print height, the largest key, and average of the keys. import java.util.*; public class BinarySearchTree1 { public Node root; public BinarySearchTree1() { root = null; } public Node getroot() { return root; } public Node Search(int KEY) { Node x = root; while (x != null && x.item != KEY) { if (KEY < x.item) { x = x.Left; } else { x = x.Right; } } return x; // x.item == key or x == null } public Node SearchRec(Node root, int key) { if (root == null) return null; if ( root.item == key ) return root; if (root.item > key) return SearchRec(root.Left, key); else return SearchRec(root.Right, key); } public void Insert(int newItem) { Node parent = null; Node newNode = new Node(newItem); Node current = root; while (current != null) { parent = current; if (newNode.item < current.item) { current = current.Left; } else { current = current.Right; } } if (root == null) { root = newNode; } else { if (newNode.item < parent.item) { parent.Left = newNode; } else { parent.Right = newNode; } } } public boolean Delete(int key) { Node parent = null; Node curr = root; while (curr != null && curr.item != key) { parent = curr; if (key < curr.item) { curr = curr.Left; } else { curr = curr.Right; } } if (curr == null) { return false; } if (curr.Left == null && curr.Right == null) { if (curr != root) { if (parent.Left == curr) { parent.Left = null; } else { parent.Right = null; } } else { root = null; } } else if (curr.Left != null && curr.Right != null) { Node successor = getSuccessor(curr.Right); int val = successor.item; Delete(successor.item); curr.item = val; } else { Node child = (curr.Left != null)? curr.Left: curr.Right; if (curr != root) { if (curr == parent.Left) { parent.Left = child; } else { parent.Right = child; } } else { root = child; } } return true; } public Node getSuccessor(Node curr) { while (curr.Left != null) { curr = curr.Left; } return curr; } public void InOrder(Node theRoot) { if (!(theRoot == null)) { InOrder(theRoot.Left); theRoot.DisplayNode(); InOrder(theRoot.Right); } } }
print height, the largest key, and average of the keys.
import java.util.*;
public class BinarySearchTree1
{
public Node root;
public BinarySearchTree1()
{
root = null;
}
public Node getroot()
{
return root;
}
public Node Search(int KEY)
{
Node x = root;
while (x != null && x.item != KEY)
{
if (KEY < x.item)
{
x = x.Left;
}
else
{
x = x.Right;
}
}
return x; // x.item == key or x == null
}
public Node SearchRec(Node root, int key)
{
if (root == null)
return null;
if ( root.item == key )
return root;
if (root.item > key)
return SearchRec(root.Left, key);
else
return SearchRec(root.Right, key);
}
public void Insert(int newItem)
{
Node parent = null;
Node newNode = new Node(newItem);
Node current = root;
while (current != null)
{
parent = current;
if (newNode.item < current.item)
{
current = current.Left;
}
else
{
current = current.Right;
}
}
if (root == null)
{
root = newNode;
}
else
{
if (newNode.item < parent.item)
{
parent.Left = newNode;
}
else
{
parent.Right = newNode;
}
}
}
public boolean Delete(int key)
{
Node parent = null;
Node curr = root;
while (curr != null && curr.item != key)
{
parent = curr;
if (key < curr.item)
{
curr = curr.Left;
}
else
{
curr = curr.Right;
}
}
if (curr == null) {
return false;
}
if (curr.Left == null && curr.Right == null)
{
if (curr != root)
{
if (parent.Left == curr) {
parent.Left = null;
}
else {
parent.Right = null;
}
}
else {
root = null;
}
}
else if (curr.Left != null && curr.Right != null)
{
Node successor = getSuccessor(curr.Right);
int val = successor.item;
Delete(successor.item);
curr.item = val;
}
else {
Node child = (curr.Left != null)? curr.Left: curr.Right;
if (curr != root)
{
if (curr == parent.Left) {
parent.Left = child;
}
else {
parent.Right = child;
}
}
else {
root = child;
}
}
return true;
}
public Node getSuccessor(Node curr)
{
while (curr.Left != null) {
curr = curr.Left;
}
return curr;
}
public void InOrder(Node theRoot)
{
if (!(theRoot == null))
{
InOrder(theRoot.Left);
theRoot.DisplayNode();
InOrder(theRoot.Right);
}
}
}

Step by step
Solved in 2 steps

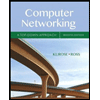
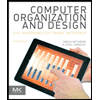
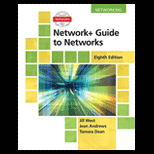
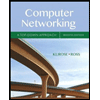
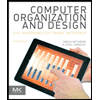
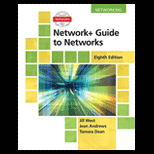
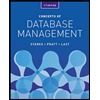
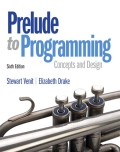
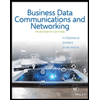