RPN (Reverse Polish Notation) is a postfix method of expression mathem functions.
Read all of the directions in the attached picture
AnsweAnyPartYouCan
UseAll8ClassesBelowToStartThis
package cs232.RPNCalc;
import java.util.ArrayList;
public interface RPNStringTokenizer {
public static ArrayList<String> tokenize (String expression) {
// take a string. If it is valid RPN stuff - integers or operators
// - then put them in a list for processing.
// anything bad (not int or one of the operators we like) and return a null.
// YOU WRITE THIS!
return null;
}
}
package cs232.RPNCalc;
import java.util.ArrayList;
public class RPNTester {
public static void main(String[] args) {
// don't change this method!
YourRPNCalculator calc = new YourRPNCalculator(new YourArrayListStack());
System.out.println("Testing ArrayList version");
testRPNCalculator(calc);
calc = new YourRPNCalculator(new YourLinkedListStack());
System.out.println("Testing LinkedList version");
testRPNCalculator(calc);
}
private static void testRPNCalculator(SimpleRPNCalculator calc) {
ArrayList<String> testExpressions = new ArrayList<>();
testExpressions.add("1 1 +"); // 2
testExpressions.add("1 3 -"); // 2
testExpressions.add("1 1 + 2 *"); // 4
testExpressions.add("1 1 2 + *"); // 3
testExpressions.add("1 1 + 2 2 * -"); // 2
testExpressions.add("11 bv +"); // bad token
testExpressions.add("2 3 + -"); // underflow on an operator
testExpressions.add("2 3 + 4 5 -"); // leftover tokens
// YOU SHOULD ADD MORE TEST CASES!
for (String s : testExpressions) {
System.out.println(calc.calculate(s));
}
}
}
package cs232.RPNCalc;
public interface SimpleRPNCalculator {
public String calculate(String inputString);
/*
* RPN (Reverse Polish Notation) is a postfix method of expression mathematical functions.
* It is traditionally used to eliminate the necessity of parenthesis by removing the
* order of operations and acting on operators as they occur.
*
* Very simply, INFIX notation "1 + 1" is represented in POSTFIX notation as "1 1 +"
* Longer expressions may change radically. "1 + 2 * 3" assumes an order of operations,
* while in POSTFIX, "2 3 * 1 +" or "1 2 3 * +"
*
* The calculator works as follows:
* if number, push to a stack
* if operator, pop two numbers, operate on them, and push the result
* if I hit an operator and there are not two numbers on the stack, it's a bad expression
* if I get to the end of the expression and there is not exactly one number on the stack,
* it's a bad expression.
*
* Making something that looks like it works - the input to output happening without a crash
* Implement LinkedListBasedStack
* Implement ArrayList
*
* YOUR CALCULATOR ONLY NEEDS TO WORK FOR +, -, and *
* YOUR CALCULATOR NEEDS TO WORK ON INTEGERS.
*
* Has to work on Doubles (this is a gimme)
* Bonus points for implementing division / (this is deceptively hard, DO THIS LAST!)
*
*
*/
}
package cs232.RPNCalc;
public interface TGStack {
public void push (Integer i);
public Integer pop();
public Integer size();
}
package cs232.RPNCalc;
import java.util.ArrayList;
public class YourArrayListStack implements TGStack {
// YOU MUST USE THIS IMPLEMENTATION - just code the methods
ArrayList<Integer> theStack = new ArrayList<>();
@Override
public void push(Integer i) {
}
@Override
public Integer pop() {
return null;
}
@Override
public Integer size() {
return null;
}
}
package cs232.RPNCalc;
public class YourLinkedListStack implements TGStack {
// YOU MUST USE THIS IMPLEMENTATION - just code the methods
private YourStackNode head = null;
private Integer size = 0;
@Override
public void push(Integer i) {
}
@Override
public Integer pop() {
return null;
}
@Override
public Integer size() {
return null;
}
}
package cs232.RPNCalc;
import java.util.ArrayList;
public class YourRPNCalculator implements SimpleRPNCalculator {
// don't change these...
TGStack theStack = null;
public YourRPNCalculator(TGStack stack) {
theStack = stack;
}
@Override
public String calculate(String inputString) {
// this is probably helpful, but you can remove...
ArrayList<String> tokens = RPNStringTokenizer.tokenize(inputString);
// here's the calculator logic!
return null;
}
}
package cs232.RPNCalc;
public class YourStackNode {
// DO NOT CHANGE THIS!
private Integer item;
private YourStackNode next;
public Integer getItem() {
return item;
}
public YourStackNode getNext() {
return next;
}
public void setItem (Integer i) {
item = i;
}
public void setNext (YourStackNode node) {
next = node;
}
}

![package cs232. RPNCalc;
package cs232. RPNCalc;
RPNStringTokenizer.java
YourArrayListStack.java
import java.util.ArrayList;
import java.util.ArrayList;
public interface RPNStringTokenizer {
public class YourArrayListStack implements TGStack {
public static ArrayList<String> tokenize (String expression) {
// YOU MUST USE THIS IMPLEMENTATION -
just code the methods
// take a string.
// - then put them in a list for processing.
// anything bad (not int or one of the operators we like) and return a null.
If it is valid RPN stuff - integers or operators
ArrayList<Integer> theStack = new ArrayList<>();
@Override
public void push(Integer i) {
// YOU WRITE THIS!
return null;
}
@Override
public Integer pop() {
return null;
package cs232.RPNCalc;
}
RPNTester.java
import java.util.ArrayList;
@Override
public Integer size() {
public class RPNTester {
return null;
public static void main(String[] args) {
}
// don't change this method!
YourRPNCalculator calc = new YourRPNCalculator(new YourArrayListStack());
System.out.println("Testing ArrayList version");
testRPNCalculator(calc);
}
YourLinkedListStack.java
package cs232. RPNCalc;
calc = new YourRPNCalculator (new YourLinkedListStack());
System.out.println("Testing LinkedList version");
testRPNCalculator(calc);
public class YourLinkedListStack implements TGStack {
// YOU MUST USE THIS IMPLEMENTATION -
private YourStackNode head = null;
private Integer size = 0;
just code the methods
}
private static void testRPNCalculator(SimpleRPNCalculator calc) {
@Override
public void push(Integer i) {
ArrayList<String> testExpressions = new ArrayList<>();
testExpressions.add("1 1 +"); // 2
testExpressions.add("1 3 -"); // 2
testExpressions.add("1 1 + 2 *"); // 4
testExpressions.add("1 1 2 + *"); // 3
testExpressions.add("1 1 + 2 2 * -"); // 2
testExpressions.add("11 bv +"); // bad token
testExpressions.add("2 3 +
testExpressions.add("2 3 + 4 5 -"); // leftover tokens
// YOU SHOULD ADD MORE TEST CASES!
}
@Override
public Integer pop() {
return null;
-"); // underflow on an operator
}
@Override
public Integer size() {
for (String s : testExpressions) {
return null;
System.out.println(calc.calculate(s));
}
}
}
}
package cs232. RPNCalc;
YourRPNCalculator.java
import java.util.ArrayList;
package cs232. RPNCalc;
public interface SimpleRPNCalculator ( SimpleRPNCalculator.java
public String calculate(String inputString);
public class YourRPNCalculator implements SimpleRPNCalculator {
// don't change these...
TGStack theStack = null;
/*
public YourRPNCalculator (TGStack stack) {
theStack = stack;
* RPN (Reverse Polish Notation) is a postfix method of expression mathematical functions.
* It is traditionally used to eliminate the necessity of parenthesis by removing the
* order of operations and acting on operators as they occur.
}
* Very simply, INFIX notation "1 + 1" is represented in POSTFIX notation as "1 1 +"
* Longer expressions may change radically.
* while in POSTFIX, "2 3 * 1 +" or "1 2 3 *
@Override
public String calculate(String inputString) {
"1 + 2 * 3" assumes an order of operations,
// this is probably helpful, but you can remove...
*
ArrayList<String> tokens = RPNStringTokenizer.tokenize (inputString);
* The calculator works as follows:
* if number, push to a stack
* if operator, pop two numbers, operate on them, and push the result
* if I hit an operator and there are not two numbers on the stack, it's a bad expression
* if I get to the end of the expression and there is not exactly one number on the stack,
it's a bad expression.
// here's the calculator logic!
return null;
}
}
- the input to output happening without a crash
* Make something that looks like it works
Implement LinkedListBasedStack
Implementing ArrayList
*
package cs232. RPNCalc;
YourStackNode.java
*
public class YourStackNode {
// DO NOT CHANGE THIS!
private Integer item;
private YourStackNode next;
* YOUR CALCULATOR ONLY NEEDS TO WORK FOR +, -,
* YOUR CALCULATOR NEEDS TO WORK ON INTEGERS.
and *
* Has
work on Doubles
his is
* Bonus points for implementing division / (this is deceptively hard, DO THIS LAST!)
public Integer getItem() {
return item;
*
}
public YourstackNode getNext() {
return next;
}
}
package cs232. RPNCalc;
TGStack.java
public void setItem (Integer i) {
i;
public interface TGStack {
item =
public void push (Integer i);
public Integer pop();
public Integer size();
}
public void setNext (YourStackNode node) {
}
next = node;
}
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F69744928-076a-4b2a-9868-f845c79a7eed%2F51eafbb4-8ce2-455f-a1c5-6872ca5ce0b0%2Fja587dk_processed.png&w=3840&q=75)

Given
RPN (Reverse Polish Notation) is a postfix method of expression mathematical functions.
It is traditionally used to eliminate the necessity of parenthesis by removing the order of operations and acting on operators as they occur.
Very simply, INFIX notation "1 + 1" is represented in POSTFIX notation as: "1 1 +". Longer expressions may change radically. "1 + 2 * 3" assumes an order of operations, while in POSTFIX, "2 3 * 1 +" or "1 2 3 * +"
The calculator works as follows: if number, push to a stack if operator, pop two numbers, operate on them, and push the result if I hit an operator and there are not two numbers on the stack, it's a bad expression if I get to the end of the expression and there is not exactly one number on the stack, it's a bad expression.
Make something that looks like it works — the input to output happening without a crash Implement LinkedListBasedStack Implement ArrayList
YOUR CALCULATOR ONLY NEEDS TO WORK FOR +, —, and * YOUR CALCULATOR NEEDS TO WORK ON INTEGERS.
Has towork on Doubles (this is a gimme) Bonus points for implementing division / (this is deceptively hard, DO THIS LAST!)
Make sure to use all 8 classes given -> to begin this question. please and thank you!
This question is very easy, just a lot of simple directions but EVERYTHING IS ALREADY SET UP for you to the right.
(Create a new package/project. Add these 8 java files to that folder. Follow the simple directions given to complete this quick question.
I hope you have a great day! Thanks for helping me.
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

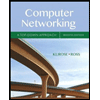
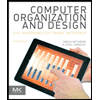
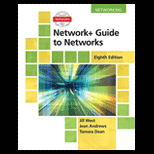
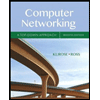
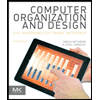
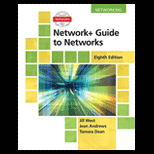
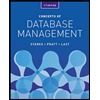
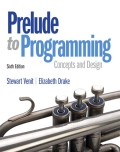
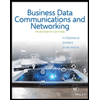