Problem John is developing a python program that comes up with a number and allows the user to guess that number. The game allows the user to input their guess, which afterward, the game will tell the user one of three things: (1) the guessed number is too high, (2) the guessed number is too low, or (3) the guessed number is correct. If #3 occurs, then the game ends. Learning Objectives Practice conditionally executing code. Practice reading portions of code already written for you. Understand the flow of a program. Understand how and when the program will be executed. Template from random import randint def guess(user_guess, correct_num): # If the user guesses wrong, tell them if their # guess is too low or too high, then return False. # Fix the XXXXX's in the template below to get it to work correctly if XXXXX: # TODO: Print the output and return False elif XXXXX: # TODO: Print the output and return False else: # TODO: If the user guesses correctly, congratulate them # and return True if __name__ == "__main__": num = randint(-50, 50) while True: user_guess = int(input("Enter your guess: ")) if guess(user_guess, num) == True: break Assignment Write the guess(user_num, correct_num) code. In the guess() code, you need a series of if statements to test three possibilities: (1) the player guessed too high, (2) the player guessed too low, or (3) the player guessed correctly. Only #3 will end the game. The number the user actually guessed is in the variable user_num. The number the game randomly generated is in the variable correct_num. The guess() code needs to do three things: (1) determine if the guess is too high, too low, or correct, (2) tell the player how they guessed (too high, too low, correctly), (3) return True if the user got the number or return False otherwise. #3 is required because we stop asking for guesses when your guess() function returns True. Otherwise, we keep looping. If the player's guess is too high, output "Your guess is too high!" and return False. If the player's guess is too low, output "Your guess is too low!" and return False. If the player's guess is correct, output "YOU GOT IT!" and return True. Testing For all input, know that the number is random, so you might get different results.Here's a sample interaction where the random number is -16. Enter your guess: 5 Your guess is too high! Enter your guess: -2 Your guess is too high! Enter your guess: -10 Your guess is too high! Enter your guess: -25 Your guess is too low! Enter your guess: -15 Your guess is too high! Enter your guess: -19 Your guess is too low! Enter your guess: -17 Your guess is too low! Enter your guess: -16 YOU GOT IT!
Problem
John is developing a python program that comes up with a number and allows the user to guess that number. The game allows the user to input their guess, which afterward, the game will tell the user one of three things: (1) the guessed number is too high, (2) the guessed number is too low, or (3) the guessed number is correct. If #3 occurs, then the game ends.
Learning Objectives
- Practice conditionally executing code.
- Practice reading portions of code already written for you.
- Understand the flow of a program.
- Understand how and when the program will be executed.
Template
from random import randint def guess(user_guess, correct_num): # If the user guesses wrong, tell them if their # guess is too low or too high, then return False.
# Fix the XXXXX's in the template below to get it to work correctly
if XXXXX:
# TODO: Print the output and return False elif XXXXX:
# TODO: Print the output and return False
else:
# TODO: If the user guesses correctly, congratulate them # and return True if __name__ == "__main__": num = randint(-50, 50) while True: user_guess = int(input("Enter your guess: ")) if guess(user_guess, num) == True: break
Assignment
Write the guess(user_num, correct_num) code.
- In the guess() code, you need a series of if statements to test three possibilities: (1) the player guessed too high, (2) the player guessed too low, or (3) the player guessed correctly. Only #3 will end the game.
- The number the user actually guessed is in the variable user_num. The number the game randomly generated is in the variable correct_num.
- The guess() code needs to do three things: (1) determine if the guess is too high, too low, or correct, (2) tell the player how they guessed (too high, too low, correctly), (3) return True if the user got the number or return False otherwise. #3 is required because we stop asking for guesses when your guess() function returns True. Otherwise, we keep looping.
- If the player's guess is too high, output "Your guess is too high!" and return False.
- If the player's guess is too low, output "Your guess is too low!" and return False.
- If the player's guess is correct, output "YOU GOT IT!" and return True.
Testing
For all input, know that the number is random, so you might get different results.Here's a sample interaction where the random number is -16.
Enter your guess: 5 Your guess is too high! Enter your guess: -2 Your guess is too high! Enter your guess: -10 Your guess is too high! Enter your guess: -25 Your guess is too low! Enter your guess: -15 Your guess is too high! Enter your guess: -19 Your guess is too low! Enter your guess: -17 Your guess is too low! Enter your guess: -16 YOU GOT IT!

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

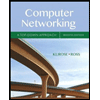
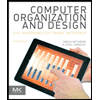
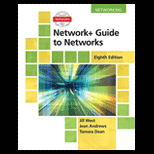
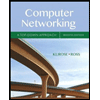
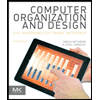
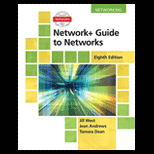
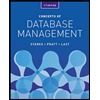
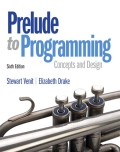
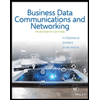