Problem #1 - Initializing and summing an array using for loops Write a program which creates a one dimensional, array of int type with 10 elements. Name the array "data[]". Initialize the array using a for loop such that each element is its index number plus 10. For example, data[0] will be a value of 10, data[1] will be 11, etc. Print out all the elements of the array using two columns. The first column is the index and the second column is the value of the element. After printing out the index and values, print out the sum of all the elements of the array. Use a for loop to sum all the elements. Make the output look like this screen shot. Name the file "array_sum.c" Index Value 10 11 12 13 14 15 16 17 18 19 Sum 145
Problem #1 - Initializing and summing an array using for loops Write a program which creates a one dimensional, array of int type with 10 elements. Name the array "data[]". Initialize the array using a for loop such that each element is its index number plus 10. For example, data[0] will be a value of 10, data[1] will be 11, etc. Print out all the elements of the array using two columns. The first column is the index and the second column is the value of the element. After printing out the index and values, print out the sum of all the elements of the array. Use a for loop to sum all the elements. Make the output look like this screen shot. Name the file "array_sum.c" Index Value 10 11 12 13 14 15 16 17 18 19 Sum 145
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter7: Arrays
Section7.1: One-dimensional Arrays
Problem 10E: (Electrical eng.) Write a program that specifies three one-dimensional arrays named current,...
Related questions
Question
100%
- Needs to be written in C
![Problem #1 - Initializing and summing an array using for loops
Write a program which creates a one dimensional, array of int type with 10 elements. Name the array "data[]". Initialize the array using a for loop such that each element is its index number
plus 10. For example, data[0] will be a value of 10, data[1] will be 11, etc. Print out all the elements of the array using two columns. The first column is the index and the second column is
the value of the element. After printing out the index and values, print out the sum of all the elements of the array. Use a for loop to sum all the elements. Make the output look like this
screen shot. Name the file "array_sum.c"
Index
Value
10
11
12
3
13
14
15
16
17
18
9
19
145
Sum
Process returned 0
Press any key to co
Problem #2 - Initialize an array and compute the average
Write a program which creates a one dimensional array of float type with 5 elements. Name the array "data[]". Initialize the array when it is created with these values: 2.5, 3.33, 4.2, 8.0, 5.1.
Compute the average of all the elements in the array using a for loop to sum the array. Print out all the elements of the array using two columns. The first column is the index and the
second column is the value of the element. After printing out the index and values, print out the average of all the elements of the array. Make the output look like this screen shot. Name
the file "array_average.c"
Index
Value
2.500000
1
3.330000
4.200000
12
3
4
Avg
8.000000
5.100000
4.626000
Process returned 0
Press any key to co](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb488d958-b9f8-4820-a61d-1a1d881a5d5d%2F96f9d993-2d4c-4a20-a8a9-586c58ecbbe4%2Fzku057i_processed.png&w=3840&q=75)
Transcribed Image Text:Problem #1 - Initializing and summing an array using for loops
Write a program which creates a one dimensional, array of int type with 10 elements. Name the array "data[]". Initialize the array using a for loop such that each element is its index number
plus 10. For example, data[0] will be a value of 10, data[1] will be 11, etc. Print out all the elements of the array using two columns. The first column is the index and the second column is
the value of the element. After printing out the index and values, print out the sum of all the elements of the array. Use a for loop to sum all the elements. Make the output look like this
screen shot. Name the file "array_sum.c"
Index
Value
10
11
12
3
13
14
15
16
17
18
9
19
145
Sum
Process returned 0
Press any key to co
Problem #2 - Initialize an array and compute the average
Write a program which creates a one dimensional array of float type with 5 elements. Name the array "data[]". Initialize the array when it is created with these values: 2.5, 3.33, 4.2, 8.0, 5.1.
Compute the average of all the elements in the array using a for loop to sum the array. Print out all the elements of the array using two columns. The first column is the index and the
second column is the value of the element. After printing out the index and values, print out the average of all the elements of the array. Make the output look like this screen shot. Name
the file "array_average.c"
Index
Value
2.500000
1
3.330000
4.200000
12
3
4
Avg
8.000000
5.100000
4.626000
Process returned 0
Press any key to co
![Problem #3 - Search a string
Write a program which counts the number of a particular character found in a string. For example, in the string "double down" there are two 'd' characters. Use a loop to go through the
string from beginning to end and compare each character in the string with the character to find. If the character is found, increment a count. Print out the string and the number found. See
the screen shot below. The string is "Find the letter i in this string." The character to find and count is 'i'. Name the file "string_search.c".
The typical way to go through a string from beginning to end is using a while loop. The test in the loop checks if the current element in the loop is a NULL character. If a NULL is found, stop
looping since the end of the string has been found. For example,
i = 0;
while(str[i] != '\0') {
}
Of course, i must be incremented to go through the string each time through the loop.
String: Find the letter i in this string.
Count: 5
Process returned 0 (0x0)
Press any key to continue.
execution time : 0.081 s](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb488d958-b9f8-4820-a61d-1a1d881a5d5d%2F96f9d993-2d4c-4a20-a8a9-586c58ecbbe4%2Fgipv93j_processed.png&w=3840&q=75)
Transcribed Image Text:Problem #3 - Search a string
Write a program which counts the number of a particular character found in a string. For example, in the string "double down" there are two 'd' characters. Use a loop to go through the
string from beginning to end and compare each character in the string with the character to find. If the character is found, increment a count. Print out the string and the number found. See
the screen shot below. The string is "Find the letter i in this string." The character to find and count is 'i'. Name the file "string_search.c".
The typical way to go through a string from beginning to end is using a while loop. The test in the loop checks if the current element in the loop is a NULL character. If a NULL is found, stop
looping since the end of the string has been found. For example,
i = 0;
while(str[i] != '\0') {
}
Of course, i must be incremented to go through the string each time through the loop.
String: Find the letter i in this string.
Count: 5
Process returned 0 (0x0)
Press any key to continue.
execution time : 0.081 s
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
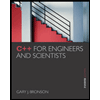
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
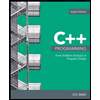
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
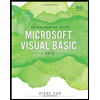
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
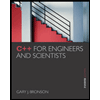
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
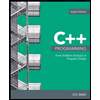
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
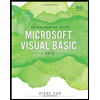
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage