[REPOST] Instructions: (USE JAVA LANGUAGE) Kindly modify, add on where it is lacking and execute the java program provided below. Don't forget to provide a screenshot of the program output. SimpleGUI.java import java.awt.*; import javax.swing.JPanel; public class SimpleGUI extends JPanel { //Contructor to have panel public SimpleGUI(Color color, int length, int width) { super.setBackground(color); super.setPreferredSize(new Dimension(length, width)); } public void paintComponent(Graphics page) { super.paintComponent(page); /* The coordinate system for the Java graphing window positions (0,0) in the upper left hand corner. The grid is then numbered in a positive direction on the x-axis (horizontally to the right) and in a positive direction on the y-axis (vertically going down). We will now be using the methods contained within the Graphics Class in the package java.awt */ //drawLine(int x1, int y1, int x2, int y2) //draw a straight line from point (x1,y1) to (x2,y2). page.setColor(Color.black); page.drawLine(200, 10, 300, 30); //exercise 1: craete your own line with different color, position and size /* drawRect(int x, int y, int width, int length) draw a rectangle with the upper left corner at (x,y) and with the specified width and length. fillRect is the same as drawRect except just it is filled */ page.setColor(Color.gray); page.fillRect(60, 30, 100, 50); //exercise 2: create your own rectangle /*drawOval(int x, int y, int width, int length) draw an oval inside an imaginary rectangle whose upper left corner is at (x,y). To draw a circle keep the width and length the same. */ page.setColor(Color.yellow); page.fillOval(120, 120, 120, 80); page.setColor(http://Color.red); page.drawRect(120, 120, 120, 80); //exercise 3: craete your own oval /* drawArc(int x, int y, int width, int length, int startAngle, int arcAngle) draw an arc inside an imaginary rectangle whose upper left corner is at (x,y). The arc is drawn from the startAngle to startAngle + arcAngle and is measured in degrees. A startAngle of 0º points horizontally to the right (like the unit circle in math). Positive is a counterclockwise rotation starting at 0º. */ page.setColor(http://Color.blue); page.fillArc(200, 50, 100, 50, 0, 90); //exercise 4: craete your own arc /* drawPolygon(int x[ ], int y[ ], int n) draw a polygon created by n line segments. The command will close the polygon. (x-coordinates go in one array with accompanying y-coordinates in the other) */ int [] x = {340, 250, 300}; int [] y = {110, 150, 170}; page.setColor(Color.pink); page.drawPolygon(x, y, 3); //exercise 5: craete your own polygon with 5 points /* drawString(String str, int x, int y); Draws a string starting at the point indicated by (x,y). Be sure you leave enough room from the top of the screen for the size of the font. */ page.setColor(Color.magenta); page.drawString("Great Job!", 300, 230); //exercise 6: craete your own message } }
[REPOST] Instructions: (USE JAVA LANGUAGE) Kindly modify, add on where it is lacking and execute the java program provided below. Don't forget to provide a screenshot of the program output.
SimpleGUI.java
import java.awt.*;
import javax.swing.JPanel;
public class SimpleGUI extends JPanel {
//Contructor to have panel
public SimpleGUI(Color color, int length, int width) {
super.setBackground(color);
super.setPreferredSize(new Dimension(length, width));
}
public void paintComponent(Graphics page) {
super.paintComponent(page);
/* The coordinate system for the Java graphing window positions (0,0) in the upper left hand corner.
The grid is then numbered in a positive direction on the x-axis (horizontally to the right) and
in a positive direction on the y-axis (vertically going down).
We will now be using the methods contained within the Graphics Class in the package java.awt
*/
//drawLine(int x1, int y1, int x2, int y2)
//draw a straight line from point (x1,y1) to (x2,y2).
page.setColor(Color.black);
page.drawLine(200, 10, 300, 30);
//exercise 1: craete your own line with different color, position and size
/* drawRect(int x, int y, int width, int length)
draw a rectangle with the upper left corner at (x,y) and with the specified width and length.
fillRect is the same as drawRect except just it is filled
*/
page.setColor(Color.gray);
page.fillRect(60, 30, 100, 50);
//exercise 2: create your own rectangle
/*drawOval(int x, int y, int width, int length)
draw an oval inside an imaginary rectangle whose upper left corner is at (x,y). To draw a circle keep the width and length the same.
*/
page.setColor(Color.yellow);
page.fillOval(120, 120, 120, 80);
page.setColor(http://Color.red);
page.drawRect(120, 120, 120, 80);
//exercise 3: craete your own oval
/* drawArc(int x, int y, int width, int length,
int startAngle, int arcAngle)
draw an arc inside an imaginary rectangle whose upper left corner is at (x,y). The arc is drawn from the startAngle to startAngle + arcAngle and is measured in degrees.
A startAngle of 0º points horizontally to the right (like the unit circle in math). Positive is a counterclockwise rotation starting at 0º.
*/
page.setColor(http://Color.blue);
page.fillArc(200, 50, 100, 50, 0, 90);
//exercise 4: craete your own arc
/* drawPolygon(int x[ ], int y[ ], int n)
draw a polygon created by n line segments. The command will close the polygon. (x-coordinates go in one array with accompanying y-coordinates in the other)
*/
int [] x = {340, 250, 300};
int [] y = {110, 150, 170};
page.setColor(Color.pink);
page.drawPolygon(x, y, 3);
//exercise 5: craete your own polygon with 5 points
/* drawString(String str, int x, int y);
Draws a string starting at the point indicated by (x,y). Be sure you leave enough room from the top of the screen for the size of the font.
*/
page.setColor(Color.magenta);
page.drawString("Great Job!", 300, 230);
//exercise 6: craete your own message
}
}

Step by step
Solved in 4 steps with 4 images

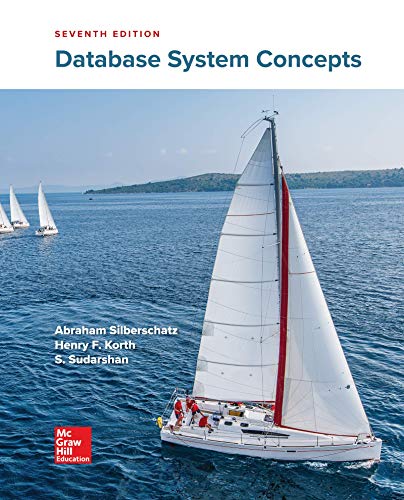
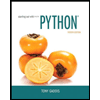
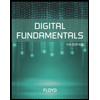
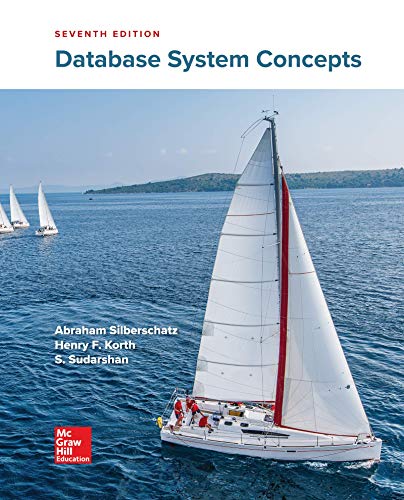
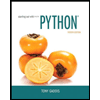
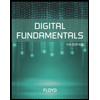
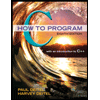
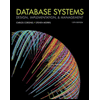
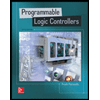