The Fast Freight Shipping Company charges the following rates: Weight of Package (in Kilograms) 2 kg or less Over 2 kg but not more than 6 kg Over 6 kg but not more than 10 kg Over 10 kg but not more than 20 kg Rate per 500 Miles Shipped $1.10 $2.20 $3.70 $4.80 Write a program that asks for the weight of the package and the distance it is to be shipped, and then displays the charges. Input Validation: Do not accept values of 0 or less for the weight of the package. Do not accept weights of more than 20 kg (this is the maximum weight the company will ship). Do not accept distances of less than 10 miles or more than 3,000 miles. These are the company's minimum and maximum shipping distances.
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
I have to do this exercise in JAVA, I already have most of the code but I don't know how to calculate the distance, for every 500 miles an amount is charged, so for example if the user chooses 520 miles it would be rounded to 1000 miles and the price would be double. So for a 6kg package the price is $2.20 but the package will be ship 520 miles so the price would be $4.40.
import java.util.Scanner;
class Main {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
int weight, miles;
double r;
/* Input - weight */
System.out.print("What is the weight of the package: ");
weight = input.nextInt();
/* Input validation for the weight */
while (weight < 0 || weight > 20){
System.out.print("invalid imput, plis input the correct weight: ");
weight = input.nextInt();
}
/* Input - distance */
System.out.print("What is the distance: ");
miles = input.nextInt();
/* Input validation for the distance */
while (miles < 10 || miles > 3000){
System.out.print("invalid imput, plis input the correct distance: ");
miles = input.nextInt();
}
if (weight <= 2){
r = 1.10;
System.out.print(" You have to pay: $" + r + " for you package");
}
else if (weight > 2 && weight <= 6 ){
r = 2.20;
System.out.print(" You have to pay: $" + r + " for you package");
}
else if (weight > 6 && weight <= 10 ){
r = 3.70;
System.out.print(" You have to pay: $" + r + " for you package");
}
else if (weight > 10 && weight <= 20 ){
r = 4.80;
System.out.print(" You have to pay: $" + r + " for you package");
}
}
}

![19 2012345678290123455劲8羽♀钍2钌455钉8p丽弘貂貉科5%刃890碰成
import java.util.Scanner;
21 class Main {
22 - public static void main(String[] args) {
Scanner input = new Scanner(System.in);
int weight, miles;
double r;
45
32 while (weight < 0 || weight > 20){
49
9
53 -
57 ~
| || /* Input weight */
System.out.print("What is the weight of the package: ");
weight = input.nextInt();
41 ~ while (miles < 10 || miles > 3000){
}
/* Input validation for the weight */
System.out.print("invalid imput, plis input the correct weight: ");
weight = input.nextInt();
/* Input distance */
System.out.print("What is the distance: ");
miles = input.nextInt();
}
/* Input validation for the distance */
System.out.print("invalid imput, plis input the correct distance: ");
miles = input.nextInt ();
}
if (weight <= 2){
r = 1.10;
System.out.print(" You have to pay: $" + r + for you package");
else if (weight > 2 && weight <= 6 ){
r = 2.20;
System.out.print(" You have to pay:
+r+ " for you package");
}
else if (weight > 6 && weight <= 10 ){
r = 3.70;
System.out.print(" You have to pay: $" + r +
}
else if (weight > 10 && weight <= 20 ) {
r = 4.80;
System.out.print(" You have to pay: $" + r +
for you package");
for you package");](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe0bef57a-e69b-4f41-b2ad-c14f6997454b%2F7f389032-ea47-4244-b633-206a1e930a69%2Fa1icdd_processed.png&w=3840&q=75)

Here is the java program for the above requirements:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
double weight, distance, rate;
// Ask for weight of the package
System.out.print("Enter the weight of the package (in kilograms): ");
weight = input.nextDouble();
// Input validation for weight
while (weight <= 0 || weight > 20) {
System.out.print("Invalid weight. Enter a weight between 0 and 20 kilograms: ");
weight = input.nextDouble();
}
// Ask for distance
System.out.print("Enter the distance to be shipped (in miles): ");
distance = input.nextDouble();
// Input validation for distance
while (distance < 10 || distance > 3000) {
System.out.print("Invalid distance. Enter a distance between 10 and 3000 miles: ");
distance = input.nextDouble();
}
// Round up distance to nearest 500
int dist =(int) Math.ceil(distance/500);
// Calculate rate based on weight of package
if (weight <= 2) {
rate = 1.10;
} else if (weight <= 6) {
rate = 2.20;
} else if (weight <= 10) {
rate = 3.70;
} else {
rate = 4.80;
}
// Display charges
System.out.println("The charges for shipping a package weighing " + weight + " kilograms for " + distance + " miles is $" + dist * rate);
}
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

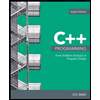
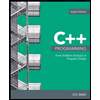