Python code not working, please check my code. Here is the question it is based off: Building and using DNA Motifs Background Sequence motifs are short, recurring (meaning conserved) patterns in DNA that are presumed to have a biological function. Often they indicate sequence-specific binding sites for proteins and other important markers. However, sometimes they are not exactly conserved, meaning some mutations can happen in a motif in a particular organism. Mutations can be DNA substitutions/deletions/insertions. Therefore, sequences are usually aligned and a consensus pattern of a motif is calculated over all examples from organisms. The following are examples of a transcription factor binding (TFB) site for the lexA repressor in_ E. Coli _located in a file called lexA.fasta: >dinD 32->52 aactgtatataaatacagtt >dinG 15->35 tattggctgtttatacagta >dinH 77->97 tcctgttaatccatacagca >dinI 19->39 acctgtataaataaccagta >lexA-1 28->48 tgctgtatatactcacagca >lexA-2 7->27 aactgtatatacacccaggg >polB(dinA) 53->73 gactgtataaaaccacagcc >recA 59->79 tactgtatgagcatacagta >recN-1 49->69 tactgtatataaaaccagtt >recN-2 27->47 tactgtacacaataacagta >recN-3 9-29 TCCTGTATGAAAAACCATTA >ruvAB 49->69 cgctggatatctatccagca >sosC 18->38 tactgatgatatatacaggt >sosD 14->34 cactggatagataaccagca >sulA 22->42 tactgtacatccatacagta >umuDC 20->40 tactgtatataaaaacagta >uvrA 83->103 tactgtatattcattcaggt >uvrB 75->95 aactgtttttttatccagta >uvrD 57->77 atctgtatatatacccagct Each line that starts with “>” is the header that states what gene this sequence was upstream of and where it is located relative to the gene. (For your purposes, we can ignore this and your code should ignore these lines when parsing the DNA sequences in). Each line in between is each nucleotide sequence of each TFB. Each nucleotide has a position in the sequence. You can assume that all sequences will be the same length. You also can do very minimal input error checking – I won’t be checking extensively for input error checking. However, do make sure that if a function relies on another function being run first, you have it do that. Creating DNAMOTIF class You will create a DNAMOTIF class that has the following attributes and functions: __init__(self): Initialize the class. self.instances=[] #These are a list of DNA sequence strings (no header) self.consensus=[] # A DNA sequence String self.counts= {'A': [], 'C': [], 'G':[],'T':[]} # A dictionary of nucleotide counts __str__: Return a string with the sequence instances of the motif on each line __len__: Return the length of a motif, which is the length of one of the sequences in the collection. Example Input: lexA=DNAMOTIF() lexA.parse("lexA.fasta") print(len(lexA)) Output: 20 parse(self,filename): read in DNA instances from a FASTA file Example Usage: lexA.parse("lexA.fasta") print(lexA) aactgtatataaatacagtt tattggctgtttatacagta tcctgttaatccatacagca acctgtataaataaccagta tgctgtatatactcacagca aactgtatatacacccaggg gactgtataaaaccacagcc tactgtatgagcatacagta tactgtatataaaaccagtt tactgtacacaataacagta TCCTGTATGAAAAACCATTA cgctggatatctatccagca tactgatgatatatacaggt cactggatagataaccagca tactgtacatccatacagta tactgtatataaaaacagta tactgtatattcattcaggt aactgtttttttatccagta atctgtatatatacccagct count(self): Count occurrences of A’s, C’s, G’s, and T’s in each position and store in a dictionary. Convert all sequences to upper case for consistency Example Input: lexA.count() To Access Result: lexA.counts={'A': [5, 13, 0, 0, 0, 1, 15, 1, 15, 4, 12, 6, 16, 6, 10, 0, 19, 0, 0, 12], 'C': [2, 3, 18, 0, 0, 0, 1, 2, 0, 1, 3, 6, 1, 4, 8, 19, 0, 0, 6, 1], 'G': [1, 2, 0, 0, 19, 3, 0, 1, 3, 1, 1, 0, 0, 0, 0, 0, 0, 18, 3, 1], 'T': [11, 1, 1, 19, 0, 15, 3, 15, 1, 13, 3, 7, 2, 9, 1, 0, 0, 1, 10, 5]} compute_consensus(self): Return an UPPERCASE sequence of the most frequent nucleotides in each position of the motif. If more than one are tied, return the first one lexicographically. Example Input: lexA.compute_consensus() To Access Result: print(lexA.consensus) TACTGTATATATATACAGTA
Python code not working, please check my code.
Here is the question it is based off:
Building and using DNA Motifs
Background
Sequence motifs are short, recurring (meaning conserved) patterns in DNA that are presumed to have a biological function. Often they indicate sequence-specific binding sites for proteins and other important markers. However, sometimes they are not exactly conserved, meaning some mutations can happen in a motif in a particular organism. Mutations can be DNA substitutions/deletions/insertions. Therefore, sequences are usually aligned and a consensus pattern of a motif is calculated over all examples from organisms.
The following are examples of a transcription factor binding (TFB) site for the lexA repressor in_ E. Coli _located in a file called lexA.fasta:
>dinD 32->52 aactgtatataaatacagtt >dinG 15->35 tattggctgtttatacagta >dinH 77->97 tcctgttaatccatacagca >dinI 19->39 acctgtataaataaccagta >lexA-1 28->48 tgctgtatatactcacagca >lexA-2 7->27 aactgtatatacacccaggg >polB(dinA) 53->73 gactgtataaaaccacagcc >recA 59->79 tactgtatgagcatacagta >recN-1 49->69 tactgtatataaaaccagtt >recN-2 27->47 tactgtacacaataacagta >recN-3 9-29 TCCTGTATGAAAAACCATTA >ruvAB 49->69 cgctggatatctatccagca >sosC 18->38 tactgatgatatatacaggt >sosD 14->34 cactggatagataaccagca >sulA 22->42 tactgtacatccatacagta >umuDC 20->40 tactgtatataaaaacagta >uvrA 83->103 tactgtatattcattcaggt >uvrB 75->95 aactgtttttttatccagta >uvrD 57->77 atctgtatatatacccagct
Each line that starts with “>” is the header that states what gene this sequence was upstream of and where it is located relative to the gene. (For your purposes, we can ignore this and your code should ignore these lines when parsing the DNA sequences in). Each line in between is each nucleotide sequence of each TFB. Each nucleotide has a position in the sequence. You can assume that all sequences will be the same length.
You also can do very minimal input error checking – I won’t be checking extensively for input error checking. However, do make sure that if a function relies on another function being run first, you have it do that.
Creating DNAMOTIF class
You will create a DNAMOTIF class that has the following attributes and functions:
- __init__(self): Initialize the class.
self.instances=[] #These are a list of DNA sequence strings (no header) self.consensus=[] # A DNA sequence String self.counts= {'A': [], 'C': [], 'G':[],'T':[]} # A dictionary of nucleotide counts
-
__str__: Return a string with the sequence instances of the motif on each line
-
__len__: Return the length of a motif, which is the length of one of the sequences in the collection.
Example Input:
lexA=DNAMOTIF() lexA.parse("lexA.fasta") print(len(lexA))
Output:
20
- parse(self,filename): read in DNA instances from a FASTA file
Example Usage:
lexA.parse("lexA.fasta") print(lexA) aactgtatataaatacagtt tattggctgtttatacagta tcctgttaatccatacagca acctgtataaataaccagta tgctgtatatactcacagca aactgtatatacacccaggg gactgtataaaaccacagcc tactgtatgagcatacagta tactgtatataaaaccagtt tactgtacacaataacagta TCCTGTATGAAAAACCATTA cgctggatatctatccagca tactgatgatatatacaggt cactggatagataaccagca tactgtacatccatacagta tactgtatataaaaacagta tactgtatattcattcaggt aactgtttttttatccagta atctgtatatatacccagct
- count(self): Count occurrences of A’s, C’s, G’s, and T’s in each position and store in a dictionary. Convert all sequences to upper case for consistency
Example Input:
lexA.count()
To Access Result:
lexA.counts={'A': [5, 13, 0, 0, 0, 1, 15, 1, 15, 4, 12, 6, 16, 6, 10, 0, 19, 0, 0, 12], 'C': [2, 3, 18, 0, 0, 0, 1, 2, 0, 1, 3, 6, 1, 4, 8, 19, 0, 0, 6, 1], 'G': [1, 2, 0, 0, 19, 3, 0, 1, 3, 1, 1, 0, 0, 0, 0, 0, 0, 18, 3, 1], 'T': [11, 1, 1, 19, 0, 15, 3, 15, 1, 13, 3, 7, 2, 9, 1, 0, 0, 1, 10, 5]}
- compute_consensus(self): Return an UPPERCASE sequence of the most frequent nucleotides in each position of the motif. If more than one are tied, return the first one lexicographically.
Example Input:
lexA.compute_consensus()
To Access Result:
print(lexA.consensus) TACTGTATATATATACAGTA
![main.py
Load default template.
self.counts= {'A': [], 'C': [), 'G':[],'T':[]}
def _str_(self):
string = ""
for i in self.instances:
string += i + "\n"
return string[:-2]
6
7
8
9.
10
11
def len_(self):
return len(self.instances)
12
13
14
15
def count(self):
for i in self.instances:
temp = i.upper()
self.counts["A"].append(temp.count("A"))
self.counts["C"].append (temp.count("C"))
self.counts["G"].append (temp.count("G"))
self.counts["T"].append(temp.count("T"))
16
17
18
19
20
21
22
def compute_consensus (self):
A = self.counts["A"]
C = self.counts["C"]
G = self.counts['G']
T = self.counts["T"]
23
24
25
26
27
28
for i in range (len(A)):
if(A[i] >= C[i] and A[i] >= G[i] and A[i] >= T[i]):
self.consensus.append("A")
elif (C[i] >= G[i] and C[i] >= T[i]):
self.consensus.append ("C")
elif (G[i] >= T[i]):
self.consensus.append("G")
29
30
31
32
33
34
35
36
else:
37
self.consensus.append("T")
38
def parse(self, filename):
with open(filename, 'r') as f:
for i in f:
if ">" in i:
continue
else:
39
40
41
42
43
44
45
self.instances.append (i)
46
When done developing your program, press the Submit for grading button below. This will
Develop mode
Submit mode
submit your program for auto-grading.
Submit for grading
Coding trail of your work What is this?
3/12 s0,0,0,2,2,2,2 U0 min:19
Latest submission - 1:13 AM EST on 03/13/22
Total score: 0/ 10
O Only show failing tests
Download this submission
1: test parse a
0/2
NameError: name 'lexA' is not defined
2: test len a
0/1
NameError: name 'lexA' is not defined
3: test counts a
0/3
NameError: name 'lexA' is not defined
4: test consensus a
0/4
NameError: name 'lexA' is not defined](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F347c60a8-9b8b-4262-9b10-12f3478495c2%2Fdb6c2f80-f7c2-48b5-9747-d22f2b84e848%2Fxkw2qj_processed.png&w=3840&q=75)

Step by step
Solved in 2 steps with 3 images

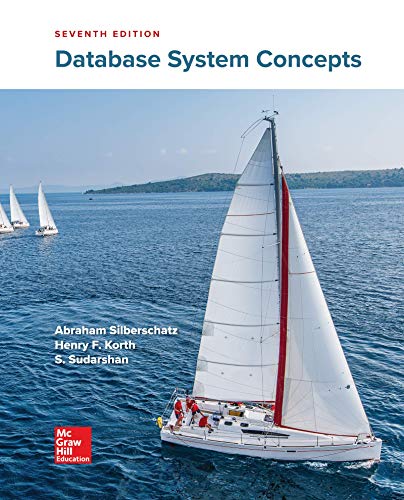
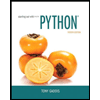
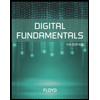
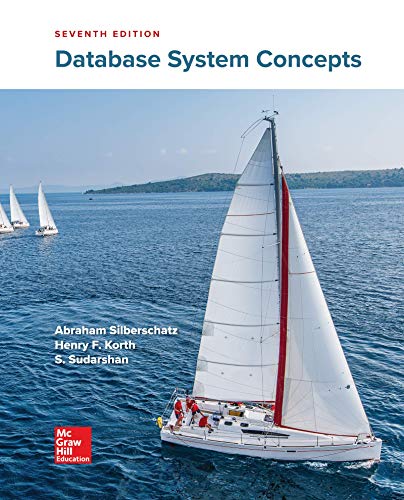
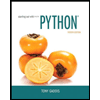
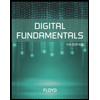
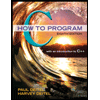
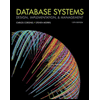
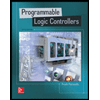