♠" "♥" "♦" or "♣". (If you don't know how to make these characters you can cut and paste from this block)
- An init method that:
- Accepts as parameters a specific rank (as a string) and suit (as a string).
- Raise a TypeError with "Invalid rank!" or "Invalid suit!" when a rank or suit is not valid.
- The __str__ and __repr__ methods to display the cards correctly (as shown below in the examples)
2. Please create a class called Deck.
This class should have:
-
An attribute, "cards", that holds a list of PlayingCard objects.
-
An init method that:
- By default stores a full deck of 52 playing card (with proper numbers and suits) in the "cards" list. Each cards will be of the class PlayingCard above
- Allows the user to specify a specific suit of the 4 ("♠" "♥" "♦" or "♣"). In this case, the program should only populate the deck with the 13 cards of that suit.
- After the cards object is initialized, call the "shuffle_deck()" function (below).
-
A "shuffle_deck()" method that randomly changes the order of cards in the deck.
- Import the random library to 'shuffle' the deck: https://docs.python.org/3.9/library/random.html#random.shuffle
- Please import it at the top of your block instead of inside the class / methods.
-
A "deal_card(card_count)" method that:
- Removes the first card_count cards from the deck and returns them as a list.
- If the deck doesnt have the card_count number of cards left to deal, return the message Cannot deal <x> cards. The deck only has <y> cards left! (do not raise an exception or print inside the method).
![Example:
>>> card1 = PlayingCard ("A", "4")
>>> print (card1)
A of 4
>>> card2 = PlayingCard ("15", "4")
<error stack >
TypeError: Invalid rank!
>>> card2 = PlayingCard ("10", "bunnies")
<error stack >
TypeError: Invalid suit!
>>> deck1 = Deck()
>>> print (deck1.cards)
[K of, A of ♥, 6 of 4, 7 of , 3 of 4, 6 of , Q of , 5 of+, 10 of, 2 of ♥, 8 of 4, 8 of , 4 of , 7 of, 3 of
+, K of +, 9 of , 4 of ♥, 10 of ♥, 10 of , A of , 9 of ♥, 7 of , 9 of +, 7 of +, 5 of , 3 of , 10 of , Q of ♥, J
of , 5 of ♥, K of ♥, K of
, 4 of 4, 5
of , 2 of, 4 of 4, 2 of
, 2 of 4, 8 of 4, Q of , 3 of 4, 6 of ♥, 6 of, A of +, A of, 3 of ♥, J of
, Q of 4, J of ♥, 8 of ♥, 9 of ]
>>> deck2 = Deck('')
>>> deck2.shuffle_deck()
>>> deck2.cards
[A of, 10 of , 3 of 4, 7 of , 5 of , 4 of 4, 8 of 4, 3 of 4, 9 of , Q of 4, 6 of 4, 2 of 4, K of 4]
>>> hand = deck2.deal_card (7)
>>> hand
[A of, 10 of 4, 3 of 4, 7 of 4, 5 of 4, 4 of 4, 8 of 4]
>>> deck2.deal_card(7)
'Cannot deal 7 cards. The deck only has 6 cards left!'](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Feb1193c9-d0d1-416d-ac45-bceed8a4dd09%2F0a63d9f1-c64d-43c3-a520-692cd72fd9dc%2Fzo1e5j_processed.png&w=3840&q=75)
Transcribed Image Text:Example:
>>> card1 = PlayingCard ("A", "4")
>>> print (card1)
A of 4
>>> card2 = PlayingCard ("15", "4")
<error stack >
TypeError: Invalid rank!
>>> card2 = PlayingCard ("10", "bunnies")
<error stack >
TypeError: Invalid suit!
>>> deck1 = Deck()
>>> print (deck1.cards)
[K of, A of ♥, 6 of 4, 7 of , 3 of 4, 6 of , Q of , 5 of+, 10 of, 2 of ♥, 8 of 4, 8 of , 4 of , 7 of, 3 of
+, K of +, 9 of , 4 of ♥, 10 of ♥, 10 of , A of , 9 of ♥, 7 of , 9 of +, 7 of +, 5 of , 3 of , 10 of , Q of ♥, J
of , 5 of ♥, K of ♥, K of
, 4 of 4, 5
of , 2 of, 4 of 4, 2 of
, 2 of 4, 8 of 4, Q of , 3 of 4, 6 of ♥, 6 of, A of +, A of, 3 of ♥, J of
, Q of 4, J of ♥, 8 of ♥, 9 of ]
>>> deck2 = Deck('')
>>> deck2.shuffle_deck()
>>> deck2.cards
[A of, 10 of , 3 of 4, 7 of , 5 of , 4 of 4, 8 of 4, 3 of 4, 9 of , Q of 4, 6 of 4, 2 of 4, K of 4]
>>> hand = deck2.deal_card (7)
>>> hand
[A of, 10 of 4, 3 of 4, 7 of 4, 5 of 4, 4 of 4, 8 of 4]
>>> deck2.deal_card(7)
'Cannot deal 7 cards. The deck only has 6 cards left!'
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
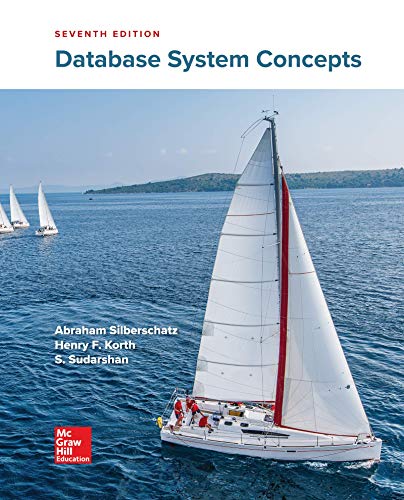
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
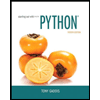
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
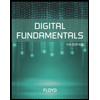
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
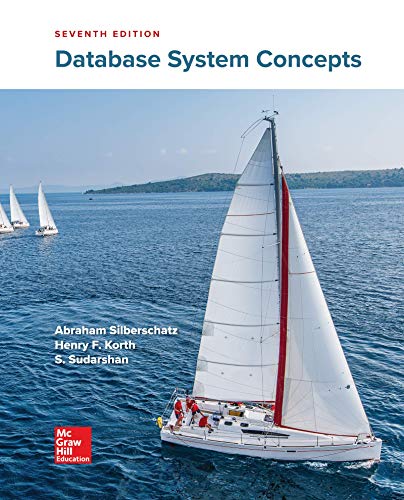
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
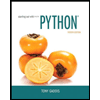
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
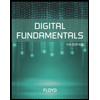
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
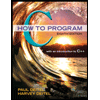
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
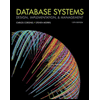
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
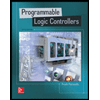
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education