Student Final Grades Write a function called student_averages () that takes the list of lists of grades as a parameter and com- putes a final grade for each student. The grading scheme for the class is: • 10% for each assignment (recall there were 4 assignments) • 20% for the midterm exam • 40% for the final exam The final grade for each student must be an integer, rounded appropriately (you can use Python's round () function for this). The final grades should be placed into a single list and this function must return that list. Class Average Write a function called average () that takes a list of integers representing the grade of each student in the class as a parameter, and returns the class average as a single, floating point number. Program Output At the very bottom of your program, call all of the functions you made above, using their return values appropriately, to print out the list containing each student's final grade, as well as the overall class average.
Student Final Grades Write a function called student_averages () that takes the list of lists of grades as a parameter and com- putes a final grade for each student. The grading scheme for the class is: • 10% for each assignment (recall there were 4 assignments) • 20% for the midterm exam • 40% for the final exam The final grade for each student must be an integer, rounded appropriately (you can use Python's round () function for this). The final grades should be placed into a single list and this function must return that list. Class Average Write a function called average () that takes a list of integers representing the grade of each student in the class as a parameter, and returns the class average as a single, floating point number. Program Output At the very bottom of your program, call all of the functions you made above, using their return values appropriately, to print out the list containing each student's final grade, as well as the overall class average.
Chapter8: Arrays
Section: Chapter Questions
Problem 9PE
Related questions
Question
Attached File: a2provided:
grades = [
[71, 14, 41, 87, 49, 93],
[66, 73, 54, 55, 94, 79],
[20, 51, 77, 20, 86, 15],
[79, 63, 72, 57, 63, 73],
[30, 66, 78, 65, 41, 45],
[11, 83, 51, 47, 68, 63],
[99, 43, 85, 86, 24, 75],
[67, 102, 11, 84, 64, 109],
[16, 24, 101, 78, 55, 89],
[100, 26, 37, 95, 106, 100],
[55, 77, 30, 34, 28, 15],
[87, 67, 13, 71, 67, 83],
[95, 65, 94, 56, 15, 92],
[102, 23, 36, 39, 60, 39],
[90, 59, 96, 105, 83, 16],
[101, 40, 17, 12, 44, 36],
[39, 63, 96, 12, 65, 82],
[95, 20, 105, 34, 69, 95],
[95, 93, 75, 18, 105, 102],
[35, 15, 82, 106, 73, 30],
[80, 52, 67, 49, 11, 88],
[86, 17, 44, 75, 78, 49],
[22, 60, 74, 110, 92, 37],
[13, 14, 15, 82, 75, 57],
[71, 106, 15, 77, 30, 98],
[43, 80, 76, 85, 102, 53],
[26, 98, 60, 80, 104, 79],
[12, 28, 40, 106, 88, 84],
[82, 75, 101, 29, 51, 75],
[20, 31, 84, 35, 19, 85],
[84, 63, 56, 101, 83, 102],
[25, 106, 36, 24, 61, 80],
[86, 75, 89, 47, 28, 27],
[38, 48, 22, 72, 53, 83],
[70, 34, 46, 86, 32, 58],
[83, 59, 38, 16, 94, 104],
[62, 110, 13, 12, 13, 83],
[90, 59, 55, 19, 69, 34],
[73, 102, 60, 49, 67, 109],
[94, 63, 100, 29, 64, 30],
[105, 19, 12, 65, 65, 47],
[10, 21, 72, 82, 104, 64],
[39, 107, 107, 108, 63, 80],
[52, 76, 77, 16, 49, 80],
[35, 30, 58, 36, 52, 51],
[102, 100, 22, 78, 57, 107],
[63, 11, 69, 61, 71, 95],
[76, 80, 83, 72, 79, 55],
[104, 14, 14, 92, 17, 89],
[46, 68, 108, 45, 23, 97]
]
[71, 14, 41, 87, 49, 93],
[66, 73, 54, 55, 94, 79],
[20, 51, 77, 20, 86, 15],
[79, 63, 72, 57, 63, 73],
[30, 66, 78, 65, 41, 45],
[11, 83, 51, 47, 68, 63],
[99, 43, 85, 86, 24, 75],
[67, 102, 11, 84, 64, 109],
[16, 24, 101, 78, 55, 89],
[100, 26, 37, 95, 106, 100],
[55, 77, 30, 34, 28, 15],
[87, 67, 13, 71, 67, 83],
[95, 65, 94, 56, 15, 92],
[102, 23, 36, 39, 60, 39],
[90, 59, 96, 105, 83, 16],
[101, 40, 17, 12, 44, 36],
[39, 63, 96, 12, 65, 82],
[95, 20, 105, 34, 69, 95],
[95, 93, 75, 18, 105, 102],
[35, 15, 82, 106, 73, 30],
[80, 52, 67, 49, 11, 88],
[86, 17, 44, 75, 78, 49],
[22, 60, 74, 110, 92, 37],
[13, 14, 15, 82, 75, 57],
[71, 106, 15, 77, 30, 98],
[43, 80, 76, 85, 102, 53],
[26, 98, 60, 80, 104, 79],
[12, 28, 40, 106, 88, 84],
[82, 75, 101, 29, 51, 75],
[20, 31, 84, 35, 19, 85],
[84, 63, 56, 101, 83, 102],
[25, 106, 36, 24, 61, 80],
[86, 75, 89, 47, 28, 27],
[38, 48, 22, 72, 53, 83],
[70, 34, 46, 86, 32, 58],
[83, 59, 38, 16, 94, 104],
[62, 110, 13, 12, 13, 83],
[90, 59, 55, 19, 69, 34],
[73, 102, 60, 49, 67, 109],
[94, 63, 100, 29, 64, 30],
[105, 19, 12, 65, 65, 47],
[10, 21, 72, 82, 104, 64],
[39, 107, 107, 108, 63, 80],
[52, 76, 77, 16, 49, 80],
[35, 30, 58, 36, 52, 51],
[102, 100, 22, 78, 57, 107],
[63, 11, 69, 61, 71, 95],
[76, 80, 83, 72, 79, 55],
[104, 14, 14, 92, 17, 89],
[46, 68, 108, 45, 23, 97]
]
(This question is to learn/practice)

Transcribed Image Text:Student Final Grades
Write a function called student_averages () that takes the list of lists of grades as a parameter and com-
putes a final grade for each student. The grading scheme for the class is:
• 10% for each assignment (recall there were 4 assignments)
• 20% for the midterm exam
• 40% for the final exam
The final grade for each student must be an integer, rounded appropriately (you can use Python's round ()
function for this). The final grades should be placed into a single list and this function must return that list.
Class Average
Write a function called average () that takes a list of integers representing the grade of each student in the
class as a parameter, and returns the class average as a single, floating point number.
Program Output
At the very bottom of your program, call all of the functions you made above, using their return values
appropriately, to print out the list containing each student's final grade, as well as the overall class average.

Transcribed Image Text:Professor Oak is in trouble: his class grades are a mess! His midterm exam was too hard, and he gave
out too many bonus marks to certain students. For this question, you will write a program to clean up the
grades and calculate the final grade for each student in the class.
Starter File
You have been provided a starter file that contains Professor Oak's student grades as a list of lists. Each
sublist has exactly 6 items, representing the grades for (in order) four assignments, a midterm exam, and
a final exam. All grades are out of 100. Just copy/paste this list to the top of your program. But keep in
mind that your code should work even if we use a different starting list (though with the same format) to
test your program.
From here, you will write three separate functions to perform three tasks, as described below.
Cleaning the Data
Write a function called clean_grades () that takes the list of lists of grades as a parameter. For all of the
student grades, this function should perform two tasks:
• Increase the midterm exam grade for ALL students by 2. Recall that the midterm grade is the 5th item
in each sublist.
• For any grade greater than 100. set the grade to be just 100 (make sure to check for this AFTER
increasing the grade for the midterm exam!)
These changes should be done by modifying the input list itself. No new lists should be created and this
function has no return value.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
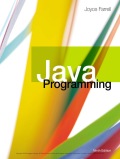
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
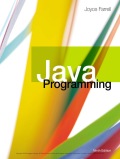
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT