Python Coding Algorithm Please check the task description. Use the test case input as a test case to see if the final code works, as the code's output should be similar to the given sample output. Hopefully, you succeed. Task Identify the relationship between the sets of inputs. The inputs represent the wires in a Bulb's Wiring System. Concept -The Bulb system is identical to an electronic circuit, so Basic Circuit Rules and Ohm's Law apply. -Wires in a Series relationship are denoted as Linear, and those in Parallel are denoted as SidebySide -Therefore, the relationships are either Linear or SidebySide. If pairs of wires are neither Linear nor SidebySide, it will be denoted as having Neither relationship. -Wires with similar origins and destinations have a SidebySide relationship. On the other hand, Linear relationships are more complex as they require a continuous and singular wire connection. The flow of electricity starts from the "Start" point and ends at the "End" point. Note: Check Basic Circuit Rules if my explanation is difficult to understand as the task follows its rules. Input Format (See Test Case Input as reference) #1. Use the input() function to receive inputs #2. The first input asks for integers NumOfWires and NumOfCheck #3. Depending on the value of NumOfWires, Next Inputs will ask for WireName, Origin, Destination, Resistance - WireName, Origin, and Destination are strings, while Resistance is an integer #4. Based on the value of NumOfCheck, the last Inputs will ask for the strings ChWire1 and ChWire2 -ChWire1 and ChWire2 are the WireName of the wires whose relationship will be checked and printed Output Format (See Test Case Output as reference) #1. Prints only after the inputs are entered. #2. Print all sets with Linear or SidebySide Relationships line by line; -The number of lines depends on the number of Linear and SidebySide groups found -Sample strategy: Store those with relationships in a list of tuples [(w1, w2), (w5, w7), ...]; If w1 and w2 are in a relationship, the output look like this: [w1, w2] #3. Display the relationship for every ChWire1 and ChWire2 pairing from Input Format #4; -The number of lines depends on the value of NumOfCheck -Sample strategy: When checking the relationship between inputted wire pairs, determine if pairs are part of the same tuple by comparing them with the list made in Output #2 sample strategy. If they are, see whether the list/set is a Linear or SidebySide set. If they are not, the relationship is a Neither. (See Sample Logic) Conditions 1. Preferred to use standard algorithms like Prim's, Kruskal's, Dijkstra's, Bellman-Ford, Floyd-Warshall, and others to make the code run fast. 2. Only use standard libraries, for example, Math and Sys. 3. 1 ≤ NumOfWires, NumOfCheck ≤ 1000 and 0 < Resistance ≤ 109 Test Case Input (See the image for the illustration of this input) 9 4 W1 Start B2 100 W2 B2 B3 100 W3 B3 B4 30 W4 B3 B4 50 W5 B3 B4 70 W6 B4 B5 70 W7 B5 B6 30 W8 B6 End 35 W9 Start End 100 W3 W5 W8 W6 W1 W7 W9 W4 Test Case Output (If the test case input is entered, this should be the result for it to be correct) [W1, W2] [W3, W4, W5] [W6, W7, W8] SidebySide Linear Neither Neither
Python Coding Algorithm
Please check the task description. Use the test case input as a test case to see if the final code works, as the code's output should be similar to the given sample output. Hopefully, you succeed.
Task
Identify the relationship between the sets of inputs. The inputs represent the wires in a Bulb's Wiring System.
Concept
-The Bulb system is identical to an electronic circuit, so Basic Circuit Rules and Ohm's Law apply.
-Wires in a Series relationship are denoted as Linear, and those in Parallel are denoted as SidebySide
-Therefore, the relationships are either Linear or SidebySide. If pairs of wires are neither Linear nor SidebySide, it will be denoted as having Neither relationship.
-Wires with similar origins and destinations have a SidebySide relationship. On the other hand, Linear relationships are more complex as they require a continuous and singular wire connection. The flow of electricity starts from the "Start" point and ends at the "End" point.
Note: Check Basic Circuit Rules if my explanation is difficult to understand as the task follows its rules.
Input Format (See Test Case Input as reference)
#1. Use the input() function to receive inputs
#2. The first input asks for integers NumOfWires and NumOfCheck
#3. Depending on the value of NumOfWires, Next Inputs will ask for WireName, Origin, Destination, Resistance
- WireName, Origin, and Destination are strings, while Resistance is an integer
#4. Based on the value of NumOfCheck, the last Inputs will ask for the strings ChWire1 and ChWire2
-ChWire1 and ChWire2 are the WireName of the wires whose relationship will be checked and printed
Output Format (See Test Case Output as reference)
#1. Prints only after the inputs are entered.
#2. Print all sets with Linear or SidebySide Relationships line by line;
-The number of lines depends on the number of Linear and SidebySide groups found
-Sample strategy: Store those with relationships in a list of tuples [(w1, w2), (w5, w7), ...]; If w1 and w2 are in a relationship, the output look like this: [w1, w2]
#3. Display the relationship for every ChWire1 and ChWire2 pairing from Input Format #4;
-The number of lines depends on the value of NumOfCheck
-Sample strategy: When checking the relationship between inputted wire pairs, determine if pairs are part of the same tuple by comparing them with the list made in Output #2 sample strategy. If they are, see whether the list/set is a Linear or SidebySide set. If they are not, the relationship is a Neither. (See Sample Logic)
Conditions
1. Preferred to use standard
2. Only use standard libraries, for example, Math and Sys.
3. 1 ≤ NumOfWires, NumOfCheck ≤ 1000 and 0 < Resistance ≤ 109
Test Case Input (See the image for the illustration of this input)
9 4
W1 Start B2 100
W2 B2 B3 100
W3 B3 B4 30
W4 B3 B4 50
W5 B3 B4 70
W6 B4 B5 70
W7 B5 B6 30
W8 B6 End 35
W9 Start End 100
W3 W5
W8 W6
W1 W7
W9 W4
Test Case Output (If the test case input is entered, this should be the result for it to be correct)
[W1, W2]
[W3, W4, W5]
[W6, W7, W8]
SidebySide
Linear
Neither
Neither
Sample Logic (Other strategies can be used as long as Test Case Output is reached)
//Identify all Linear and SidebySide Wire Combinations
//Store Combinations in the list of tuples and mark if the combination is Linear or SidebySide
Ex. [(W1, W2), (W6, W7, W8)] Make an indicator to identify it as Linear
[(W3, W4, W5)] Make an indicator to identify it as SidebySide
//When checking happens, compare the given ChWire1 and ChWire2 with the stored tuples to test if the combination exists if there is none, this results in neither
Ex. W3 W5 combination exists in [(W3, W4, W5)] ; SidebySide
W8 W6 combination exists in [(W1, W2), (W6, W7, W8)] ; Linear
W1 W7 are part of [(W1, W2), (W6, W7, W8)], but they are not in the same tuple; Neither
W9 W4 relationship does not exist; Neither
Test Case Input Illustration
//See the image below
![Start
"
SERIES: [W1,W2]
B2
W1
100
TU
B3
W2
PARALLEL: [W3, W4,W5]
W3
30
(
100
W4
50
W5
70
A
W9
100
B4
W6
70
SERIES: [W6, W7, W8]
B5
W7
30
B6
W8
End](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2d64c7ed-10d5-40a6-a2f9-d17718796d50%2F718b0888-3561-41a4-9cab-bd93acbf3c39%2F0j2uejq_processed.jpeg&w=3840&q=75)

Step by step
Solved in 6 steps with 5 images

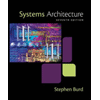
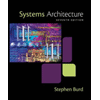