Please fill in the blanks from 26 to 78 in C. /*This program will print students’ information and remove students using linked list*/ #include #include //Declare a struct student (node) with 5 members
Please fill in the blanks from 26 to 78 in C.
/*This program will print students’ information and remove students using linked list*/
#include<stdio.h>
#include<stdlib.h>
//Declare a struct student (node) with 5 members
//each student node contains an ID, an age, graduation year,
// 1 pointer points to the next student, and 1 pointer points to the previous student.
struct student
{
int ID;
int age;
int classOf;
struct student* next;
struct student* prev;
};
/* - This function takes the head pointer and iterates through the list to get all 3 integers needed from the user, and save it to our list - Update the pointer to point to the next node.*/
void scanLL(struct student* head)
{
while(head != NULL) //this condition makes sure the list is not at the end
{
printf("Lets fill in the information. Enter 3 integers for the student's ID, age, and graduation year: ");
scanf("%i %i %i",&&head->ID, &head->age, &head->classOf); //in order: ID, age, graduation year
head = head->next;
}
}
/*This function prints the list forwards or backwards depends on the flag. If flag is 1, we want to print forward If flag is 0, we want to print backwards. Depends on such flag, we will set our link to move to next or previous student*/
void printLL(struct student* head, int flag)
{
while(head != NULL)
{
printf("ID: %5d.\tAge: %3d.\tClass Of: %4d.\n\n",head->ID, head->age, head->classOf);
//Compare the flag to update the pointer. If flag is 1, we want to move forward
// else, we will move backwards.
if(flag == 1) head = head->next; //forwards
else head = head->prev; //backwards
}
}
/*This function will take an address of the node we need to remove/ Regardless of the size of our linked list, there are only 3 cases. Case 1: If the address to remove is the first student in the list: We need to set s2 (2nd student) as first in list Case 2: If the address to remove is the last student in the list. We need to set student before last as last in list Case 3: Any other students in the middle of the list We need to take care of both directions. For ex: to remove student s3, we'll need to set s2.next to be s4 and (A) s4.previous to be s2 (B) */
void removeNode(__26__ __27__ stu2Rm)
{
//removing first node, we just need to set its next node as first node
if(__28__ == NULL) // check if it's first node
__29__ -> __30__ -> __31__ = __32__;
//removing last node, we just need to set its previous node as last node
else if(__33__ == NULL) //check if it's last node
__34__ -> __35__ -> __36__ = __37__;
//if it's any other node, we need to update both of its previous and next
else //every node in between
{
__38__ -> __39__ -> __40__ = __41__; //update the previous link Case 3(A)
__42__ -> __43__ -> __44__ = __45__; //update the next link Case 3(B)
}
}
int main()
{
//Create 4 character nodes, and link a head pointer to first node.
struct student s1, s2, s3, s4;
struct __46__ head = __47__;
//Link all nodes to its next, no spaces
// we'll do in this order s1 -> s2 -> s3 -> s4
__48__ = __49__; //make a link from s1 to s2
__50__ = __51__; //make a link from s2 to s3
__52__ = __53__; //make a link from s3 to s4
__54__ = __55__; //make a link to show s4 is the end
//Link all nodes to its previous, no spaces
// we'll do in this order s4 -> s3 -> s2 -> s1
__56__ = __57__; //make a link from s4 to s3
__58__ = __59__; //make a link from s3 to s2
__60__ = __61__; //make a link from s2 to s1
__62__ = __63__; //make a link to show s1 is the end
scanLL(head); //fill in the students' info in our list
printf("\nPrinting forwards:\n");
printLL(__64__ , __65__); //print back the student's info from the list. Check function header to parameters //Printing backwards
head = __66__; //set the start of the list
printf("\nPrinting backwards:\n");
printLL(__67__ , __68__);
//Get a student to remove
int node2Rm;
printf("\nEnter a node to remove. 1 for s1 through 4 for s4.\n");
scanf("%i", &node2Rm);
struct student* rmNode; //this is used to check to print again after removing a node
switch (node2Rm)
{
//rmNode will save the address of the node needed to be removed
case 1:
rmNode = __69__;
break;
case 2:
rmNode =__70__;
break;
case 3:
rmNode = __71__;
break;
case 4:
rmNode = __72__;
break;
default:
printf("Invalid choice. Bye\n");
exit(0);
}
printf("Removing node: \n");
removeNode(rmNode); //remove the node
/*To print back, we need to set the head pointer
To print forwards, we want to check if the node we just removed is s1, if not, set head to s1. if it is, we need to set head to s2.
Same to print backwards, but with s4 instead.*/
//Forwards print
head = (rmNode == &s1) ? __73__ : __74__;
printf("\nPrinting forwards AFTER removing a node:\n");
printLL(__75__,__76__); //remember the flag to print forwards
//Backwards print
head = (rmNode == &s4) ? __77__ : __78__;
printf("\nPrinting backwards AFTER removing a node:\n");
printLL(__79__,__80__); //remember the flag to print backwards
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images

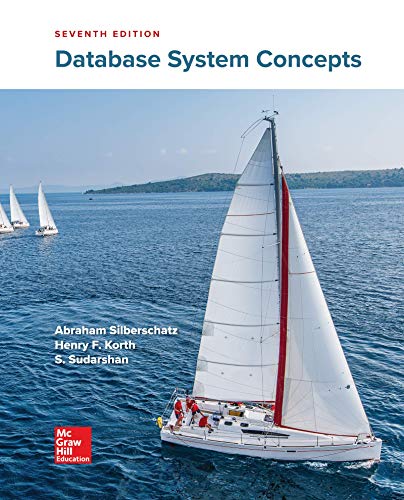
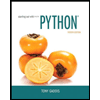
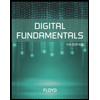
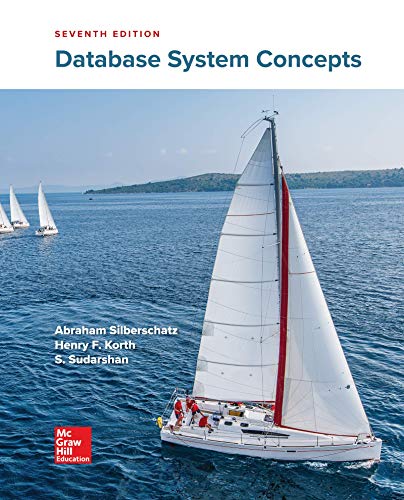
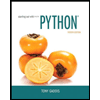
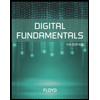
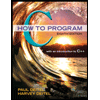
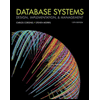
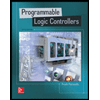