The purpose of this assignment is to practice OOP with Decisions, Loops, Arrays and ArrayLists, Input/Output Files, Class design, Interfaces, Polymorphism and Exception Handling. Create a NetBeans project named HW3 Yourld. Develop classes for the required solutions. Impa ant: Apply good programming practices: Provide API documentation comments for your class(s), class constructor(s) and method(s) using the Java standard form for documentation comments discussed in this course. Use meaningful variable and constant names. Show your name, university id and section number as a comment at the start of each class. Submit to Moodle the compressed file of your project. Problem: In this assignment, you should study and program an application for drawing graphic objects using object- oriented programming concepts. There are several graphic objects that can be drawn however for the purpose of this project your application will be able to draw only three types: lines, rectangles and parallelograms. Write a Java application that draws different graphic objects on a single canvas. Your application should be able to draw lines, rectangles and parallelograms. The application should allow the user to move these objects to
The purpose of this assignment is to practice OOP with Decisions, Loops, Arrays and ArrayLists, Input/Output Files, Class design, Interfaces, Polymorphism and Exception Handling. Create a NetBeans project named HW3 Yourld. Develop classes for the required solutions. Impa ant: Apply good programming practices: Provide API documentation comments for your class(s), class constructor(s) and method(s) using the Java standard form for documentation comments discussed in this course. Use meaningful variable and constant names. Show your name, university id and section number as a comment at the start of each class. Submit to Moodle the compressed file of your project. Problem: In this assignment, you should study and program an application for drawing graphic objects using object- oriented programming concepts. There are several graphic objects that can be drawn however for the purpose of this project your application will be able to draw only three types: lines, rectangles and parallelograms. Write a Java application that draws different graphic objects on a single canvas. Your application should be able to draw lines, rectangles and parallelograms. The application should allow the user to move these objects to
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Solve this problem

Transcribed Image Text:1/ 2
The purpose of this assignment is to practice 0OP with Decisions, Loops, Arrays and ArrayLists, Input/Output
Files, Class design, Interfaces, Polymorphism and Exception Handling. Create a NetBeans project named
HW3 Yourld. Develop classes for the required solutions.
Impa tant: Apply good programming practices:
"Provide API documentation comments for your class(s), class constructor(s) and method(s) using the
Java standard form for documentation comments discussed in this course.
Use meaningful variable and constant names.
Show your name, university id and section number as a comment at the start of each class.
Submit to Moodle the compressed file of your project.
Problem:
In this assignment, you should study and program an application for drawing graphic objects using object-
oriented programming concepts. There are several graphic objects that can be drawn however for the purpose
of this project your application will be able to draw only three types: lines, rectangles and parallelograms.
Write a Java application that draws different graphic objects on a single canvas. Your application should be able
to draw lines, rectangles and parallelograms. The application should allow the user to move these objects to
specific location (x, y), and fill the objects if needed. In this assignment you should not use any of the graphics
packages provided by java, instead you should create your own drawings by using the System.out printing
feature.
Specifications:
Each graphic object is drawn in a specific location (x, y) (the left top most corner of the object).
A line object has a length and can be moved, and drawn.
A rectangle object has width and height and can be moved, drawn and filled or not with a specific
character as shown in the Figure of the sample run.
A parallelogram also has a defined base and height and can be moved, drawn, and filled or not with a
specific character.
All your drawing must be on one canvas. You can assume that a canvas is a two-dimensional array of
characters, representing the pixels of the canvas, and you just need to fill the cells of this matrix as
needed by each created object. For instance, for a canvas of size 15 x 20, the result of the pseudocode
for Figure 1 will produce the following content in the canvas array.
For example, the following pseudocode will create a parallelogram of base 8 and height 6, then it will be moved
to position (5, 2), filled with "+", and then it will be drawn on the canvas. After that, a line object of length 15
is drawn starting at (2, 4). The output of the pseudocode is shown in Figure 1.
R.
38

Transcribed Image Text:2/2
parallelogram = new Parallelogram (8, 6)
parallelogram.moveTo (5,2).
parallelogram.setFilled (true)
parallelogram.setFillingSymbol ('+')
parallelogram.draw(canvas)
++++++++
++++++++
++++++++
++++++++
line = new Line (2, 4, 15)
line.draw (canvas)
++++++
show (canvas)
Figure 1:Output of the pseudocode example
Your program should read the specifications of graphic objects from a text File provided by the user using the
command line. Each graphic object starts with a letter indicating the type of the object (L: line, R: rectangle,
or P: parallelogram), followed by the properties of the object (e.g. position (optional), length of a line, width
and length for a rectangle, or base and height for a parallelogram). The next lines will define any operations
applied to that object (e.g. moveTo 2 5, filled yes, filling +, draw). Each object description is terminated by a
dashed line as shown in the sample below (Figure 2).
Your program should read the details of each object, apply the requested operations, handle any exceptions
might be raised, and finally show the entire canvas that has all the drawn objects.
objects.txt
L 15
moveTo 2 4
draw
moveTo 5 2
R 20 3 5 5
filled yes
filling +
draw
P 10 6
draw
moveTo 24 11
draw
P 15 15 5 3
filled yes
draw
R 74
filled no
moveTo 5 10
draw
L327
Figure 2: Sample Input file and the drawing corresponding to its content.
Grading Table:
38
DELL
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
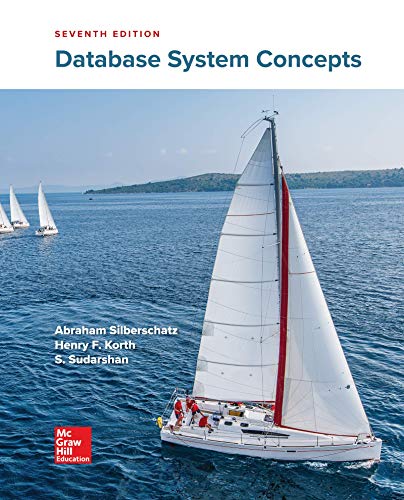
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
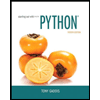
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
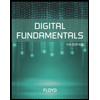
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
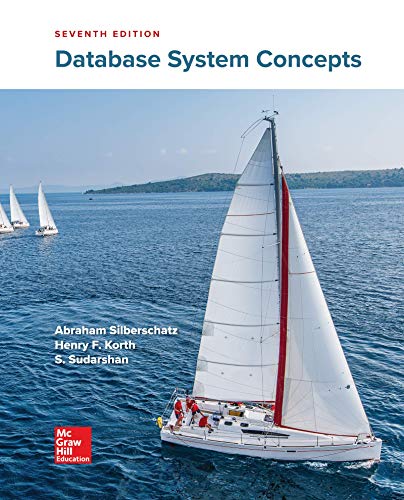
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
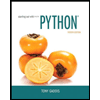
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
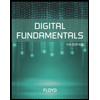
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
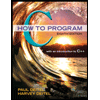
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
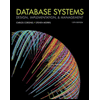
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
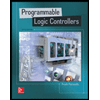
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education