public Node(T el, Node l, Node r) { this.element = el; this.left = l; this.right = r; } // getters & setters public T getElement() { return element; } public void setElement(T el) { this.element = el; } public Node getLeft() { return left; } public Node getRight(){
!!! JAVA ONLY!!
Create a class Tree to model a binary tree. This class should contain a single Node which is the
root node of the tree, and should have appropriate getters/setters and toString().
Again, test out your class by creating a tree and nodes objects; and by calling various methods
on the them in the main method.
Create the following tree using instances of your classes:
Hoare
Jones
Fitzgerald Astarte
Roscoe
Broadfoot Creese
This tree captures PhD supervision hierarchy: Jones was supervised by Hoare, etc.
Write a method traceAndPrint for your Tree class. It should take as a parameter a string
of L and R characters, and, starting at the root, trace a path through the tree, taking a left
child when encountering L and a right child when encountering R. Each node passed, starting
with the root, should be printed to the console. If directions given are wrong, i.e. a null is
encountered, or any other character is passed to the method, an exception should be thrown.
The exception should indicate what the error is.
For example: using the tree from “LR” should print “Hoare, Jones, Astarte”. “RRR”
should throw an exception. “LZ” should throw an exception.
Hint: consider using the toCharArray method of the String class to help you parse the input
*****************************************************************************************************************
NODE.java
public class Node<T> {
// attributes: an element, and two children
private T element;
private Node<T> left;
private Node<T> right;
// simple constructor which wraps an element and sets no children
public Node(T el) {
this.element = el;
this.left = null;
this.right = null;
}
// constructor for creating Nodes with children right away
public Node(T el, Node<T> l, Node<T> r) {
this.element = el;
this.left = l;
this.right = r;
}
// getters & setters
public T getElement() {
return element;
}
public void setElement(T el) {
this.element = el;
}
public Node<T> getLeft() {
return left;
}
public Node<T> getRight(){
return right;
}
public void setLeft(Node<T> l) {
this.left = l;
}
public void setRight(Node<T> r) {
this.right = r;
}
// generates a string for printing, wrapping each Node in () for moderately easy viewing
public String toString() {
String out = "(";
// handle an empty node (though these should not be constructable!)
if (this.element == null) {
out += "<null> ";
} else {
out += "E: " + element.toString() + " ";
}
// stringify the left child and any of its children
out += "L: ";
if (this.left == null) {
out += "<null> ";
} else {
out += left.toString() + " ";
}
// stringify the right node and any of its children
out += "R: ";
if (this.right == null) {
out += "<null>";
} else {
out += right.toString() + " ";
}
out += ")";
return out;
}
}


Step by step
Solved in 2 steps

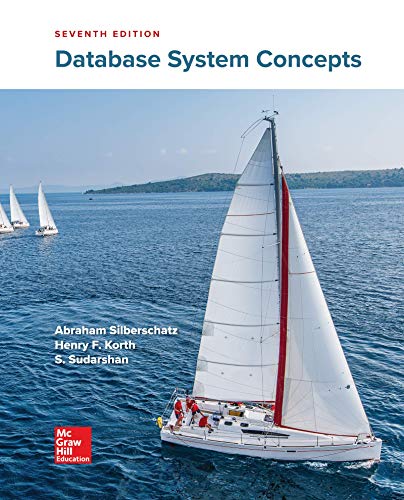
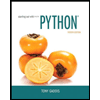
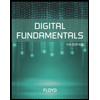
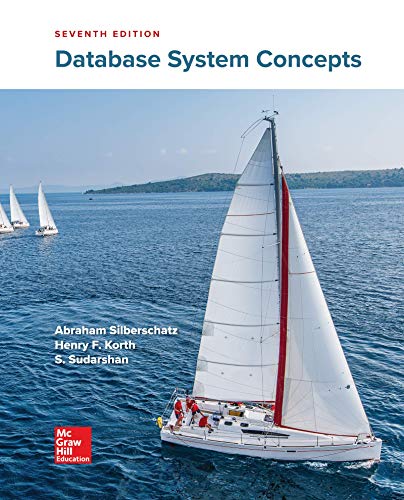
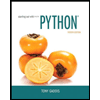
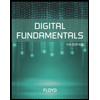
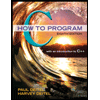
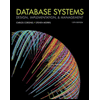
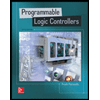