The function below takes an array of User objects and returns the User object that has taken the most steps. The body of the function first declares a variable that is an optional User, then loops through all of the users in the array. Inside each iteration of the loop, it will check if topCompetitor has a value or not by unwrapping it. If topCompetitor doesn't have a value, then the current user in the iteration is assumed to have the highest score and is assigned to topCompetitor. If topCompetitor has a value, there is code to check whether the current user in the iteration has taken more steps than the user that is assigned to topCompetitor. At that point, the goal is to assign the user with the higher score to topCompetitor. However, the code generates a compiler error because, due to improper variable shadowing, topCompetitor has a narrower scope than it should if it is going to be reassigned. Fix the compiler error below and call getWinner(competitors:), passing in the array competitors. Print the name property of the returned User object. You'll know that you fixed the function properly if the user returned is activeSitter. func getwinner(competitors: [User]) -> User? { var topCompetitor: User? for competitor in competitors { if let topCompetitor = topCompetitor { if competitor.stepsToday > topCompetitor.stepsToday { topCompetitor = competitor Cannot assign to value: 'topCompetitor' is a 'let' constant } else { topCompetitor = competitor return topCompetitor
The function below takes an array of User objects and returns the User object that has taken the most steps. The body of the function first declares a variable that is an optional User, then loops through all of the users in the array. Inside each iteration of the loop, it will check if topCompetitor has a value or not by unwrapping it. If topCompetitor doesn't have a value, then the current user in the iteration is assumed to have the highest score and is assigned to topCompetitor. If topCompetitor has a value, there is code to check whether the current user in the iteration has taken more steps than the user that is assigned to topCompetitor. At that point, the goal is to assign the user with the higher score to topCompetitor. However, the code generates a compiler error because, due to improper variable shadowing, topCompetitor has a narrower scope than it should if it is going to be reassigned. Fix the compiler error below and call getWinner(competitors:), passing in the array competitors. Print the name property of the returned User object. You'll know that you fixed the function properly if the user returned is activeSitter. func getwinner(competitors: [User]) -> User? { var topCompetitor: User? for competitor in competitors { if let topCompetitor = topCompetitor { if competitor.stepsToday > topCompetitor.stepsToday { topCompetitor = competitor Cannot assign to value: 'topCompetitor' is a 'let' constant } else { topCompetitor = competitor return topCompetitor
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
How do I fix this compiler error using Swift code?
![The function below takes an array of User objects and returns the User object that has taken the most steps. The body of the function first declares a variable that is an optional User, then
loops through all of the users in the array. Inside each iteration of the loop, it will check if topCompetitor has a value or not by unwrapping it. If topCompetitor doesn't have a value,
then the current user in the iteration is assumed to have the highest score and is assigned to topCompetitor. If topCompetitor has a value, there is code to check whether the current
user in the iteration has taken more steps than the user that is assigned to topCompetitor.
At that point, the goal is to assign the user with the higher score to topCompetitor. However, the code generates a compiler error because, due to improper variable shadowing,
topCompetitor has a narrower scope than it should if it is going to be reassigned. Fix the compiler error below and call getWinner(competitors:), passing in the array
competitors. Print the name property of the returned User object. You'll know that you fixed the function properly if the user returned is activeSitter.
func getWinner(competitors: [User]) -> User? {
var topCompetitor: User?
for competitor in competitors {
if let topCompetitor
topCompetitor {
if competitor.stepsToday > topCompetitor.stepsToday {
= competitor
%3D
topCompetitor
Cannot assign to value: 'topCompetitor' is a 'let' constant
}
} else {
topCompetitor = competitor
}
}
return topCompetitor
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fbea0901b-9b71-43dc-90d5-d9e10ed3de89%2F7795fb3c-5365-4c89-bcc0-f693d391f7a8%2Fzhd0mqd_processed.png&w=3840&q=75)
Transcribed Image Text:The function below takes an array of User objects and returns the User object that has taken the most steps. The body of the function first declares a variable that is an optional User, then
loops through all of the users in the array. Inside each iteration of the loop, it will check if topCompetitor has a value or not by unwrapping it. If topCompetitor doesn't have a value,
then the current user in the iteration is assumed to have the highest score and is assigned to topCompetitor. If topCompetitor has a value, there is code to check whether the current
user in the iteration has taken more steps than the user that is assigned to topCompetitor.
At that point, the goal is to assign the user with the higher score to topCompetitor. However, the code generates a compiler error because, due to improper variable shadowing,
topCompetitor has a narrower scope than it should if it is going to be reassigned. Fix the compiler error below and call getWinner(competitors:), passing in the array
competitors. Print the name property of the returned User object. You'll know that you fixed the function properly if the user returned is activeSitter.
func getWinner(competitors: [User]) -> User? {
var topCompetitor: User?
for competitor in competitors {
if let topCompetitor
topCompetitor {
if competitor.stepsToday > topCompetitor.stepsToday {
= competitor
%3D
topCompetitor
Cannot assign to value: 'topCompetitor' is a 'let' constant
}
} else {
topCompetitor = competitor
}
}
return topCompetitor
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

Recommended textbooks for you
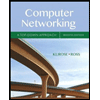
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
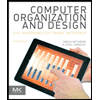
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
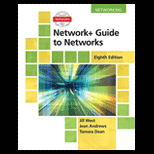
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
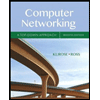
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
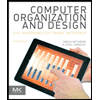
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
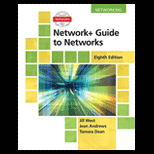
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
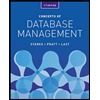
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
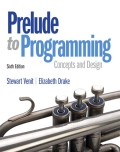
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
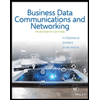
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY