want to add update function .c and .h class in that code
i want to add update function .c and .h class in that code
Menu
1. Create inventory
2. Update inventory
3. Search vaccine
4. Display vaccine
Search.h : Copy the below code and save as Search.h file.
#ifndef SEARCH_H_INCLUDED
#define SEARCH_H_INCLUDED
int search_vaccine();
#endif
Display.h : Copy the below code and save as Display.h file.
#ifndef DISPLAY_H_INCLUDED
#define DISPLAY_H_INCLUDED
void display_vaccine();
#endif
Create.h : Copy the below code and save as Create.h file.
#ifndef CREATE_H_INCLUDED
#define CREATE_H_INCLUDED
void create_inventory();
#endif
Create.c :
#include<stdio.h>
#include "Create.h"
void create_inventory()
{
int option;
char vaccName[15];
char vaccCode[2];
char country[15];
int qty;
float populaion;
FILE* infile;
infile = fopen("Vaccine.txt", "w");
if (infile == NULL)
{
printf("Vaccine.txt file not found\n");
}
option = 1;
while (option != 0)
{
printf("Enter Vaccine Name : ");
scanf("%s", vaccName);
printf("Enter Vaccine Code : ");
scanf("%s", vaccCode);
printf("Enter Country : ");
scanf("%s", country);
printf("Enter Dosage Required : ");
scanf("%d", &qty);
printf("Enter Population Covered : ");
scanf("%f", &populaion);
fprintf(infile, "%15s %2s %15s %d %3.2f\n", vaccName, vaccCode, country, qty, populaion);
printf("\nEnter 1 to continue and 0 to exit : ");
scanf("%d", &option);
if (option == 0)
break;
}
fclose(infile);
}
Display.c:
#include "Display.h"
#include<stdio.h>
void display_vaccine()
{
char vaccName[15];
char vaccCode[2];
char country[15];
int qty;
float populaion;
FILE* infile;
infile = fopen("dist.txt", "r");
if (infile == NULL)
{
printf("Vaccine.txt file not found\n");
}
printf("%15s\t%2s\t%15s\t%6s\t%10s\n", "Vaccine Name", "Vaccine Code", "Country", "Dosage", "Population");
// Reading the file
while (fscanf(infile, "%s %s %s %d %f\n", vaccName, vaccCode, country, &qty, &populaion) != EOF)
{
printf("%15s\t%2s\t%15s\t%d\t%3.2f\n", vaccName, vaccCode, country, qty, populaion);
}
fclose(infile);
}
Search.c:
#include "Search.h"
#include<stdio.h>
int search_vaccine()
{
char vaccName[15];
char vaccCode[2];
char country[15];
int qty;
float populaion;
FILE* infile;
char vcode[2];
char temp[2];
int value;
infile = fopen("Vaccine.txt", "r");
printf("Enter Vaccine Code to Search : ");
scanf("%s", vcode);
if (infile == NULL) // checking for file existence
{
printf("Vaccine.txt file not found\n");
}
strcpy(temp, vcode);
while (fscanf(infile, "%s %s %s %d %f\n", vaccName, vaccCode, country, &qty, &populaion) != EOF)
{
if (vaccCode[0] == temp[0] && vaccCode[1] == temp[1])
{
printf("%15s\t%2s\t%15s\t%6s\t%10s\n", "Vaccine Name", " Vaccine Code", "Country", "Dosage", "Population");
printf("%15s\t%2s\t%15s\t%d\t%3.2f\n", vaccName, vaccCode, country, qty, populaion);
}
}
fclose(infile);
}
TestMain.c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include "Search.h"
#include "Display.h"
#include "Create.h"
struct vacc {
char vaccName[15];
char vaccCode[2];
char country[15];
int qty;
float population;
}v[10];
// Function Declarations
void update_vacc_qty();
int main()
{
int ch;
do {
printf("\n 1:create inventory");
printf("\n 2: display vaccine info ");
printf("\n 3: search vaccine");
printf("\n enter your choice (0 to exit):");
scanf("%d", &ch);
switch (ch)
{
case 1: create_inventory();
break;
case 2: display_vaccine();
break;
case 3: search_vaccine();
break;
default: break;
}
} while (ch != 0);
return 0;
}
void update_vacc_qty()
{
int t;
int option;
char vaccName[15];
char vaccCode[2];
char country[15];
int qty;
float population;
FILE* infile;
infile = fopen("Vaccine.txt", "w");
if (infile == NULL)
{
printf("dist.txt file not found\n");
}
option = 1;
while (option != 0)
{
printf("Enter Vaccine Name : ");
scanf("%s", vaccName);
printf("Enter Vaccine Code : ");
scanf("%s", vaccCode);
printf("Enter Country : ");
scanf("%s", country);
printf("Enter Dosage Required : ");
scanf("%d", &qty);
printf("Enter Population Covered : ");
scanf("%f", &population);
fprintf(infile, "%s %s %s %d %3.2f\n", vaccName, vaccCode, country, qty, population);
printf("\nEnter 1 to continue and 0 to exit : ");
scanf("%d", &option);
if (option == 0)
fclose(infile);
}
{
printf("dist.txt file not found\n");
}
t = 0;
while (fscanf(infile, "%s %s %s %d %f\n", vaccName, vaccCode, country, &qty, &population) != EOF)
{
//printf("%s",vaccName);
strcpy(v[t].vaccName, vaccName);
strcpy(v[t].vaccCode, vaccCode);
strcpy(v[t].country, country);
v[t].qty = qty;
v[t].population = population;
t++;
}
struct vacc temp;
for (int i = 0; i < t - 1; i++)
{
for (int j = 0; j < (t - 1 - i); j++)
{
if (v[j].qty < v[j + 1].qty)
{
temp.qty = v[j].qty;
v[j].qty = v[j + 1].qty;
v[j + 1].qty = temp.qty;
}
}
}
printf("%15s\t%2s\t%15s\t%6s\t%10s\n", "Vaccine Name", "Vaccine Code", "Country", "Dosage", "Population");
for (int i = 0;i < t; i++) {
printf("%15s\t%13s\t%15s\t%d\t%3.2f\n", v[i].vaccName, v[i].vaccCode, v[i].country, v[i].qty, v[i].population);
}
fclose(infile);
}


Step by step
Solved in 2 steps

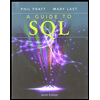
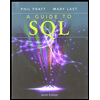