Please help.The html and css code + instructions are in the image. The server code is below Thank you var express = require('express'); //we must have express installed! var app = express(); var idCounter = 0; // we will assign every round of game play a unique ID var gameInfo = {}; // an empty JS object, later it's going to store the code for each end-user var port = 3000; app.post('/post', (req, res) => { //print info to console console.log("New express client"); console.log("Query received: "); console.log(JSON.parse(req.query['data'])); //populate a header response res.header("Access-Control-Allow-Origin", "*"); var queryInfo = JSON.parse(req.query['data']); if (queryInfo['action'] == 'generateWord') { //are we asked to generate a word? idCounter++; var nameID = queryInfo['name'] + idCounter; generateWord(nameID); var jsontext = JSON.stringify({ 'action': 'generateWord', 'nameID': nameID, 'len': gameInfo[nameID][0].length, 'msg': 'New word generated!!!' }); res.send(jsontext); } else if (queryInfo['action'] == "evaluate") { //are we asked to evaluate a guess? var [correct, counter, attemptWord] = makeGuess(gameInfo[queryInfo['name']], queryInfo['letterGuess'], queryInfo['guessWord']); gameInfo[queryInfo['name']][1] = counter /* the response will have 6 parts: request action, whether won or not, number of exact matches, number of colors that are correct, number of wrong colors, and the answer if the game ended */ var jsontext = JSON.stringify({ 'action': 'evaluate', 'correct': correct, 'attemptWord': attemptWord, 'num_errors': counter }); console.log(jsontext); res.send(jsontext); } else { res.send(JSON.stringify({ 'msg': 'error!!!' })); } }).listen(3000); console.log("Server is running!"); /* * Evauate the client's guess * The parameters to this function are: * input, letterGuess, attemptWord * input is the server's stored info for this client * - input[0] contains the client's secret WORD * - input[1] contains the client's ERROR COUNT * letterGuess is the client attempted guess (it is a letter) * attemptWord records the client's current progress on the word (it is an array) * * The function returns an array [correct, num_errors, attemptWord] * correct is a BOOLEAN that indicates if the user's guess is correct * num_errors is count of the user's ERRORS thus far * attemptWord is an updated record of the client's current progress on the word */ function makeGuess(input, letterGuess, attemptWord){ //you will write this code! //input[0] contains this user's secret word //input[1] contains this user's error count //letterGuess is the letter that the user has guessed //attemptWord is a log of letters the user has guessed correctly. //STEP 1. Cycle thru the user's secret word //Assess if and where letterGuess appears in this word //If it appears in the word, update the ltters within //attemptWord accordingly //STEP 2. Calculate the user's error count, //if it is the case that letterGuess does not exist in //input[0] //RETURN an array containing a BOOLEAN, //a NUMBER, and attemptWord (which is an array) //Your return statement will look something //like this: //return [correct, num_errors, attemptWord] } function generateWord(clientName) { var possibleWords = [ ["R", "A", "D", "I", "O"], ["T","E","A","M","W","O","R","K"], ["W","E","B","D","E","S","I","G","N"], ["E","D","U","C","A","T","I","O","N"], ["C","H","O","C","O","L","A","T","E"], ["U","N","I","V","E","R","S","I","T","Y"] ] //generate word var index = //... Finish this line!! Select an element from possibleWords at random. gameInfo[clientName] = [possibleWords[index], 0]; //store the secret word and error count for this user! }
Please help.The html and css code + instructions are in the image. The server code is below
Thank you
var express = require('express'); //we must have express installed!
var app = express();
var idCounter = 0; // we will assign every round of game play a unique ID
var gameInfo = {}; // an empty JS object, later it's going to store the code for each end-user
var port = 3000;
app.post('/post', (req, res) => {
//print info to console
console.log("New express client");
console.log("Query received: ");
console.log(JSON.parse(req.query['data']));
//populate a header response
res.header("Access-Control-Allow-Origin", "*");
var queryInfo = JSON.parse(req.query['data']);
if (queryInfo['action'] == 'generateWord') { //are we asked to generate a word?
idCounter++;
var nameID = queryInfo['name'] + idCounter;
generateWord(nameID);
var jsontext = JSON.stringify({
'action': 'generateWord',
'nameID': nameID,
'len': gameInfo[nameID][0].length,
'msg': 'New word generated!!!'
});
res.send(jsontext);
} else if (queryInfo['action'] == "evaluate") { //are we asked to evaluate a guess?
var [correct, counter, attemptWord] = makeGuess(gameInfo[queryInfo['name']], queryInfo['letterGuess'], queryInfo['guessWord']);
gameInfo[queryInfo['name']][1] = counter
/* the response will have 6 parts: request action, whether won or not, number of exact matches,
number of colors that are correct, number of wrong colors, and the answer if the game ended */
var jsontext = JSON.stringify({
'action': 'evaluate',
'correct': correct,
'attemptWord': attemptWord,
'num_errors': counter
});
console.log(jsontext);
res.send(jsontext);
} else {
res.send(JSON.stringify({ 'msg': 'error!!!' }));
}
}).listen(3000);
console.log("Server is running!");
/*
* Evauate the client's guess
* The parameters to this function are:
* input, letterGuess, attemptWord
* input is the server's stored info for this client
* - input[0] contains the client's secret WORD
* - input[1] contains the client's ERROR COUNT
* letterGuess is the client attempted guess (it is a letter)
* attemptWord records the client's current progress on the word (it is an array)
*
* The function returns an array [correct, num_errors, attemptWord]
* correct is a BOOLEAN that indicates if the user's guess is correct
* num_errors is count of the user's ERRORS thus far
* attemptWord is an updated record of the client's current progress on the word
*/
function makeGuess(input, letterGuess, attemptWord){
//you will write this code!
//input[0] contains this user's secret word
//input[1] contains this user's error count
//letterGuess is the letter that the user has guessed
//attemptWord is a log of letters the user has guessed correctly.
//STEP 1. Cycle thru the user's secret word
//Assess if and where letterGuess appears in this word
//If it appears in the word, update the ltters within
//attemptWord accordingly
//STEP 2. Calculate the user's error count,
//if it is the case that letterGuess does not exist in
//input[0]
//RETURN an array containing a BOOLEAN,
//a NUMBER, and attemptWord (which is an array)
//Your return statement will look something
//like this:
//return [correct, num_errors, attemptWord]
}
function generateWord(clientName) {
var possibleWords = [
["R", "A", "D", "I", "O"],
["T","E","A","M","W","O","R","K"],
["W","E","B","D","E","S","I","G","N"],
["E","D","U","C","A","T","I","O","N"],
["C","H","O","C","O","L","A","T","E"],
["U","N","I","V","E","R","S","I","T","Y"]
]
//generate word
var index = //... Finish this line!! Select an element from possibleWords at random.
gameInfo[clientName] = [possibleWords[index], 0]; //store the secret word and error count for this user!
}
![H. Task 2 Server side
In this task, you will complete the server-side JS file. What do you need to do in this task is the following
steps:
i) You will add one line to the function generateWord. This is indicated in the comments. The line
should select an index at random from the array of possibilities. Make sure you generate a
number in the appropriate interval.
ii) You will then write the function makeGuess. This function is used to evaluate a user's guess of a
letter, on the server side. There are three parameters to this function: input, letterGuess, and
attemptWord. The input parameter is the server's information for this client. It is an array;
input[0] contains the client's secret WORD and input[1] contains the client's current ERROR
COUNT. The input parameter letterGuess is the client attempted guess (and is a letter). Finally,
the parameter attemptWord is a record the client's current progress on the given word. It is an
array that contains all the letters the user has correctly guessed and "_" in place of the
characters that the user has yet to guess. The function will return an array of three values as
follows: [correct, num_errors, attemptWord]. The value correct should be a BOOLEAN that
indicates if the user's guess is correct (i.e. it exists in the target word), num_errors should be an
updated count of the user's ERRORS and attemptWord should be an updated record of the client's
progress. More information can be found in the comments in the code.
iii) Finally, follow the instructions at the end of this manual to run the server locally on your machine
and play the game on your device any time.
Note: since you are running the server on your local computer, you will be able see the output by
the server code, examining how the messages are transferred between the client and server. And
the server responds back with information as well.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd9d632c5-d4ea-478f-a512-43d3c8c76b91%2F849e5f84-d382-49d0-8e5e-947f012d540a%2Fbs3bvwc_processed.png&w=3840&q=75)


Step by step
Solved in 3 steps with 1 images

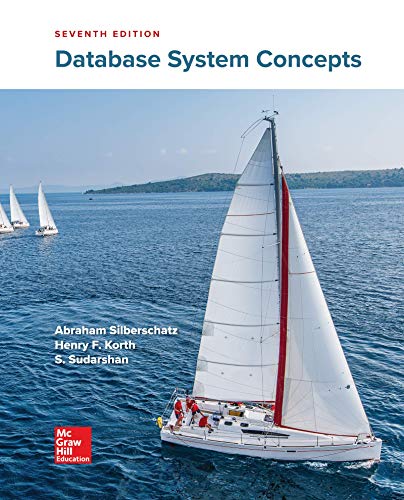
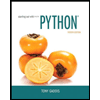
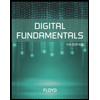
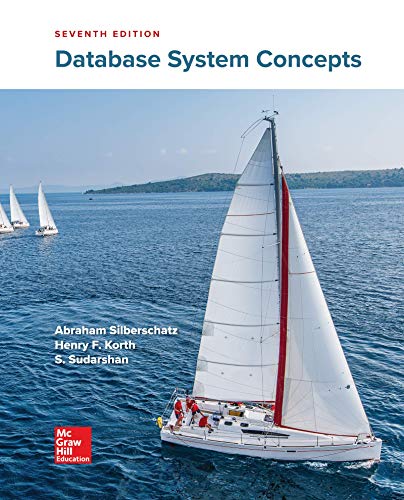
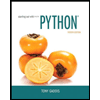
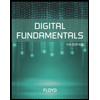
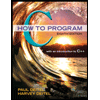
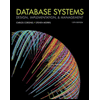
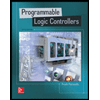