Repeat all activity from 2.3.4. Provide similar array manipulations using the months of the year. var days = ['Sunday', 'Monday', 'Tuesday']; console.log(days[0]); console.log(days[2]); days[3] = 'Wednesday'; console.log(days.toString()); days[6] = 'Saturday'; console.log(days.toString()); var theDayOff = days.slice(6) console.log(theDayOff.toString()); console.log(days.toString()); console.log(days.toString()); days.splice(4, 2, "Thursday", "Friday"); console.log(days.toString()); console.log(days.toString()); days.push('theExtraDay') console.log(days.toString()); let extras = days.pop(); console.log(days.toString()); console.log(extras); days.sort(); console.log(days.toString());
Repeat all activity from 2.3.4. Provide similar array manipulations using the months of the year. var days = ['Sunday', 'Monday', 'Tuesday']; console.log(days[0]); console.log(days[2]); days[3] = 'Wednesday'; console.log(days.toString()); days[6] = 'Saturday'; console.log(days.toString()); var theDayOff = days.slice(6) console.log(theDayOff.toString()); console.log(days.toString()); console.log(days.toString()); days.splice(4, 2, "Thursday", "Friday"); console.log(days.toString()); console.log(days.toString()); days.push('theExtraDay') console.log(days.toString()); let extras = days.pop(); console.log(days.toString()); console.log(extras); days.sort(); console.log(days.toString());
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Repeat all activity from 2.3.4. Provide similar array manipulations using the months of the year.
var days = ['Sunday', 'Monday', 'Tuesday'];
console.log(days[0]);
console.log(days[2]);
days[3] = 'Wednesday';
console.log(days.toString());
days[6] = 'Saturday';
console.log(days.toString());
var theDayOff = days.slice(6)
console.log(theDayOff.toString());
console.log(days.toString());
console.log(days.toString());
days.splice(4, 2, "Thursday", "Friday");
console.log(days.toString());
console.log(days.toString());
days.push('theExtraDay')
console.log(days.toString());
let extras = days.pop();
console.log(days.toString());
console.log(extras);
days.sort();
console.log(days.toString());
![Or even more unusual:
With the result
Sunday
Tuesday
Sunday, Monday, Tuesday, Wednesday
The results:
Sunday, Monday, Tuesday, Wednesday,,,Saturday
What is more interesting: some specific method like slice and splice
The slice() method slices out a piece of an array into a new array
var theDayOff days.slice(6)
console.log theDayoff.toString());
6 days [6] "Saturday";
console.log(days.toString());
console.log(days.toString());
With the result:
Saturday
Sunday, Monday, Tuesday, Wednesday,,,Saturday
The splice() method can be used to add new items to an array:
console.log(days.toString());
days.splice 4, 2, "Thursday", "Friday");
console.log(days.toString());
With the result
Sunday, Monday, Tuesday, Wednesday,,,Saturday
Sunday, Monday, Tuesday, Wednesday, Thursday, Friday, Saturday
There are several other methods we can also mention such as push(), pop() and sort()
The examples are below:
12
console.log(days.toString());
13
days.push("the ExtraDay')
14 console.log(days.toString());
15 let extras days.pop();
16 console.log(days.toString());
17 console.log(extras);
18
days.sort();
19
console.log(days.toString());
Sunday, Monday, Tuesday, Wednesday...Saturday
Sunday, Monday, Tuesday, Wednesday, Thursday, Friday, Saturday
Sunday, Monday, Tuesday, Wednesday, Thursday, Friday, Saturday, the Extrabay
Sunday, Monday, Tuesday, Wednesday, Thursday, Friday, Saturday
theExtraDay
Friday, Monday, Saturday, Sunday, Thursday, Tuesday, Wednesday
As we can see the strings were sorted alphabetically
Repeat all activity from 2.3.4. Provide similar array manipulations using the months of the year.
TASK 5. Perform all required activities in 2.3.1-2.3.4, submit your
18](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2ae76079-938b-4b5d-8ceb-1dfbc10f88ce%2F75d7df91-a495-4aeb-9651-295527370b0e%2Ffu48vxd_processed.png&w=3840&q=75)
Transcribed Image Text:Or even more unusual:
With the result
Sunday
Tuesday
Sunday, Monday, Tuesday, Wednesday
The results:
Sunday, Monday, Tuesday, Wednesday,,,Saturday
What is more interesting: some specific method like slice and splice
The slice() method slices out a piece of an array into a new array
var theDayOff days.slice(6)
console.log theDayoff.toString());
6 days [6] "Saturday";
console.log(days.toString());
console.log(days.toString());
With the result:
Saturday
Sunday, Monday, Tuesday, Wednesday,,,Saturday
The splice() method can be used to add new items to an array:
console.log(days.toString());
days.splice 4, 2, "Thursday", "Friday");
console.log(days.toString());
With the result
Sunday, Monday, Tuesday, Wednesday,,,Saturday
Sunday, Monday, Tuesday, Wednesday, Thursday, Friday, Saturday
There are several other methods we can also mention such as push(), pop() and sort()
The examples are below:
12
console.log(days.toString());
13
days.push("the ExtraDay')
14 console.log(days.toString());
15 let extras days.pop();
16 console.log(days.toString());
17 console.log(extras);
18
days.sort();
19
console.log(days.toString());
Sunday, Monday, Tuesday, Wednesday...Saturday
Sunday, Monday, Tuesday, Wednesday, Thursday, Friday, Saturday
Sunday, Monday, Tuesday, Wednesday, Thursday, Friday, Saturday, the Extrabay
Sunday, Monday, Tuesday, Wednesday, Thursday, Friday, Saturday
theExtraDay
Friday, Monday, Saturday, Sunday, Thursday, Tuesday, Wednesday
As we can see the strings were sorted alphabetically
Repeat all activity from 2.3.4. Provide similar array manipulations using the months of the year.
TASK 5. Perform all required activities in 2.3.1-2.3.4, submit your
18
![2.3.4. Arrays
Arrays in JS are Objects.
Create a file arrays.js
We will start from the basics.
Will bring us the output
With the output
chapter08> arrays.js ›...
With the result
Looks easy. Brackets denote an array the same as in many other languages.
Arrays in JavaScript does not have a fix size, so we can easily do something like this:
chapter>amays.js > ...
var days ['Sunday'", "Monday", "Tuesday"];
console.log(days[0]);
var days ["Sunday", "Monday", "Tuesday'];
The results:
console.log(days[0]);
console.log(days[2]);
PS C:\Users\galae\OneDrive\Desktop\35\chapter68> node arrays
Sunday
Tuesday
Or even more unusual:
3 console.log(days [2]);
4 days [3] 'Wednesday";
$ console.log days.toString());
PS C:\Users\galae\OneDrive\Desktop\35\chapter88> node arrays
Sunday
Tuesday
Sunday, Monday, Tuesday, Wednesday
days [6] "Saturday";
7 console.log(days.toString());
Sunday, Monday, Tuesday, Wednesday...Saturday
What is more interesting: some specific method like slice and splice
The slice method slices out a piece of an array into a new array
var theDayoff days.slice(6)
console.logtheDayoff.toString());
console.log(days.toString());
Saturday
Sunday, Monday, Tuesday, Wednesday,,,Saturday
The splice() method can be used to add new items to an array:
console.log(days.toString());
days.splice 4, 2, "Thursday", "Friday");
console.log(days.toString());
With the result
Sunday, Monday, Tuesday, Wednesday,,,Saturday
Sunday, Monday, Tuesday, Wednesday, Thursday, Friday Saturday
17](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2ae76079-938b-4b5d-8ceb-1dfbc10f88ce%2F75d7df91-a495-4aeb-9651-295527370b0e%2F1wj9yo3_processed.png&w=3840&q=75)
Transcribed Image Text:2.3.4. Arrays
Arrays in JS are Objects.
Create a file arrays.js
We will start from the basics.
Will bring us the output
With the output
chapter08> arrays.js ›...
With the result
Looks easy. Brackets denote an array the same as in many other languages.
Arrays in JavaScript does not have a fix size, so we can easily do something like this:
chapter>amays.js > ...
var days ['Sunday'", "Monday", "Tuesday"];
console.log(days[0]);
var days ["Sunday", "Monday", "Tuesday'];
The results:
console.log(days[0]);
console.log(days[2]);
PS C:\Users\galae\OneDrive\Desktop\35\chapter68> node arrays
Sunday
Tuesday
Or even more unusual:
3 console.log(days [2]);
4 days [3] 'Wednesday";
$ console.log days.toString());
PS C:\Users\galae\OneDrive\Desktop\35\chapter88> node arrays
Sunday
Tuesday
Sunday, Monday, Tuesday, Wednesday
days [6] "Saturday";
7 console.log(days.toString());
Sunday, Monday, Tuesday, Wednesday...Saturday
What is more interesting: some specific method like slice and splice
The slice method slices out a piece of an array into a new array
var theDayoff days.slice(6)
console.logtheDayoff.toString());
console.log(days.toString());
Saturday
Sunday, Monday, Tuesday, Wednesday,,,Saturday
The splice() method can be used to add new items to an array:
console.log(days.toString());
days.splice 4, 2, "Thursday", "Friday");
console.log(days.toString());
With the result
Sunday, Monday, Tuesday, Wednesday,,,Saturday
Sunday, Monday, Tuesday, Wednesday, Thursday, Friday Saturday
17
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
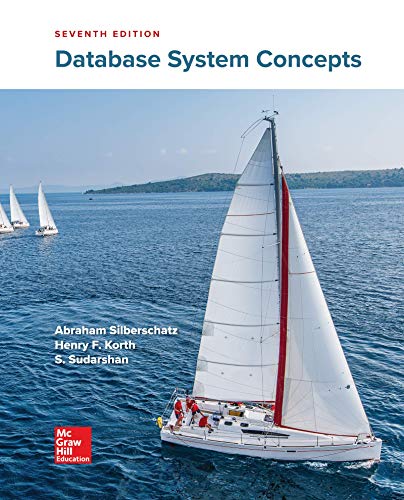
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
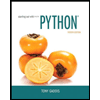
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
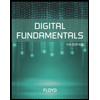
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
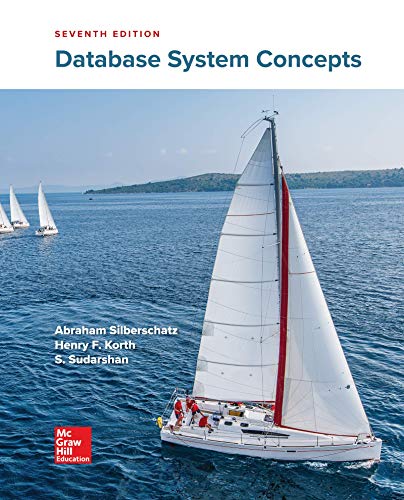
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
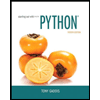
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
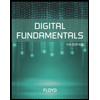
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
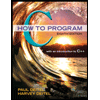
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
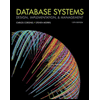
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
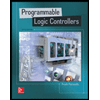
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education