Please help, and please use the functions provided (in python) – Refer to questions attached in the images, and the starter code below (I cannot upload page 3 with example outputs for removeDuplicates(self)). Do not change the function names or given starter code in your script. You are not allowed to use any other data structures for the purposes of manipulating/sorting elements, nor may you use any modules from the Python to insert and remove elements from the list. You are not allowed to swap data from the nodes when adding the node to be sorted. Traversing the linked list and updating ‘next’ references are the only required and acceptable operations. You are not allowed to use any kind of built-in sorting method or import any other libraries. If you are unable to complete a function, use the pass statement to avoid syntax errors. Starter code - class Node: def __init__(self, value): self.value = value self.next = None def __str__(self): return "Node({})".format(self.value) __repr__ = __str__ class SortedLinkedList: ''' >>> x=SortedLinkedList() >>> x.add(8.76) >>> x.add(1) >>> x.add(1) >>> x.add(1) >>> x.add(5) >>> x.add(3) >>> x.add(-7.5) >>> x.add(4) >>> x.add(9.78) >>> x.add(4) >>> x Head:Node(-7.5) Tail:Node(9.78) List:-7.5 -> 1 -> 1 -> 1 -> 3 -> 4 -> 4 -> 5 -> 8.76 -> 9.78 >>> x.replicate() Head:Node(-7.5) Tail:Node(9.78) List:-7.5 -> -7.5 -> 1 -> 1 -> 1 -> 3 -> 3 -> 3 -> 4 -> 4 -> 4 -> 4 -> 4 -> 4 -> 4 -> 4 -> 5 -> 5 -> 5 -> 5 -> 5 -> 8.76 -> 8.76 -> 9.78 -> 9.78 >>> x Head:Node(-7.5) Tail:Node(9.78) List:-7.5 -> 1 -> 1 -> 1 -> 3 -> 4 -> 4 -> 5 -> 8.76 -> 9.78 >>> x.removeDuplicates() >>> x Head:Node(-7.5) Tail:Node(9.78) List:-7.5 -> 1 -> 3 -> 4 -> 5 -> 8.76 -> 9.78 ''' def __init__(self): # You are not allowed to modify the constructor self.head=None self.tail=None def __str__(self): # You are not allowed to modify this method temp=self.head out=[] while temp: out.append(str(temp.value)) temp=temp.next out=' -> '.join(out) return f'Head:{self.head}\nTail:{self.tail}\nList:{out}' __repr__=__str__ def isEmpty(self): return self.head == None def __len__(self): count=0 current=self.head while current: current=current.next count+=1 return count def add(self, value): # --- YOUR CODE STARTS HERE pass def replicate(self): # --- YOUR CODE STARTS HERE pass def removeDuplicates(self): # --- YOUR CODE STARTS HERE pass -page 3 continued- removeDuplicates(self) Removes any duplicate nodes from the list, so it modifies the original list. This method must traverse the list only once. It modifies the original list. Output None Nothing is returned when the method completes the work Examples: >>> x=SortedLinkedList() >>> x.removeDuplicates() >>> x Head:None Tail:None List: >>> x.add(1) >>> x.add(1) >>> x.add(1) >>> x.add(1) >>> x Head:Node(1) Tail:Node(1) List:1 -> 1 -> 1 -> 1 >>> x.removeDuplicates() >>> x Head:Node(1) Tail:Node(1) List:1 >>> x.add(1) >>> x.add(2) >>> x.add(2) >>> x.add(2) >>> x.add(3) >>> x.add(4) >>> x.add(5) >>> x.add(5) >>> x.add(6.7) >>> x.add(6.7) >>> x Head:Node(1) Tail:Node(6.7) List:1 -> 1 -> 2 -> 2 -> 2 -> 3 -> 4 -> 5 -> 5 -> 6.7 -> 6.7 >>> x.removeDuplicates() >>> x Head:Node(1) Tail:Node(6.7) List:1 -> 2 -> 3 -> 4 -> 5 -> 6.7
Please help, and please use the functions provided (in python) – Refer to questions attached in the images, and the starter code below (I cannot upload page 3 with example outputs for removeDuplicates(self)). Do not change the function names or given starter code in your script. You are not allowed to use any other data structures for the purposes of manipulating/sorting elements, nor may you use any modules from the Python to insert and remove elements from the list. You are not allowed to swap data from the nodes when adding the node to be sorted. Traversing the linked list and updating ‘next’ references are the only required and acceptable operations. You are not allowed to use any kind of built-in sorting method or import any other libraries. If you are unable to complete a function, use the pass statement to avoid syntax errors.
Starter code -
def __init__(self, value):
self.value = value
self.next = None
def __str__(self):
return "Node({})".format(self.value)
__repr__ = __str__
class SortedLinkedList:
'''
>>> x=SortedLinkedList()
>>> x.add(8.76)
>>> x.add(1)
>>> x.add(1)
>>> x.add(1)
>>> x.add(5)
>>> x.add(3)
>>> x.add(-7.5)
>>> x.add(4)
>>> x.add(9.78)
>>> x.add(4)
>>> x
Head:Node(-7.5)
Tail:Node(9.78)
List:-7.5 -> 1 -> 1 -> 1 -> 3 -> 4 -> 4 -> 5 -> 8.76 -> 9.78
>>> x.replicate()
Head:Node(-7.5)
Tail:Node(9.78)
List:-7.5 -> -7.5 -> 1 -> 1 -> 1 -> 3 -> 3 -> 3 -> 4 -> 4 -> 4 -> 4 -> 4 ->
4 -> 4 -> 4 -> 5 -> 5 -> 5 -> 5 -> 5 -> 8.76 -> 8.76 -> 9.78 -> 9.78
>>> x
Head:Node(-7.5)
Tail:Node(9.78)
List:-7.5 -> 1 -> 1 -> 1 -> 3 -> 4 -> 4 -> 5 -> 8.76 -> 9.78
>>> x.removeDuplicates()
>>> x
Head:Node(-7.5)
Tail:Node(9.78)
List:-7.5 -> 1 -> 3 -> 4 -> 5 -> 8.76 -> 9.78
'''
def __init__(self): # You are not allowed to modify the constructor
self.head=None
self.tail=None
def __str__(self): # You are not allowed to modify this method
temp=self.head
out=[]
while temp:
temp=temp.next
out=' -> '.join(out)
return f'Head:{self.head}\nTail:{self.tail}\nList:{out}'
__repr__=__str__
def isEmpty(self):
return self.head == None
def __len__(self):
count=0
current=self.head
while current:
current=current.next
count+=1
return count
def add(self, value):
# --- YOUR CODE STARTS HERE
pass
def replicate(self):
# --- YOUR CODE STARTS HERE
pass
def removeDuplicates(self):
# --- YOUR CODE STARTS HERE
pass
Removes any duplicate nodes from the list, so it modifies the original list. This method must traverse the list only once. It modifies the original list.
Output
None Nothing is returned when the method completes the work
>>> x=SortedLinkedList()
>>> x.removeDuplicates()
>>> x
Head:None
Tail:None
List:
>>> x.add(1)
>>> x.add(1)
>>> x.add(1)
>>> x.add(1)
>>> x
Head:Node(1)
Tail:Node(1)
List:1 -> 1 -> 1 -> 1
>>> x.removeDuplicates()
>>> x
Head:Node(1)
Tail:Node(1)
List:1
>>> x.add(1)
>>> x.add(2)
>>> x.add(2)
>>> x.add(2)
>>> x.add(3)
>>> x.add(4)
>>> x.add(5)
>>> x.add(5)
>>> x.add(6.7)
>>> x.add(6.7)
>>> x
Head:Node(1)
Tail:Node(6.7)
List:1 -> 1 -> 2 -> 2 -> 2 -> 3 -> 4 -> 5 -> 5 -> 6.7 -> 6.7
>>> x.removeDuplicates()
>>> x
Head:Node(1)
Tail:Node(6.7)
List:1 -> 2 -> 3 -> 4 -> 5 -> 6.7



Find an implementation below.
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

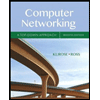
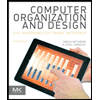
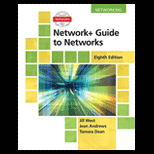
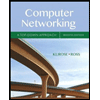
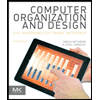
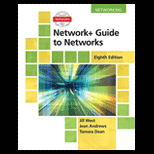
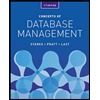
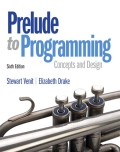
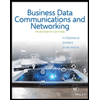