Please Solve this C++ question as per the instructions. Please don't copy other solutions. Thank you so much for your help! Q. Write a C++ program that will allocates an array of integers of size 10 (Use standard C++11 for the array declaration). Next it will prompt the user to enter 10 distinct integer values to be stored in the array that you just created, in the order they were read. Then, the program allocates an auxiliary array with the size of original array (10) and using the following two functions copies all the even numbers of original array in the auxiliary array followed by odd numbers. To do this you need to implement two functions as follows: 1- Separate Even(int[], int[]): This function accepts the original array and auxiliary array as the input and copies all the even numbers of the original array in the auxiliary array. Then it returns the number of even elements copied form original array to the auxiliary array. 2- SeparateOdd(int[], int[], int): This function accepts the original array, auxiliary array, and number of even numbers in the original array as the inputs (this function has 3 input arguments), and copies all the odd numbers of the original array after the even numbers (that are already copied) in the auxiliary array. This function does not return any value. Your program should display the auxiliary array after calling the above two functions. All output must be formatted exactly as displayed in the two samples below. Please enter 10 integers: 14 265 48 11 21 54 88 90 713 7 The content of auxiliary array is: 14 48 54 88 90 265 11 21 713 7 Even Odd Please enter 10 integers: 13 265 48 11 21 54 88 90 713 7 The content of auxiliary array is: 48 54 88 90 13 265 11 21 713 7 Even Odd
Please Solve this C++ question as per the instructions. Please don't copy other solutions. Thank you so much for your help! Q. Write a C++ program that will allocates an array of integers of size 10 (Use standard C++11 for the array declaration). Next it will prompt the user to enter 10 distinct integer values to be stored in the array that you just created, in the order they were read. Then, the program allocates an auxiliary array with the size of original array (10) and using the following two functions copies all the even numbers of original array in the auxiliary array followed by odd numbers. To do this you need to implement two functions as follows: 1- Separate Even(int[], int[]): This function accepts the original array and auxiliary array as the input and copies all the even numbers of the original array in the auxiliary array. Then it returns the number of even elements copied form original array to the auxiliary array. 2- SeparateOdd(int[], int[], int): This function accepts the original array, auxiliary array, and number of even numbers in the original array as the inputs (this function has 3 input arguments), and copies all the odd numbers of the original array after the even numbers (that are already copied) in the auxiliary array. This function does not return any value. Your program should display the auxiliary array after calling the above two functions. All output must be formatted exactly as displayed in the two samples below. Please enter 10 integers: 14 265 48 11 21 54 88 90 713 7 The content of auxiliary array is: 14 48 54 88 90 265 11 21 713 7 Even Odd Please enter 10 integers: 13 265 48 11 21 54 88 90 713 7 The content of auxiliary array is: 48 54 88 90 13 265 11 21 713 7 Even Odd
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter7: Arrays
Section: Chapter Questions
Problem 6PP: (Numerical) a. Define an array with a maximum of 20 integer values, and fill the array with numbers...
Related questions
Question
Please Solve this C++ question as per the instructions. Please don't copy other solutions.
Thank you so much for your help!
Q. Write a C++ program that will allocates an array of integers of size 10 (Use standard C++11 for the array declaration). Next it will prompt the user to enter 10 distinct integer values to be stored in the array that you just created, in the order they were read. Then, the program allocates an auxiliary array with the size of original array (10) and using the following two functions copies all the even numbers of original array in the auxiliary array followed by odd numbers. To do this you need to implement two functions as follows:
1- Separate Even(int[], int[]): This function accepts the original array and auxiliary array as the input and copies all the even numbers of the original array in the auxiliary array. Then it returns the number of even elements copied form original array to the auxiliary array.
2- SeparateOdd(int[], int[], int): This function accepts the original array, auxiliary array, and number of even numbers in the original array as the inputs (this function has 3 input arguments), and copies all the odd numbers of the original array after the even numbers (that are already copied) in the auxiliary array. This function does not return any value.
Your program should display the auxiliary array after calling the above two functions.
All output must be formatted exactly as displayed in the two samples below.
Please enter 10 integers: 14 265 48 11 21 54 88 90 713 7
The content of auxiliary array is:
14 48 54 88 90 265 11 21 713 7
Even Odd
Please enter 10 integers: 13 265 48 11 21 54 88 90 713 7
The content of auxiliary array is:
48 54 88 90 13 265 11 21 713 7
Even Odd

Transcribed Image Text:Your program should display the auxiliary array after calling the above two functions.
All output must be formatted exactly as displayed in the two samples below.
Please enter 10 integers: 14 265 48 11 21 54 88 90 713 7
The content of auxiliary array is:
14 48 54 88 90 265 11 21 713 7
Evens
Odds
Please enter 10 integers: 13 265 48 11 21 54 88 98 713 7J
The content of auxiliary array is:
Evens
Odds
48 54 88 90 13 265 11 21 713 7
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
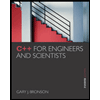
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
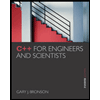
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr