Question I need a java program for a Craps game When the program is run, it will prompt the player for the starting balance of their account (in dollars). At the start of each game, the player will be asked for their bet. The bet must be at least $1, but cannot exceed the balance of their account During the game, the outcome of each roll must be output to the display. When the outcome of the game is decided, the account balance must be updated accordingly based on the player’s bet. If the player wins, the amount of the bet is added to the account balance; if the player loses, the account balance is decreased by the bet amount. At the end of each game, the game outcome must be summarized (e.g., player wins or loses), along with the player’s updated account balance. The player will continue to play Craps games until one of the following conditions occur: They voluntarily elect to end the session. After each game, once all required information has been output, the player will be prompted to play another game or to end the session. Their account balance goes to zero. In this case, the program will automatically terminate the session At the conclusion of the session, the program will output the following information before terminating: Number of games played Percentage of games won and lost Current account balance Percentage increase or decrease in account balance since the session began. The Math.random() method must be used to generate the value for each dice roll. During the game, the outcome of each dice roll must be output to the display, nominally one line of output text per roll. Likewise, the outcome of the game must be summarized (player win or lose). Have the rolls initated automatically or manually(pressing the R key to do this) The program must make use of dialog and message boxes (JOptionPane class). Prompts for input to the user (e.g., taking bets) should employ these devices. At least one JOptionPane instance must be used in your program. Rules for the Game: At the start of the game, the player rolls two dice. Let x be sum of those dice. If x is 7 or 11, the player wins instantly If x is 2, 3, or 12, the player loses instantly If the sum is any other number, the player repeatedly rolls two dice until sum is either x or If sum is x then the player wins If the sum is 7 then the player loses Game Output: (It doesn't need to be exactly like this) Do you wish to play another game? y Please input your bet for the game >> $100 Invalid bet – Your balance is only: $50 Please input your bet for the game >> $25 Game #9 starting with bet of: $25.00 Player rolls: 11 Player wins :-^) ! Your account balance is now: $75 Do you wish to play another game? y Please input your bet for the game >> $75 Game #10 starting with bet of: $75.00 Player rolls: 10 -- must roll 10 again to win Player rolls: 12 Player rolls: 3 Player rolls: 7 Player loses :-(( Your account balance is now: $ 0.00 Sadly, you are no longer eligible to play Your ending account balance is: $ 0.00 Your starting balance has decreased by $200.00 (-100%) Of the 10 games played, you have won 3 games (30.0 %) and lost 7 games (70.0 %) Buh, bye – Get more $$ and play again soon! Exceptions: This is what I created for any potential errors. String response; while (true) { response = JOptionPane.showInputDIalog("Enter your strating account balance ($)"); try { startBalance = Double.parseDouble(response); } catch (NullPointerExcpetion e) { System.out.println("Error: The box cannot be empty!"); continue; } catch (NumberFormatException e) { System.out.println("Error: You must enter a numeric value in the box!"); continue; } if (startBalance >= MIN_INIT_BALANCE) break; System.out.printf("Please enter at least $%5.2f for a starting balance\n", MIN_INIT_BALANCE);
I need a java program for a Craps game
When the program is run, it will prompt the player for the starting balance of their account (in dollars).
At the start of each game, the player will be asked for their bet. The bet must be at least $1, but cannot exceed the balance of their account
During the game, the outcome of each roll must be output to the display.
When the outcome of the game is decided, the account balance must be updated accordingly based on the player’s bet. If the player wins, the amount of the bet is added to the account balance; if the player loses, the account balance is decreased by the bet amount.
At the end of each game, the game outcome must be summarized (e.g., player wins or loses), along with the player’s updated account balance.
The player will continue to play Craps games until one of the following conditions occur:
They voluntarily elect to end the session. After each game, once all required information has been output, the player will be prompted to play another game or to end the session.
Their account balance goes to zero. In this case, the program will automatically terminate the session
At the conclusion of the session, the program will output the following information before terminating:
Number of games played
Percentage of games won and lost
Current account balance
Percentage increase or decrease in account balance since the session began.
The Math.random() method must be used to generate the value for each dice roll.
During the game, the outcome of each dice roll must be output to the display, nominally one line of output text per roll. Likewise, the outcome of the game must be summarized (player win or lose).
Have the rolls initated automatically or manually(pressing the R key to do this)
The program must make use of dialog and message boxes (JOptionPane class). Prompts for input to the user (e.g., taking bets) should employ these devices. At least one JOptionPane instance must be used in your program.
Rules for the Game:
At the start of the game, the player rolls two dice. Let x be sum of those dice.
If x is 7 or 11, the player wins instantly
If x is 2, 3, or 12, the player loses instantly
If the sum is any other number, the player repeatedly rolls two dice until sum is either x or
If sum is x then the player wins
If the sum is 7 then the player loses
Game Output: (It doesn't need to be exactly like this)
Do you wish to play another game? y
Please input your bet for the game >> $100
Invalid bet – Your balance is only: $50
Please input your bet for the game >> $25
Game #9 starting with bet of: $25.00
Player rolls: 11
Player wins :-^) !
Your account balance is now: $75
Do you wish to play another game? y
Please input your bet for the game >> $75
Game #10 starting with bet of: $75.00
Player rolls: 10 -- must roll 10 again to win
Player rolls: 12
Player rolls: 3
Player rolls: 7
Player loses :-((
Your account balance is now: $ 0.00
Sadly, you are no longer eligible to play
Your ending account balance is: $ 0.00
Your starting balance has decreased by $200.00 (-100%)
Of the 10 games played, you have won 3 games (30.0 %) and lost 7 games (70.0 %)
Buh, bye – Get more $$ and play again soon!
Exceptions: This is what I created for any potential errors.
String response;
while (true)
{
response = JOptionPane.showInputDIalog("Enter your strating account balance ($)");
try
{
startBalance = Double.parseDouble(response);
}
catch (NullPointerExcpetion e)
{
System.out.println("Error: The box cannot be empty!");
continue;
}
catch (NumberFormatException e)
{
System.out.println("Error: You must enter a numeric value in the box!");
continue;
}
if (startBalance >= MIN_INIT_BALANCE)
break;
System.out.printf("Please enter at least $%5.2f for a starting balance\n", MIN_INIT_BALANCE);

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

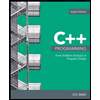
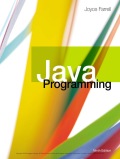
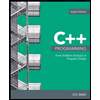
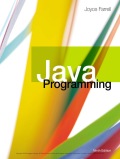
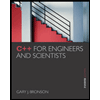
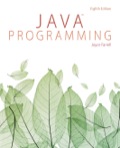