Time remaining: 00:09:10 Computer Science this file is named HW3.Java can someone run it through a GNU compiler and please show me the outputs import java.io.FileWriter; import java.io.IOException; public class HW3 { public static void main(String[] args) throws IOException { // 0th argument contains the name of algorithm String algo = args[0]; // 1st argument contains the name of file // Make a new file FileWriter fw = new FileWriter(args[1]); if (algo.equals("p1")) { // 2nd argument comes in the form of string. // convert it to intger and run a loop for (int i = 1; i <= Integer.parseInt(args[2]); i++) { double[] x = new double[(int) Math.pow(10, i)]; // use start and end for recording time long start = System.currentTimeMillis(); prefixAverage1(x); long end = System.currentTimeMillis(); double totalTime = Math.log10(end - start); fw.write(String.valueOf(totalTime)); String newLine = System.getProperty("line.separator"); fw.write(newLine); } } else if (algo.equals("p2")) { for (int i = 1; i <= Integer.parseInt(args[2]); i++) { double[] x = new double[(int) Math.pow(10, i)]; long start = System.currentTimeMillis(); prefixAverage2(x); long end = System.currentTimeMillis(); double totalTime = Math.log10(end - start); fw.write(String.valueOf(totalTime)); String newLine = System.getProperty("line.separator"); fw.write(newLine); } } else if (algo.equals("e1")) { for (int i = 1; i <= Integer.parseInt(args[2]); i++) { int[] x = new int[(int) Math.pow(10, i)]; long start = System.currentTimeMillis(); example1(x); long end = System.currentTimeMillis(); double totalTime = Math.log10(end - start); fw.write(String.valueOf(totalTime)); String newLine = System.getProperty("line.separator"); fw.write(newLine); } } else if (algo.equals("e2")) { for (int i = 1; i <= Integer.parseInt(args[2]); i++) { int[] x = new int[(int) Math.pow(10, i)]; long start = System.currentTimeMillis(); example2(x); long end = System.currentTimeMillis(); double totalTime = Math.log10(end - start); fw.write(String.valueOf(totalTime)); String newLine = System.getProperty("line.separator"); fw.write(newLine); } } else if (algo.equals("e3")) { for (int i = 1; i <= Integer.parseInt(args[2]); i++) { int[] x = new int[(int) Math.pow(10, i)]; long start = System.currentTimeMillis(); example3(x); long end = System.currentTimeMillis(); double totalTime = Math.log10(end - start); fw.write(String.valueOf(totalTime)); String newLine = System.getProperty("line.separator"); fw.write(newLine); } } else if (algo.equals("e4")) { for (int i = 1; i <= Integer.parseInt(args[2]); i++) { int[] x = new int[(int) Math.pow(10, i)]; long start = System.currentTimeMillis(); example4(x); long end = System.currentTimeMillis(); double totalTime = Math.log10(end - start); fw.write(String.valueOf(totalTime)); String newLine = System.getProperty("line.separator"); fw.write(newLine); } } else if (algo.equals("e5")) { for (int i = 1; i <= Integer.parseInt(args[2]); i++) { int[] x = new int[(int) Math.pow(10, i)]; long start = System.currentTimeMillis(); // You can take a new array here, I am just running it for x here example5(x, x); long end = System.currentTimeMillis(); double totalTime = Math.log10(end - start); fw.write(String.valueOf(totalTime)); String newLine = System.getProperty("line.separator"); fw.write(newLine); } } fw.close(); } public static double[] prefixAverage1(double[] x) { int n = x.length; double[] a = new double[n]; for (int j = 0; j < n; j++) { double total = 0; for (int i = 0; i <= j; i++) { total += x[i]; a[j] = total / (j + 1); } } return a; } public static double[] prefixAverage2(double[] x) { int n = x.length; double[] a = new double[n]; double total = 0; for (int j = 0; j < n; j++) { total += x[j]; a[j] = total / (j + 1); } return a; } public static int example1(int[] arr) { int n = arr.length, total = 0; for (int j = 0; j < n; j++) total += arr[j]; return total; } public static int example2(int[] arr) { int n = arr.length, total = 0; for (int j = 0; j < n; j += 2) total += arr[j]; return total; } public static int example3(int[] arr) { int n = arr.length, total = 0; for (int j = 0; j < n; j++) for (int k = 0; k <= j; k++) total += arr[j]; return total; } public static int example4(int[] arr) { int n = arr.length, prefix = 0, total = 0; for (int j = 0; j < n; j++) { prefix += arr[j]; total += prefix; } return total; } public static int example5(int[] first, int[] second) { int n = first.length, count = 0; for (int i = 0; i < n; i++) { int total = 0; for (int j = 0; j < n; j++) for (int k = 0; k <= j; k++) total += first[k]; if (second[i] == total) count++; } return count; } }
Computer Science
this file is named HW3.Java can someone run it through a GNU compiler and please show me the outputs
import java.io.FileWriter;
import java.io.IOException;
public class HW3 {
public static void main(String[] args) throws IOException {
// 0th argument contains the name of
String algo = args[0];
// 1st argument contains the name of file
// Make a new file
FileWriter fw = new FileWriter(args[1]);
if (algo.equals("p1")) {
// 2nd argument comes in the form of string.
// convert it to intger and run a loop
for (int i = 1; i <= Integer.parseInt(args[2]); i++) {
double[] x = new double[(int) Math.pow(10, i)];
// use start and end for recording time
long start = System.currentTimeMillis();
prefixAverage1(x);
long end = System.currentTimeMillis();
double totalTime = Math.log10(end - start);
fw.write(String.valueOf(totalTime));
String newLine = System.getProperty("line.separator");
fw.write(newLine);
}
} else if (algo.equals("p2")) {
for (int i = 1; i <= Integer.parseInt(args[2]); i++) {
double[] x = new double[(int) Math.pow(10, i)];
long start = System.currentTimeMillis();
prefixAverage2(x);
long end = System.currentTimeMillis();
double totalTime = Math.log10(end - start);
fw.write(String.valueOf(totalTime));
String newLine = System.getProperty("line.separator");
fw.write(newLine);
}
} else if (algo.equals("e1")) {
for (int i = 1; i <= Integer.parseInt(args[2]); i++) {
int[] x = new int[(int) Math.pow(10, i)];
long start = System.currentTimeMillis();
example1(x);
long end = System.currentTimeMillis();
double totalTime = Math.log10(end - start);
fw.write(String.valueOf(totalTime));
String newLine = System.getProperty("line.separator");
fw.write(newLine);
}
} else if (algo.equals("e2")) {
for (int i = 1; i <= Integer.parseInt(args[2]); i++) {
int[] x = new int[(int) Math.pow(10, i)];
long start = System.currentTimeMillis();
example2(x);
long end = System.currentTimeMillis();
double totalTime = Math.log10(end - start);
fw.write(String.valueOf(totalTime));
String newLine = System.getProperty("line.separator");
fw.write(newLine);
}
} else if (algo.equals("e3")) {
for (int i = 1; i <= Integer.parseInt(args[2]); i++) {
int[] x = new int[(int) Math.pow(10, i)];
long start = System.currentTimeMillis();
example3(x);
long end = System.currentTimeMillis();
double totalTime = Math.log10(end - start);
fw.write(String.valueOf(totalTime));
String newLine = System.getProperty("line.separator");
fw.write(newLine);
}
} else if (algo.equals("e4")) {
for (int i = 1; i <= Integer.parseInt(args[2]); i++) {
int[] x = new int[(int) Math.pow(10, i)];
long start = System.currentTimeMillis();
example4(x);
long end = System.currentTimeMillis();
double totalTime = Math.log10(end - start);
fw.write(String.valueOf(totalTime));
String newLine = System.getProperty("line.separator");
fw.write(newLine);
}
} else if (algo.equals("e5")) {
for (int i = 1; i <= Integer.parseInt(args[2]); i++) {
int[] x = new int[(int) Math.pow(10, i)];
long start = System.currentTimeMillis();
// You can take a new array here, I am just running it for x here
example5(x, x);
long end = System.currentTimeMillis();
double totalTime = Math.log10(end - start);
fw.write(String.valueOf(totalTime));
String newLine = System.getProperty("line.separator");
fw.write(newLine);
}
}
fw.close();
}
public static double[] prefixAverage1(double[] x) {
int n = x.length;
double[] a = new double[n];
for (int j = 0; j < n; j++) {
double total = 0;
for (int i = 0; i <= j; i++) {
total += x[i];
a[j] = total / (j + 1);
}
}
return a;
}
public static double[] prefixAverage2(double[] x) {
int n = x.length;
double[] a = new double[n];
double total = 0;
for (int j = 0; j < n; j++) {
total += x[j];
a[j] = total / (j + 1);
}
return a;
}
public static int example1(int[] arr) {
int n = arr.length, total = 0;
for (int j = 0; j < n; j++)
total += arr[j];
return total;
}
public static int example2(int[] arr) {
int n = arr.length, total = 0;
for (int j = 0; j < n; j += 2)
total += arr[j];
return total;
}
public static int example3(int[] arr) {
int n = arr.length, total = 0;
for (int j = 0; j < n; j++)
for (int k = 0; k <= j; k++)
total += arr[j];
return total;
}
public static int example4(int[] arr) {
int n = arr.length, prefix = 0, total = 0;
for (int j = 0; j < n; j++) {
prefix += arr[j];
total += prefix;
}
return total;
}
public static int example5(int[] first, int[] second) {
int n = first.length, count = 0;
for (int i = 0; i < n; i++) {
int total = 0;
for (int j = 0; j < n; j++)
for (int k = 0; k <= j; k++)
total += first[k];
if (second[i] == total) count++;
}
return count;
}
}

Step by step
Solved in 2 steps with 1 images

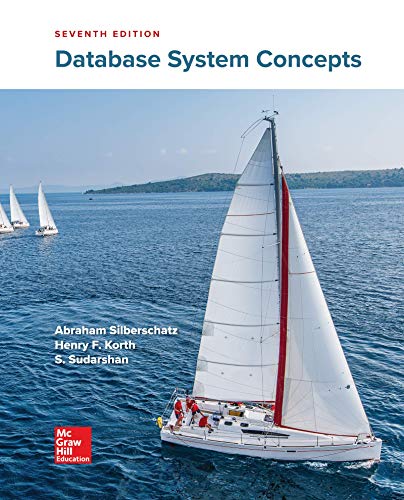
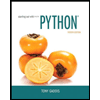
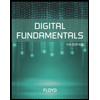
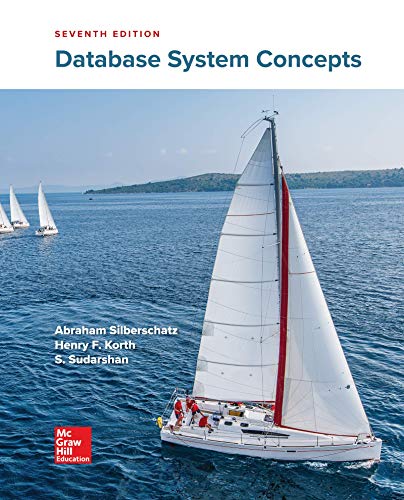
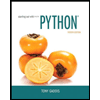
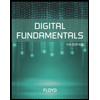
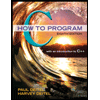
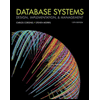
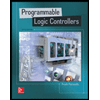