Two of the menu options (#2 and #3) are unfinished, and you need to complete the code necessary to write to and read from a binary file. You will need to read through the code to find the functions that are called by these options, and then supply the missing code. How do I get menu 2 and 3 to display properly?
Two of the menu options (#2 and #3) are unfinished, and you need to complete the code necessary to write to and read from a binary file. You will need to read through the code to find the functions that are called by these options, and then supply the missing code. How do I get menu 2 and 3 to display properly?
the .cpp and .h have the information:
.cpp file:
// Corporate Sales Data Output
using namespace std;
#include <iostream>
#include <fstream>
#include <stdlib.h>
#include "HeaderClassActivity1.h"
int main() {
Sales qtrSales[NUM_QTRS];
while (true) {
cout << "Select an option: " << endl;
cout << "1. Input data" << endl;
cout << "2. Write data to file" << endl;
cout << "3. Read data from file" << endl;
cout << "4. Display sales" << endl;
cout << "99. End
int menuItem;
cin >> menuItem;
if (menuItem == 1) inputData(qtrSales);
if (menuItem == 2) {
cout << "Enter filename: ";
string filename;
cin >> filename;
writeToFile(qtrSales, NUM_QTRS, filename);
}
if (menuItem == 3) {
cout << "Enter filename: ";
string filename;
cin >> filename;
readFromFile(qtrSales, NUM_QTRS, filename);
}
if (menuItem == 4) displaySales(qtrSales, NUM_QTRS);
if (menuItem == 99) return 0;
}
}
void inputData(Sales qtrSales[]) {
string divs[] = { "East", "West", "North", "South" };
int idx = 0;
for (string div : divs) {
for (int i = 0; i < 4; i++) {
cout << "Enter sales for " << div << " division, quarter " << i + 1 << ": ";
//cin >> qtrSales[idx].sales;
// We are faking data entry here for test purposes!
qtrSales[idx].sales = (i + 1) * 16.4 * RAND_MAX / rand();
cout << qtrSales[idx].sales << endl;
strncpy_s(qtrSales[idx].name, div.c_str(), STR_LEN);
qtrSales[idx].qtr = i + 1;
idx++;
}
}
}
void writeToFile(Sales qtrSales[], int size, string filename) {
ofstream outFile;
outFile.open(filename, ios::binary);
for (int i = 0; i < size; i++) {
// Loop through the array of Sales structs, output each to outFile
}
outFile.close();
}
void readFromFile(Sales qtrSales[], int size, string filename) {
ifstream inFile;
inFile.open(filename, ios::binary);
for (int i = 0; i < size; i++) {
// Read in each Sales struct from inFile to the Sales struct array
}
inFile.close();
}
HeaderClassActivity1.h file:
#pragma once
const int NUM_QTRS = 16;
const int STR_LEN = 6;
struct Sales {
char name[STR_LEN];
int qtr;
double sales;
};
void inputData(Sales qtrSales[]);
void writeToFile(Sales qtrSales[], int size, string filename);
void readFromFile(Sales qtrSales[], int size, string filename);
void displaySales(Sales qtrSales[], int size);
void displaySales(Sales qtrSales[], int size) {
for (int i = 0; i < size; i++) {
cout << "Division: " << qtrSales[i].name << " quarter " << qtrSales[i].qtr << " sales: " << qtrSales[i].sales << endl;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

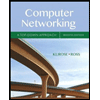
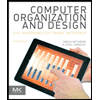
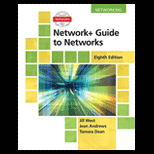
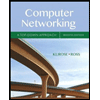
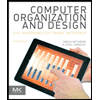
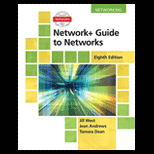
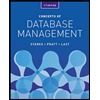
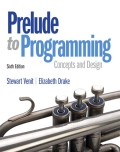
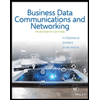