There is a code below this template. The template must be implemented into the code but I am unable to run the code whenever I try to implement the code. How do I inmplement the template into the code? // -- brief statement as to the file’s purpose //XXXX-XXX- ADD YOUR SECTION NUMBER // //Include statements #include #include using namespace std; //Global declarations: Constants and type definitions only -- no variables //Function prototypes int main() { //In cout statement below SUBSTITUTE your name and lab number cout << "Your name -- Lab Number" << endl << endl; //Variable declarations //Program logic //Closing program statements system("pause"); return 0; } //Function definitions Code: #include // Defining a header file #include // Defining a header file using namespace std; //Enum planetType enum planetType { Mercury, Venus, Earth, Moon, Mars, Jupiter, Saturn, Uranus, Neptune, Pluto }; // Including inputs from users void GetUserInput(float &w, string &p){ // User Weight Input cout << "Please enter your weight: " << endl;// print message cin >> w; // Input user to a world cout << "Please enter planet name [Mercury, Venus, Earth, Moon, Mars, Jupiter, Saturn, Uranus, Neptune, Pluto] : " << endl; // print the planet name cin >> p; } // InputToPlanet typeConvertInput planetType ConvertInputToPlanetType(string p){ planetType s; if(p.compare("Mercury") == 0 || p.compare("mercury") == 0){ s = Mercury; } if(p.compare("Venus") == 0 || p.compare("venus") == 0){ s = Venus; } if(p.compare("Earth") == 0 || p.compare("earth") == 0){ s = Earth; } if(p.compare("Moon") == 0 || p.compare("moon") == 0){ s = Moon; } if(p.compare("Mars") == 0 || p.compare("mars") == 0){ s = Mars; } if(p.compare("Jupiter") == 0 || p.compare("jupiter") == 0){ s = Jupiter; } if(p.compare("Saturn") == 0 || p.compare("saturn") == 0){ s = Saturn; } if(p.compare("Uranus") == 0 || p.compare("uranus") == 0){ s = Uranus; } if(p.compare("Neptune") == 0 || p.compare("neptune") == 0){ s = Neptune; } if(p.compare("Pluto") == 0 || p.compare("pluto") == 0){ s = Pluto; } return s; } // Weight of Output void OutputWeight(planetType s, float w){ switch(s) // using switch case { case Mercury : cout << " Your weight will be on planet Mercury" << (w * 0.4155); // print the message break; case Venus : cout << " Your weight will be on planet Venus" << (w * 0.8975); //print the message break; case Earth : cout << " Your weight will be on planet Earth " << (w * 1.0);//print the message break; case Moon : cout << " Your weight will be on planet Moon" << (w * 0.166);// print the message break; case Mars : cout << " Your weight will be on planet Mars" << (w * 0.3507);// print the message break; case Jupiter : cout << " Your weight will be on planet Jupiter" << (w * 2.5374);// print the message break; case Saturn : cout << " Your weight will be on planet Saturn" << (w * 1.067); //print the message break; case Uranus : cout << " Your weight will be on planet Uranus " << (w * 0.8947);// print the message break; case Neptune : cout << " Your weight will be on planet Neptune " << (w * 1.1794);// print the message break; case Pluto : cout << " Your weight will be on planet Pluto" << (w * 0.0899); // print the message } } int main() //defining a main function { float w; //declaring float variable string p; // declaring string variable planetType s; // Including inputs from users GetUserInput(w, p); // Check the input is invalid if(!(p.compare("Mercury") == 0 || p.compare("mercury") == 0 || p.compare("Venus") == 0 || p.compare("venus") == 0 || p.compare("Earth") == 0 || p.compare("earth") == 0 || p.compare("Moon") == 0 || p.compare("moon") == 0 || p.compare("Mars") == 0 || p.compare("mars") == 0 || p.compare("Jupiter") == 0 || p.compare("jupiter") == 0 || p.compare("Saturn") == 0 || p.compare("saturn") == 0 || p.compare("Uranus") == 0 || p.compare("uranus") == 0 || p.compare("Neptune") == 0 || p.compare("neptune") == 0 || p.compare("Pluto") == 0 || p.compare("pluto") == 0)){ cout << " Error: one planet's name should be [Mercury, Venus, Earth, Moon, Mars, Jupiter, Saturn, Uranus, Neptune, Pluto] : " << endl; // print the message } else{ // TypeConvertInputToPlanet s = ConvertInputToPlanetType(p); // Output of the Weight OutputWeight(s, w); } return 0; }
There is a code below this template. The template must be implemented into the code but I am unable to run the code whenever I try to implement the code. How do I inmplement the template into the code?
// -- brief statement as to the file’s purpose
//XXXX-XXX- ADD YOUR SECTION NUMBER
// //Include statements
#include <iostream>
#include <string>
using namespace std;
//Global declarations: Constants and type definitions only -- no variables
//Function prototypes
int main()
{
//In cout statement below SUBSTITUTE your name and lab number
cout << "Your name -- Lab Number" << endl << endl;
//Variable declarations
//Program logic
//Closing program statements
system("pause");
return 0;
}
//Function definitions
Code:
#include <iostream> // Defining a header file
#include <string> // Defining a header file
using namespace std;
//Enum planetType
enum planetType {
Mercury, Venus,
Earth, Moon,
Mars, Jupiter,
Saturn, Uranus,
Neptune, Pluto };
// Including inputs from users
void GetUserInput(float &w, string &p){
// User Weight Input
cout << "Please enter your weight: " << endl;// print message
cin >> w;
// Input user to a world
cout << "Please enter planet name [Mercury, Venus, Earth, Moon, Mars, Jupiter, Saturn, Uranus, Neptune, Pluto] : " << endl; // print the planet name
cin >> p;
}
// InputToPlanet typeConvertInput
planetType ConvertInputToPlanetType(string p){
planetType s;
if(p.compare("Mercury") == 0 || p.compare("mercury") == 0){
s = Mercury;
}
if(p.compare("Venus") == 0 || p.compare("venus") == 0){
s = Venus;
}
if(p.compare("Earth") == 0 || p.compare("earth") == 0){
s = Earth;
}
if(p.compare("Moon") == 0 || p.compare("moon") == 0){
s = Moon;
}
if(p.compare("Mars") == 0 || p.compare("mars") == 0){
s = Mars;
}
if(p.compare("Jupiter") == 0 || p.compare("jupiter") == 0){
s = Jupiter;
}
if(p.compare("Saturn") == 0 || p.compare("saturn") == 0){
s = Saturn;
}
if(p.compare("Uranus") == 0 || p.compare("uranus") == 0){
s = Uranus;
}
if(p.compare("Neptune") == 0 || p.compare("neptune") == 0){
s = Neptune;
}
if(p.compare("Pluto") == 0 || p.compare("pluto") == 0){
s = Pluto;
}
return s;
}
// Weight of Output
void OutputWeight(planetType s, float w){
switch(s) // using switch case
{
case Mercury :
cout << " Your weight will be on planet Mercury" << (w * 0.4155); // print the message
break;
case Venus :
cout << " Your weight will be on planet Venus" << (w * 0.8975); //print the message
break;
case Earth :
cout << " Your weight will be on planet Earth " << (w * 1.0);//print the message
break;
case Moon :
cout << " Your weight will be on planet Moon" << (w * 0.166);// print the message
break;
case Mars :
cout << " Your weight will be on planet Mars" << (w * 0.3507);// print the message
break;
case Jupiter :
cout << " Your weight will be on planet Jupiter" << (w * 2.5374);// print the message
break;
case Saturn :
cout << " Your weight will be on planet Saturn" << (w * 1.067); //print the message
break;
case Uranus :
cout << " Your weight will be on planet Uranus " << (w * 0.8947);// print the message
break;
case Neptune :
cout << " Your weight will be on planet Neptune " << (w * 1.1794);// print the message
break;
case Pluto :
cout << " Your weight will be on planet Pluto" << (w * 0.0899); // print the message
}
}
int main() //defining a main function
{
float w; //declaring float variable
string p; // declaring string variable
planetType s;
// Including inputs from users
GetUserInput(w, p);
// Check the input is invalid
if(!(p.compare("Mercury") == 0 || p.compare("mercury") == 0 ||
p.compare("Venus") == 0 || p.compare("venus") == 0 ||
p.compare("Earth") == 0 || p.compare("earth") == 0 ||
p.compare("Moon") == 0 || p.compare("moon") == 0 ||
p.compare("Mars") == 0 || p.compare("mars") == 0 ||
p.compare("Jupiter") == 0 || p.compare("jupiter") == 0 ||
p.compare("Saturn") == 0 || p.compare("saturn") == 0 ||
p.compare("Uranus") == 0 || p.compare("uranus") == 0 ||
p.compare("Neptune") == 0 || p.compare("neptune") == 0 ||
p.compare("Pluto") == 0 || p.compare("pluto") == 0)){
cout << " Error: one planet's name should be [Mercury, Venus, Earth, Moon, Mars, Jupiter, Saturn, Uranus, Neptune, Pluto] : " << endl; // print the message
}
else{
// TypeConvertInputToPlanet
s = ConvertInputToPlanetType(p);
// Output of the Weight
OutputWeight(s, w);
}
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

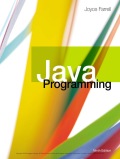
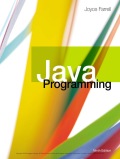