provide me all the files with all the functions implemented with proper code.file: fscMalloc.h #ifndef FSCMALLOC_H#define FSCMALLOC_H#ifdef __cplusplusextern "C" {#endif #include <stddef.h> #include <stdio.h> typedef struct { size_t size; int magic; } fsc_alloc_header_t; typedef struct _fsc_node_t { size_t size; struct _fsc_node_t* next; } fsc_free_node_t; typedef struct { fsc_free_node_t* head; int magicNumber; } memoryStructure; typedef enum { FIRST_FIT_RETURN_FIRST, FIRST_FIT_RETURN_SECOND, BEST_FIT_RETURN_FIRST, BEST_FIT_RETURN_SECOND, WORST_FIT_RETURN_FIRST, WORST_FIT_RETURN_SECOND, NEXT_FIT_RETURN_FIRST, NEXT_FIT_RETURN_SECOND } fscAllocationMethod; enum { MBToB = 1048576 }; void* fscMemorySetup(memoryStructure*, fscAllocationMethod, size_t sizeInBytes); void* fscMalloc(memoryStructure*, size_t sizeInBytes); // returns memory, 0 if failure void fscFree(memoryStructure*, void *); // returns memory to the pool void fscMemoryCleanup(memoryStructure*); void printFreeList(FILE * out, fsc_free_node_t* head); #ifdef __cplusplus}#endif #endif /* FSCMALLOC_H */ file: fscMalloc.c #include "fscMalloc.h"#include <assert.h>#include <sys/mman.h>#include <stdlib.h> /* for rand() */#include <time.h> /* for the init of rand */#include <stddef.h> /* for size_t */ void* fscMemorySetup(memoryStructure* m, fscAllocationMethod am, size_t sizeInBytes) { if (FIRST_FIT_RETURN_FIRST != am) { fprintf(stderr, "This code only supports the FIRST_FIT_RETURN_FIRST allocation method\n"); return 0; } /* You need to write the code here */ return 0;} void* fscMalloc(memoryStructure* m, size_t requestedSizeInBytes) { /* You need to write the code here */} void fscFree(memoryStructure* m, void * returnedMemory) {} /* Given a node, prints the list for you. */void printFreeList(FILE * out, fsc_free_node_t* head) { /* given a node, prints the list. */ fsc_free_node_t* current = head; fprintf(out, "About to dump the free list:\n"); while (0 != current) { fprintf(out, "Node address: %'u\t Node size (stored): %'u\t Node size (actual) %'u\t Node next:%'u\n", current, current->size, current->size + sizeof (fsc_free_node_t), current->next); current = current->next; }}
provide me all the files with all the functions implemented with proper code.
file: fscMalloc.h
#ifndef FSCMALLOC_H
#define FSCMALLOC_H
#ifdef __cplusplus
extern "C" {
#endif
#include <stddef.h>
#include <stdio.h>
typedef struct {
size_t size;
int magic;
} fsc_alloc_header_t;
typedef struct _fsc_node_t {
size_t size;
struct _fsc_node_t* next;
} fsc_free_node_t;
typedef struct {
fsc_free_node_t* head;
int magicNumber;
} memoryStructure;
typedef enum {
FIRST_FIT_RETURN_FIRST,
FIRST_FIT_RETURN_SECOND,
BEST_FIT_RETURN_FIRST,
BEST_FIT_RETURN_SECOND,
WORST_FIT_RETURN_FIRST,
WORST_FIT_RETURN_SECOND,
NEXT_FIT_RETURN_FIRST,
NEXT_FIT_RETURN_SECOND
} fscAllocationMethod;
enum { MBToB = 1048576 };
void* fscMemorySetup(memoryStructure*, fscAllocationMethod, size_t sizeInBytes);
void* fscMalloc(memoryStructure*, size_t sizeInBytes); // returns memory, 0 if failure
void fscFree(memoryStructure*, void *); // returns memory to the pool
void fscMemoryCleanup(memoryStructure*);
void printFreeList(FILE * out, fsc_free_node_t* head);
#ifdef __cplusplus
}
#endif
#endif /* FSCMALLOC_H */
file: fscMalloc.c
#include "fscMalloc.h"
#include <assert.h>
#include <sys/mman.h>
#include <stdlib.h> /* for rand() */
#include <time.h> /* for the init of rand */
#include <stddef.h> /* for size_t */
void* fscMemorySetup(memoryStructure* m, fscAllocationMethod am, size_t sizeInBytes) {
if (FIRST_FIT_RETURN_FIRST != am) {
fprintf(stderr, "This code only supports the FIRST_FIT_RETURN_FIRST allocation method\n");
return 0;
}
/* You need to write the code here
*/
return 0;
}
void* fscMalloc(memoryStructure* m, size_t requestedSizeInBytes) {
/* You need to write the code here
*/
}
void fscFree(memoryStructure* m, void * returnedMemory) {
}
/* Given a node, prints the list for you. */
void printFreeList(FILE * out, fsc_free_node_t* head) {
/* given a node, prints the list. */
fsc_free_node_t* current = head;
fprintf(out, "About to dump the free list:\n");
while (0 != current) {
fprintf(out,
"Node address: %'u\t Node size (stored): %'u\t Node size (actual) %'u\t Node next:%'u\n",
current,
current->size,
current->size + sizeof (fsc_free_node_t),
current->next);
current = current->next;
}
}

Step by step
Solved in 2 steps

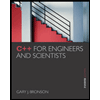
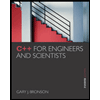