This discussion assignment examines variable scope. For this assignment, we will be examining the Beverage2 class (an updated version of the Beverage class from discussion 1), its subclass Espresso, and the TestBeverage2 class. This time, assume that all source files are in the same package. Note that some lines may wrap, but are still considered on one line. Examine the code below. Recall that variables have four kinds of scope: static, instance, local, and block. For
This discussion assignment examines variable scope. For this assignment, we will be examining the Beverage2 class (an updated version of the Beverage class from discussion 1), its subclass Espresso, and the TestBeverage2 class. This time, assume that all source files are in the same package. Note that some lines may wrap, but are still considered on one line.
Examine the code below. Recall that variables have four kinds of scope: static, instance, local, and block. For each variable listed below, identify its scope (static, instance, local, or block) and describe the scope using complete sentences. An example description is provided, after the code listing, for the ounces variable in the Beverage2 class.
Please describe the scope for the following variables:
- The num variable in the Beverage2 class
- The price variable in the Beverage2 class
- The shots variable in the Espresso class
- The b variable in the TestBeverage2 class
- The j variable in the TestBeverage2 class
public abstract class Beverage2 {
private static int num;
private int ounces;
private double price;
protected Beverage2(int ounces, double price) {
this.ounces = ounces;
this.price = price;
num++;
}
public int getOunces() { return ounces; }
public double getPrice() { return price; }
public static int getNum() { return num; }
public abstract String drink();
}
public class Espresso extends Beverage2 {
private int shots;
protected Espresso (int ounces, double price, int shots) {
super(ounces, price);
this.shots = shots;
}
public int getShots() { return shots; }
public String drink() {
return "The espresso is strong.";
}
}
public class TestBeverage2 {
public static void main(String[] args) {
Espresso b;
for (int j = 0; j < 3; j++) {
b = new Espresso(2, 2.49, j + 1);
System.out.print("Drink #" + Beverage2.getNum() + ": ");
System.out.println("Espresso with " + b.getShots() + " shot(s)");
}
System.out.println("\nYou ordered " + Beverage2.getNum() + " drinks.");
}
}
Example description of scope for the ounces variable in the Beverage2 class: The ounces variable in the Beverage2 class is an instance variable of Beverage2, and therefore has instance variable scope. Whenever a Beverage2 object is instantiated, it has an ounces variable that lives as long as that Beverage2 object exists.

Step by step
Solved in 2 steps

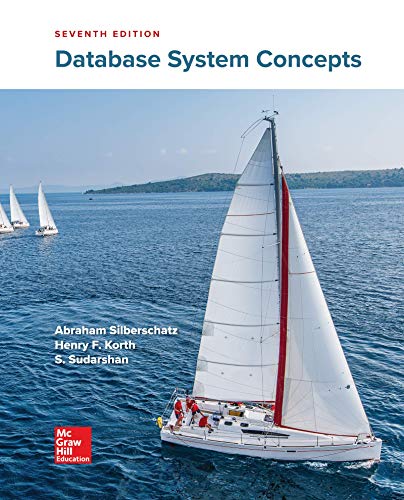
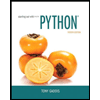
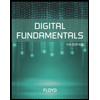
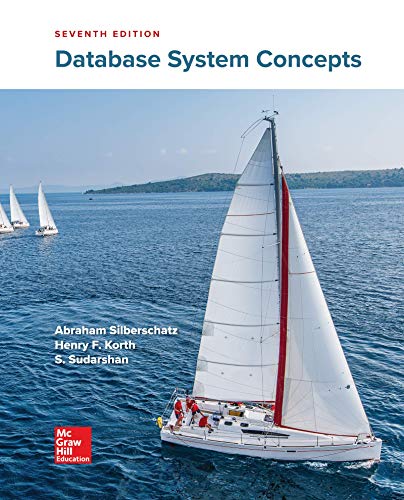
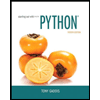
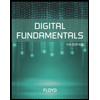
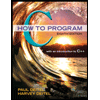
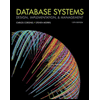
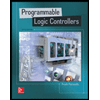