Please help me design a Airworthy class in C++. Develop a high-quality, object-oriented C++ program that performs a simulation using a heap implementation of a priority queue. A simulation creates a model of a real-world situation, allowing us to introduce a variety of conditions and observe their effects. For instance, a flight simulator challenges a pilot to respond to varying conditions and measures how well the pilot responds. Simulation is frequently used to measure current business practices, such as the number of checkout lines in a grocery store or the number of tellers in a bank, so that management can determine the fewest number of employees required to meet customer needs. Airlines have been experimenting with different boarding procedures to shorten the entire boarding time, keep the flights on-time, reduce aisle congestion, and make the experience more pleasant for passengers and crew. A late-departing flight can cause a domino effect: the departure gate is tied up and cannot be used by other landing or departing flights, passengers on board the late flight may miss connecting flights and require rebooking and possibly overnight arrangements (meals and lodging) passengers complain about being late, and/or about having to rearrange their plans. Thus, late flights have a huge operational impact. For this assignment, we will simulate boarding procedures for Airworthy Airlines. The Airline's current procedure is as follows: pre-board in the following order: families with young children or people who need help (e.g., wheelchair) first class and/or business class passengers elite passengers (frequent fliers) and those passengers seated in exit rows conduct general boarding in reverse, from the back of the plane to the front in the following order: rows 23-26 rows 17-22 rows 11-16 rows 5-10 Airworthy is considering revising their boarding procedure such that general boarding is done randomly, meaning the first passenger in line for general boarding is the first passenger to board (i.e., general boarding passengers all have the same priority). Airworthy suspects this random general boarding method will improve the flow of passengers, getting them on board and seated more quickly. It is also less labor-intensive for Airworthy's customer service agents because it significantly reduces the number of boarding announcements required and eliminates confrontations with customers trying to board "out of turn." Note that the revision is to general boarding only. The pre-boarding procedures will not be changed. Develop an Airworthy class that contains the following data members: exactly one priority queue where the priority is set using Airworthy's previous boarding procedure exactly one priority queue where the priority is set using Airworthy's new random boarding procedure the amount of time, in seconds, required to board a plane using Airworthy's previous boarding procedure the amount of time, in seconds, required to board a plane using Airworthy's new random boarding procedure You may use additional attributes, as needed. The Airworthy class must include methods to support the following functions of the class: a constructor read the data from the input file load the two priority queues run the simulation When running the simulation, assume that: A passenger who is not blocked by another passenger requires one second to board. A passenger is blocked when the previous passenger is sitting in the same row or a row closer to the front of the plane. A blocked passenger requires 25 seconds to board HeapException.cpp: #include "HeapException.h" HeapException::HeapException(const string& msg) : logic_error("Heap Exception: " + msg) { } HeapException.h: #ifndef HEAPEXCEPTION_H #define HEAPEXCEPTION_H #include #include using namespace std; class HeapException : public logic_error { public: HeapException(const string& msg = ""); }; #endif /* HEAPEXCEPTION_H */ HeapADT: #ifndef HEAPADT_H #define HEAPADT_H template class HeapADT { public: virtual bool isEmpty() const = 0; virtual int getItemCount() const = 0; virtual T peek() const = 0; virtual bool add(const T& newItem) = 0; virtual bool remove() = 0; virtual void clear() = 0; }; #endif /* HEAPADT_H */ ArrayMaxHeap.h: #ifndef ARRAYMAXHEAP_H #define ARRAYMAXHEAP_H #include "HeapADT.h" template class ArrayMaxHeap : public HeapADT { public: ArrayMaxHeap(); virtual ~ArrayMaxHeap(); bool isEmpty() const; int getItemCount() const; T peek() const; bool add(const T& newItem); bool remove(); void clear(); private: static const int ROOT_INDEX = 0; static const int CAPACITY = 5000; int itemCount; T* items; int getLeftChild(int currNode) const; int getRightChild(int currNode) const; int getParent(int currNode) const; bool isLeaf(int currNode) const; void percolateDown(int subtree); }; #include "ArrayMaxHeap.cpp" #endif /* ARRAYMAXHEAP_H */
Please help me design a Airworthy class in C++.
Develop a high-quality, object-oriented C++ program that performs a simulation using a heap implementation of a priority queue.
A simulation creates a model of a real-world situation, allowing us to introduce a variety of conditions and observe their effects. For instance, a flight simulator challenges a pilot to respond to varying conditions and measures how well the pilot responds. Simulation is frequently used to measure current business practices, such as the number of checkout lines in a grocery store or the number of tellers in a bank, so that management can determine the fewest number of employees required to meet customer needs.
Airlines have been experimenting with different boarding procedures to shorten the entire boarding time, keep the flights on-time, reduce aisle congestion, and make the experience more pleasant for passengers and crew. A late-departing flight can cause a domino effect:
- the departure gate is tied up and cannot be used by other landing or departing flights,
- passengers on board the late flight may miss connecting flights and require rebooking and possibly overnight arrangements (meals and lodging)
- passengers complain about being late, and/or about having to rearrange their plans.
Thus, late flights have a huge operational impact.
For this assignment, we will simulate boarding procedures for Airworthy Airlines. The Airline's current procedure is as follows:
- pre-board in the following order:
- families with young children or people who need help (e.g., wheelchair)
- first class and/or business class passengers
- elite passengers (frequent fliers) and those passengers seated in exit rows
- conduct general boarding in reverse, from the back of the plane to the front in the following order:
- rows 23-26
- rows 17-22
- rows 11-16
- rows 5-10
Airworthy is considering revising their boarding procedure such that general boarding is done randomly, meaning the first passenger in line for general boarding is the first passenger to board (i.e., general boarding passengers all have the same priority). Airworthy suspects this random general boarding method will improve the flow of passengers, getting them on board and seated more quickly. It is also less labor-intensive for Airworthy's customer service agents because it significantly reduces the number of boarding announcements required and eliminates confrontations with customers trying to board "out of turn." Note that the revision is to general boarding only. The pre-boarding procedures will not be changed.
Develop an Airworthy class that contains the following data members:
- exactly one priority queue where the priority is set using Airworthy's previous boarding procedure
- exactly one priority queue where the priority is set using Airworthy's new random boarding procedure
- the amount of time, in seconds, required to board a plane using Airworthy's previous boarding procedure
- the amount of time, in seconds, required to board a plane using Airworthy's new random boarding procedure
You may use additional attributes, as needed. The Airworthy class must include methods to support the following functions of the class:
- a constructor
- read the data from the input file
- load the two priority queues
- run the simulation
When running the simulation, assume that:
- A passenger who is not blocked by another passenger requires one second to board.
- A passenger is blocked when the previous passenger is sitting in the same row or a row closer to the front of the plane. A blocked passenger requires 25 seconds to board
HeapException.cpp:
#include "HeapException.h"
HeapException::HeapException(const string& msg)
: logic_error("Heap Exception: " + msg) {
}
HeapException.h:
#ifndef HEAPEXCEPTION_H
#define HEAPEXCEPTION_H
#include <stdexcept>
#include <string>
using namespace std;
class HeapException : public logic_error {
public:
HeapException(const string& msg = "");
};
#endif /* HEAPEXCEPTION_H */
HeapADT:
#ifndef HEAPADT_H
#define HEAPADT_H
template <class T>
class HeapADT {
public:
virtual bool isEmpty() const = 0;
virtual int getItemCount() const = 0;
virtual T peek() const = 0;
virtual bool add(const T& newItem) = 0;
virtual bool remove() = 0;
virtual void clear() = 0;
};
#endif /* HEAPADT_H */
ArrayMaxHeap.h:
#ifndef ARRAYMAXHEAP_H
#define ARRAYMAXHEAP_H
#include "HeapADT.h"
template <class T>
class ArrayMaxHeap : public HeapADT<T> {
public:
ArrayMaxHeap();
virtual ~ArrayMaxHeap();
bool isEmpty() const;
int getItemCount() const;
T peek() const;
bool add(const T& newItem);
bool remove();
void clear();
private:
static const int ROOT_INDEX = 0;
static const int CAPACITY = 5000;
int itemCount;
T* items;
int getLeftChild(int currNode) const;
int getRightChild(int currNode) const;
int getParent(int currNode) const;
bool isLeaf(int currNode) const;
void percolateDown(int subtree);
};
#include "ArrayMaxHeap.cpp"
#endif /* ARRAYMAXHEAP_H */
![semplate celass T>
a boel Arraylanlleap::remeve() (
it (ialapty())
shrov Heaptacepeden ("Teying to renove from an pey heap. ")
else (
items ROOT_INDExj. itens (itesCouns - 11
percelateDovn(R0oT_INEC),
• Teat if heap is empty
* fretuen tsue if empty
Bemplate celas D
bool Arraylanlleap dalapty() conss (
zeturn (iseCount 0.
/secursive nethoda
semplate cclass T
veid Araytlanlleap>spereolateDown (ins ubecee) (
it (Iiateat (ubtcee)) (
//ialeaf() is the base ease
ins lefchild gettefch4(auberee)
int aChild lefschilds
im rightchild getkightch1d(muberee)
it (zightChild < itemCount) (
//eight child exists
st (itens (elghschi14) > isens(lefechild))
I/aChild has the inder of the largeat value of 2 children
if (ites (aubteee) <ite (aChild])
sont « "unpping « itema (onbtree)
«* and « itens (naC4 « endl
vap(iten (eubtcee), ban aCld))
percelateDovn (machd)
/peivate methoda
template celass T
O inn Arrayanlleap getletia4(in curallode) const (
revurn eurrlode + 1r
semplate celan T
O Lnn ArrayaalleapsgetightChld(int eurellede) conan
Eun curlede 2+ 2
semplate celans T
E ins Arrayanleap getPacent (ins eullodel con
en (eurllode - 1) / 2
seplane cela D
boel Araytanleap>IIslent (in eurelede) ceert (
sEum getietcd leurelede) eC
D O U- O-](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fce52493c-af35-4b0a-9ee4-a1a491078c62%2F6e579c15-43bb-4d62-99ea-a10af2d0608d%2F7lukdxk_processed.jpeg&w=3840&q=75)
![finclude "Arraylanleap.h"
finelude "HeapEscepsien.h"
semplate celass D
Arraytlanlleap>:Arraslallenp() I lteaCount (0) (
itens nev T(CAPACITY)
template celass D
Arraytlantlleapt>1-Arraylallenp ()
delese () itensI
/interface nethods
* Add an iten te the max beap
* tpatan neuiten iten to be added
* tretuen true if successful
26
27
semplate cela D
boel Arraytlanlleap::add(eonas Ti neviten) (
if (isenCouns CAPACITI)
shrov Heaptaception("Tsying te add se a full heap. ")
29
30
31
32
/add te bottom/eight of taee
itens fisencoune)nevite
ins nevllode LbenCoun
33
boel inPlace false
//peneelate new node up te preper place
while (nevlede > 0 lialace) (
in parent garens (nevilode)
it (isen (nevilede) < ite (parens))
inlace seves
else {
Seut < "Bupping itema (nedlede]
«and « items (pavent) < endi:
vap (ite (nevlede), 1bem (parenn)),
evlode parent
1//new node ia place
LeCoun
Remores all itms from the heap
semplate celass D
veid Arraylalleap selear ()I
iteCouns Or
Retuna the munber of itms in the heap
*Iretun the aunber of iems ia the beap
semplate celass T
Lan AaylanlleapDpetitecent ) conas
eun enCou](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fce52493c-af35-4b0a-9ee4-a1a491078c62%2F6e579c15-43bb-4d62-99ea-a10af2d0608d%2Fg9wf02f_processed.jpeg&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

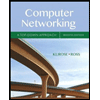
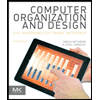
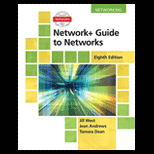
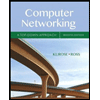
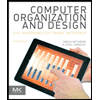
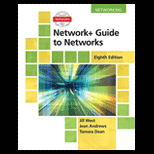
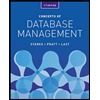
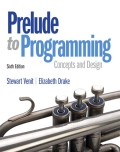
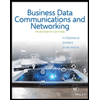