We want the program to use a DEQueue object to create a sorted list of names. The program gives a user the following three options to choose from – insert, delete, and quit. If the user selects ‘insert’, the program should accept a name (String type) from the user and insert it into the deque in a sorted manner. If the user selects ‘delete’, the program should accept a name (String type) from the user and delete that name from the deque. Display the contents of deque after each insert/delete operation. The program continues to prompt the user for options until the user chooses to quit. You have to implement the insert and delete operations. *Using a skeleton
We want the program to use a DEQueue object to create a sorted list of names. The program gives a user the following three options to choose from – insert, delete, and quit. If the user selects ‘insert’, the program should accept a name (String type) from the user and insert it into the deque in a sorted manner. If the user selects ‘delete’, the program should accept a name (String type) from the user and delete that name from the deque. Display the contents of deque after each insert/delete operation. The program continues to prompt the user for options until the user chooses to quit. You have to implement the insert and delete operations. *Using a skeleton
Chapter2: Using Data
Section: Chapter Questions
Problem 14RQ
Related questions
Question
We want the
accept a name (String type) from the user and insert it into the deque in a sorted manner. If the
user selects ‘delete’, the program should accept a name (String type) from the user and delete
that name from the deque. Display the contents of deque after each insert/delete operation. The
program continues to prompt the user for options until the user chooses to quit. You have to
implement the insert and delete operations.
*Using a skeleton
![A
*AppPart2.java X Dequelnterface.java
1 package assign4DEQ;
2
3 import java.util.Scanner;
4
50 /**
6 * COSC 2100 - Spring 2023
7 * Assignment#4
8
9 * @author Dr.. edited by Julianne Browne
*/
10
11
12 public class AppPart2 {
13
140
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
* Create a sorted list of names
236
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51 }
System.out.print("1: insert, ");
System.out.print("2: delete, ");
System.out.println("3: quit");
public static void main(String[] args) {
DEQueue<String> names = new DEQueue<String>();
Scanner scan = new Scanner(System.in);
int operation;
// indicates user's choice of operation
boolean keepGoing = true; //to help with quit option
while (keepGoing) {
System.out.print("\nChoose an operation - "');
if (scan.hasNextInt())
else{
operation = scan.nextInt ();
return;
*DEQueue.java
}
switch (operation) {
case 1:
System.out.println("Error: you must enter an integer.");
System.out.println("Terminating test.");
case 3: // quit
keepGoing
//TODO (6) Implement the insert operation
break;
case 2: //TODO (7) Implement the delete operation
break;
break;
default:
*DLLNode.java
false;
System.out.println("Goodbye!!");
=
return;
}//end of switch
}//end of while
scan.close();
QueueUnderflowException.java
System.out.println("Error in operation choice. Terminating test.");
Writable
Smart Insert
9:16:143
0
U
—](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fffb11e9a-c047-4f4c-b0a7-ca87c58eaccc%2F83704e47-b28c-4904-8738-e8b1c8e4555b%2Fcldxfn_processed.png&w=3840&q=75)
Transcribed Image Text:A
*AppPart2.java X Dequelnterface.java
1 package assign4DEQ;
2
3 import java.util.Scanner;
4
50 /**
6 * COSC 2100 - Spring 2023
7 * Assignment#4
8
9 * @author Dr.. edited by Julianne Browne
*/
10
11
12 public class AppPart2 {
13
140
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
* Create a sorted list of names
236
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51 }
System.out.print("1: insert, ");
System.out.print("2: delete, ");
System.out.println("3: quit");
public static void main(String[] args) {
DEQueue<String> names = new DEQueue<String>();
Scanner scan = new Scanner(System.in);
int operation;
// indicates user's choice of operation
boolean keepGoing = true; //to help with quit option
while (keepGoing) {
System.out.print("\nChoose an operation - "');
if (scan.hasNextInt())
else{
operation = scan.nextInt ();
return;
*DEQueue.java
}
switch (operation) {
case 1:
System.out.println("Error: you must enter an integer.");
System.out.println("Terminating test.");
case 3: // quit
keepGoing
//TODO (6) Implement the insert operation
break;
case 2: //TODO (7) Implement the delete operation
break;
break;
default:
*DLLNode.java
false;
System.out.println("Goodbye!!");
=
return;
}//end of switch
}//end of while
scan.close();
QueueUnderflowException.java
System.out.println("Error in operation choice. Terminating test.");
Writable
Smart Insert
9:16:143
0
U
—
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
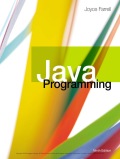
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
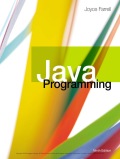
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT