Please modify the C++ data structure program below to detect and display multiple candidates with the same number of votes: multiple winners, multiple candidates with the second highest number of votes, multiple candidates with the third highest number of votes, and so on. Suppose the candidates are A, B, C, D, E, F and G and their votes are: A 100 B 200 C 200 D 400 E 100 F 400 G 300 H 300 Then your program should display, A Fourth Place B Third Place C Third Place D First Place E Fourth Place F First Place G Second Place H Second Place with the amount of votes they received, for example: A Fourth Place with 100 votes, B Third Place with 200 votes, C Third Place with 200 and etc. Text file names and program below. Thank you. votes.txt A = Johnson B = Miller C = Robinson D = Duffy E = Asthon F = Sampson G = Adams H = Williams ________________________________________________________________________________ #include #include #include #include #include #include #include #include using namespace std; const string filename = "votes.txt"; const int maxCandidates = 8; struct Candidate { string name = ""; size_t votes = 0; double percent = 0.0; }; int main() { ifstream inps(filename); assert(inps); array candidates; size_t nCandidates = 0; while (inps.good() && nCandidates < maxCandidates) { string name; inps >> name; size_t votes; inps >> votes; candidates[nCandidates].name = name; candidates[nCandidates].votes = votes; nCandidates++; } if (nCandidates > 0) { size_t namefieldwidth = 12; size_t maxvotes = 0; size_t maxidx = 0; size_t totalvotes = 0; for (size_t idx = 0; idx < nCandidates; idx++) { totalvotes += candidates[idx].votes; if (candidates[idx].votes > maxvotes) { maxvotes = candidates[idx].votes; maxidx = idx; } } size_t namefieldwith = 16; size_t votesfieldwidth = 16; size_t percentfieldwidth = 16; if (totalvotes > 0) { for (size_t idx = 0; idx < nCandidates; idx++) candidates[idx].percent = 100.0 * candidates[idx].votes / static_cast(totalvotes); } cout << fixed << setprecision(2); cout << left << setw(namefieldwidth) << "Candidate" << ' ' << right << setw(votesfieldwidth) << "Votes Received" << ' ' << right << setw(percentfieldwidth) << setfill(' ') << "% of Total Votes " << endl << endl; for (size_t idx = 0; idx < nCandidates; ++idx) cout << left << setw(namefieldwidth) << candidates[idx].name << ' ' << right << setw(votesfieldwidth) << candidates[idx].votes << ' ' << right << setw(percentfieldwidth) << setfill(' ') << candidates[idx].percent << endl; size_t totalvotesfieldwidth = namefieldwidth + votesfieldwidth + 1; cout << setw(totalvotesfieldwidth) << totalvotes << endl << endl; cout << "The winner of the Election is " << candidates[maxidx].name << endl; } return 0; } This is my second time with this question. This statement below was added to the code on the first attemp and the image uploaded was the output. That is incorrect. Could you please modify the code according to the instructions please. Thank you /** Implementation/Code change to display multiple winners This way more than one candidate with same number of maximum votes can be displayed **/ //Loops through every candidate for (size_t idx = 0; idx < nCandidates; idx++) { //If the candidate has max votes if (candidates[idx].votes == maxvotes) { //Display the candidate name cout << candidates[idx].name << endl; } } } return 0; }
Please modify the C++ data structure program below to detect and display multiple candidates with the same number of votes: multiple winners, multiple candidates with the second highest number of votes, multiple candidates with the third highest number of votes, and so on.
Suppose the candidates are A, B, C, D, E, F and G and their votes are:
A 100
B 200
C 200
D 400
E 100
F 400
G 300
H 300
Then your program should display,
A Fourth Place
B Third Place
C Third Place
D First Place
E Fourth Place
F First Place
G Second Place
H Second Place
with the amount of votes they received, for example: A Fourth Place with 100 votes, B Third Place with 200 votes, C Third Place with 200 and etc. Text file names and program below. Thank you.
votes.txt |
A = Johnson |
B = Miller |
C = Robinson |
D = Duffy |
E = Asthon |
F = Sampson |
G = Adams |
H = Williams |
________________________________________________________________________________
#include <array>
#include <cassert>
#include <cmath>
#include <fstream>
#include <iomanip>
#include <iostream>
#include <string>
#include <vector>
using namespace std;
const string filename = "votes.txt";
const int maxCandidates = 8;
struct Candidate
{
string name = "";
size_t votes = 0;
double percent = 0.0;
};
int main()
{
ifstream inps(filename);
assert(inps);
array<Candidate, maxCandidates> candidates;
size_t nCandidates = 0;
while (inps.good() && nCandidates < maxCandidates)
{
string name;
inps >> name;
size_t votes;
inps >> votes;
candidates[nCandidates].name = name;
candidates[nCandidates].votes = votes;
nCandidates++;
}
if (nCandidates > 0)
{
size_t namefieldwidth = 12;
size_t maxvotes = 0;
size_t maxidx = 0;
size_t totalvotes = 0;
for (size_t idx = 0; idx < nCandidates; idx++)
{
totalvotes += candidates[idx].votes;
if (candidates[idx].votes > maxvotes)
{
maxvotes = candidates[idx].votes;
maxidx = idx;
}
}
size_t namefieldwith = 16;
size_t votesfieldwidth = 16;
size_t percentfieldwidth = 16;
if (totalvotes > 0)
{
for (size_t idx = 0; idx < nCandidates; idx++)
candidates[idx].percent = 100.0 * candidates[idx].votes / static_cast<double>(totalvotes);
}
cout << fixed << setprecision(2);
cout << left << setw(namefieldwidth) << "Candidate" << ' ' << right << setw(votesfieldwidth) << "Votes Received" << ' ' << right << setw(percentfieldwidth) << setfill(' ') << "% of Total Votes " << endl << endl;
for (size_t idx = 0; idx < nCandidates; ++idx)
cout << left << setw(namefieldwidth) << candidates[idx].name << ' ' << right << setw(votesfieldwidth) << candidates[idx].votes << ' ' << right << setw(percentfieldwidth) << setfill(' ') << candidates[idx].percent << endl;
size_t totalvotesfieldwidth = namefieldwidth + votesfieldwidth + 1;
cout << setw(totalvotesfieldwidth) << totalvotes << endl << endl;
cout << "The winner of the Election is " << candidates[maxidx].name << endl;
}
return 0;
}
/**
Implementation/Code change to display multiple winners
This way more than one candidate with same number of maximum votes can be displayed
**/
//Loops through every candidate
for (size_t idx = 0; idx < nCandidates; idx++)
{
//If the candidate has max votes
if (candidates[idx].votes == maxvotes)
{
//Display the candidate name
cout << candidates[idx].name << endl;
}
}
}
return 0;
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

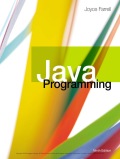
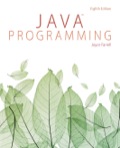
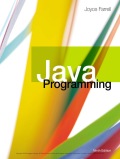
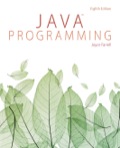