Step 1: Start the IDLE Environment for Python. Prior to entering code, save your file by clicking on File and then Save. Select your location and save this file as Lab5-3.py. Be sure to include the .py extension. Step 2: Document the first few lines of your program to include your name, the date, and a brief description of what the program does. Step 3: Start your program with the following code for main: # Lab 5-3 Practicing for loops # A Basic For loop # The Second Counter code # The Accumulator code # The Average Age code Step 4: Under the documentation for A Basic For Loop, add the following lines of code: print('I will display the numbers 1 through 5.') for num in [1, 2, 3, 4, 5]: print num On the first iteration, 1 is placed into the variable num and num is then printed to the screen. The process is continued as follows: Execute your program. Notice that the output is as follows: >>> I will display the numbers 1 through 5. 1 2 3 4 5 >>> Step 5: The next loop to code is the Second Counter code. This loop can be processed in the same way as Step 4; however, it would take a long time to write 1 through 60 in the for loop definition. Therefore, the range function should be used to simplify the process. Write a for loop that has a range from 1 to 61. If you stop at 60, only 59 seconds will be printed. If you only provide one argument, the starting value will be 0. (Reference the Critical Review section above for the exact syntax.) Step 6: The next loop to code is the Accumulator code. Start by initializing a total variable to 0. This must be done in order to accumulate values. Step 7: The next step is to write a for loop that iterates 5 times. The easiest way to do this is the following. for counter in range(5): Step 8: Inside the for loop, allow the user to enter a number. Then, add an accumulation statement that adds the number to total. In Python, the range function determines the number of iterations, so it is not necessary to manually increment counter. Step 9: Outside of the for loop, use a print statement that will display the total. Step 10: Compare your sample input and output to the following: Enter a number: 54 Enter a number: 32 Enter a number: 231 Enter a number: 23 Enter a number: 87 The total is 427 Step 11: The final loop to code is the Average Age code. Start by initializing totalAge and averageAge to 0. (Reference the Critical Review section above on Letting the User Control the Number of Iterations). Step 12: The next step is to ask how many ages they want to enter. Store the answer in the number variable. Step 13: Write the definition for the for loop using the range function such as: for counter in range(0, number): Step 14: Inside the for loop, allow the user to enter an age. Step 15: Inside the for loop, add the code that will accumulate age into the totalAge variable. Step 16: Outside of the loop, calculate the average age as averageAge = totalAge / number. Step 17: Outside of the loop, display the averageAge variable to the screen. Step 18: Compare your sample input and output to the following: How many ages do you want to enter: 6 Enter an age: 13 Enter an age: 43 Enter an age: 25 Enter an age: 34 Enter an age: 28 Enter an age: 43 The average age is 31 >>> Step 18: Execute your program so that all loops work and paste the final code below
Step 1: Start the IDLE Environment for Python. Prior to entering code, save your file by clicking on File and then Save. Select your location and save this file as Lab5-3.py. Be sure to include the .py extension.
Step 2: Document the first few lines of your program to include your name, the date, and a brief description of what the program does.
Step 3: Start your program with the following code for main:
# Lab 5-3 Practicing for loops
# A Basic For loop
# The Second Counter code
# The Accumulator code
# The Average Age code
Step 4: Under the documentation for A Basic For Loop, add the following lines of code:
print('I will display the numbers 1 through 5.')
for num in [1, 2, 3, 4, 5]:
print num
On the first iteration, 1 is placed into the variable num and num is then printed to the screen. The process is continued as follows:
Execute your program. Notice that the output is as follows:
>>>
I will display the numbers 1 through 5.
1
2
3
4
5
>>>
Step 5: The next loop to code is the Second Counter code. This loop can be processed in the same way as Step 4; however, it would take a long time to write 1 through 60 in the for loop definition. Therefore, the range function should be used to simplify the process. Write a for loop that has a range from 1 to 61. If you stop at 60, only 59 seconds will be printed. If you only provide one argument, the starting value will be 0. (Reference the Critical Review section above for the exact syntax.)
Step 6: The next loop to code is the Accumulator code. Start by initializing a total variable to 0. This must be done in order to accumulate values.
Step 7: The next step is to write a for loop that iterates 5 times. The easiest way to do this is the following.
for counter in range(5):
Step 8: Inside the for loop, allow the user to enter a number. Then, add an accumulation statement that adds the number to total. In Python, the range function determines the number of iterations, so it is not necessary to manually increment counter.
Step 9: Outside of the for loop, use a print statement that will display the total.
Step 10: Compare your sample input and output to the following:
Enter a number: 54
Enter a number: 32
Enter a number: 231
Enter a number: 23
Enter a number: 87
The total is 427
Step 11: The final loop to code is the Average Age code. Start by initializing totalAge and averageAge to 0. (Reference the Critical Review section above on Letting the User Control the Number of Iterations).
Step 12: The next step is to ask how many ages they want to enter. Store the answer in the number variable.
Step 13: Write the definition for the for loop using the range function such as:
for counter in range(0, number):
Step 14: Inside the for loop, allow the user to enter an age.
Step 15: Inside the for loop, add the code that will accumulate age into the totalAge variable.
Step 16: Outside of the loop, calculate the average age as averageAge = totalAge / number.
Step 17: Outside of the loop, display the averageAge variable to the screen.
Step 18: Compare your sample input and output to the following:
How many ages do you want to enter: 6
Enter an age: 13
Enter an age: 43
Enter an age: 25
Enter an age: 34
Enter an age: 28
Enter an age: 43
The average age is 31
>>>
Step 18: Execute your program so that all loops work and paste the final code below

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

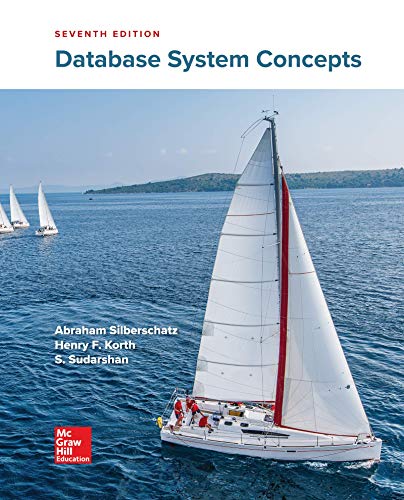
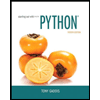
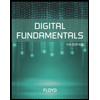
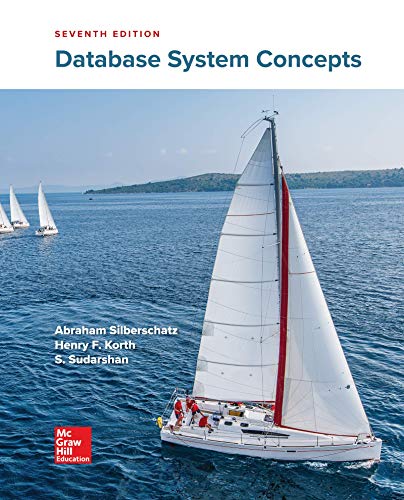
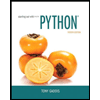
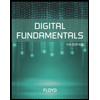
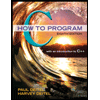
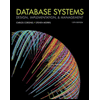
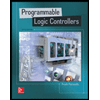