Using two classes, create a Java program that can convert a Fahrenheit temperature to Celsius and Kelvin. Temperature Class (Temperature.java) One field, a double, named fTemp. • One constructor that accepts one argument. o The constructor should assign the value of this argument to fTemp. A method named toCelsius that returns the temperature (value of fTemp) in Celsius. F to C conversion: (fTemp - 32) * (5/9) • A method named tokelvin that returns the temperature (value of fTemp) in Kelvin. F to K conversion: (fTemp + 459.67) * (5/9) Neither the toCelsius or tokelvin methods should alter the value of the fTemp field. TemperatureDemo Class (TemperatureDemo.java) This is the class that will demo a Temperature object; thus, it contains the main method. In the main method: Prompt the user to enter a temperature in Fahrenheit. Construct an instance of your Temperature object o The user's input value is passed as the argument to the constructor Using the Temperature object's toCelsius, and tokelvin methods, print: o The temperature in Celsius. o The temperature in Kelvin. o All output should be rounded to 2 decimal places. Be sure to use comments to document your code.
Using two classes, create a Java program that can convert a Fahrenheit temperature to Celsius and Kelvin. Temperature Class (Temperature.java) One field, a double, named fTemp. • One constructor that accepts one argument. o The constructor should assign the value of this argument to fTemp. A method named toCelsius that returns the temperature (value of fTemp) in Celsius. F to C conversion: (fTemp - 32) * (5/9) • A method named tokelvin that returns the temperature (value of fTemp) in Kelvin. F to K conversion: (fTemp + 459.67) * (5/9) Neither the toCelsius or tokelvin methods should alter the value of the fTemp field. TemperatureDemo Class (TemperatureDemo.java) This is the class that will demo a Temperature object; thus, it contains the main method. In the main method: Prompt the user to enter a temperature in Fahrenheit. Construct an instance of your Temperature object o The user's input value is passed as the argument to the constructor Using the Temperature object's toCelsius, and tokelvin methods, print: o The temperature in Celsius. o The temperature in Kelvin. o All output should be rounded to 2 decimal places. Be sure to use comments to document your code.
Chapter3: Using Methods, Classes, And Objects
Section: Chapter Questions
Problem 13PE
Related questions
Question
Could you please help me with this program ??

Transcribed Image Text:Using two classes, create a Java program that can convert a Fahrenheit temperature to Celsius and
Kelvin.
Temperature Class (Temperature.java)
One field, a double, named fTemp.
• One constructor that accepts one argument.
o The constructor should assign the value of this argument to fTemp.
• A method named toCelsius that returns the temperature (value of fTemp) in Celsius.
F to C conversion: (fTemp - 32) * (5/9)
• A method named toKelvin that returns the temperature (value of fTemp) in Kelvin.
F to K conversion: (fTemp + 459.67) * (5/9)
Neither the toCelsius or tokelvin methods should alter the value of the fTemp field.
TemperatureDemo Class (TemperatureDemo.java)
This is the class that will demo a Temperature object; thus, it contains the main method.
In the main method:
Prompt the user to enter a temperature in Fahrenheit.
Construct an instance of your Temperature object
o The user's input value is passed as the argument to the constructor
• Using the Temperature object's toCelsius, and tokelvin methods, print:
o The temperature in Celsius.
o The temperature in Kelvin.
o All output should be rounded to 2 decimal places.
Be sure to use comments to document your code.
Sample Input/Output
Please enter the Fahrenheit temperature: 98.6
Celsius Temperature: 37.00
Kelvin Temperature: 310.15
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
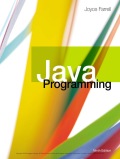
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
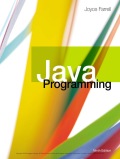
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT