WHAT CAN I USE TO SORT WITHOUT USING PANDAS, LAMBDAS OR ITEMGETTER? import csv manufacturerList = [] priceList = [] serviceList = [] with open('ManufacturerList.csv', 'r') as man_list: ml = csv.reader(man_list, delimiter=',') for row in ml: manufacturerList.append(row) print(row) with open('PriceList.csv', 'r') as price_list: pl = csv.reader(price_list, delimiter=',') for row in pl: priceList.append(row) print(row) with open('ManufacturerList.csv', 'r') as service_list: sl = csv.reader(service_list, delimiter=',') for row in sl: serviceList.append(row) print(row) new_mfl = (sorted(manufacturerList, key='id')) new_prl = (sorted(priceList, key='None')) new_sdl = (sorted(serviceList, key='None')) for x in range(0, len(new_mfl)): new_mfl[x].append(priceList[x][1]) for x in range(0, len(new_mfl)): new_mfl[x].append(serviceList[x][1]) new_list = new_mfl inventoryList = (sorted(list, key=1)) with open('FullInventory.csv', 'w') as inventory_file: csv_writer = csv.writer(inventory_file) for i in range(0, len(inventoryList)): csv_writer.writerow(inventoryList[i]) item_type = inventoryList tower_list = [] laptop_list = [] phone_list = [] for i in range(0, len(item_type)): if item_type[i][2] == 'tower': tower_list.append(item_type[i]) elif item_type[i][2] == 'phone': phone_list.append(item_type[i]) elif item_type[i][2] == 'laptop': laptop_list.append(item_type[i]) with open('LaptopInventory.csv', 'w') as new_file1: laptop_writer = csv.writer(new_file1) for i in range(0, len(laptop_list)): laptop_writer.writerow(laptop_list[i]) with open('PhoneInventory.csv', 'w') as new_file2: phone_writer = csv.writer(new_file2) for i in range(0, len(phone_list)): phone_writer.writerow(phone_list[i]) with open('TowerInventory.csv', 'w') as new_file3: tower_writer = csv.writer(new_file3) for i in range(0, len(tower_list)): tower_writer.writerow(tower_list[i]) damaged_list = [] for i in range(0, len(item_type)): if item_type[i][3] == 'damaged': damaged_list.append(item_type[i]) damaged_list = (sorted(damaged_list, key=row[3], reverse=True)) with open('DamagedInventory.csv', 'w') as new_file4: damaged_writer = csv.writer(new_file4) for i in range(0, len(damaged_list)): damaged_writer.writerow(damaged_list[i]) user_man = str(input('Enter manufacturer name: ')) user_type = str(input('Enter your item type: ')) user_item = [] while user_man != 'q': for i in range(0, len(new_list)): if user_man in new_list[i] and user_type in new_list[i]: user_item.append(new_list[i]) if len(user_item) != 0: user_item = sorted(user_item, key=row[4], reverse=True) print('Your item is ', user_item[0]) else: print('No such item in inventory') user_man = str(input('Enter your manufacturer or q to exit: ')) user_type = str(input('Enter your item type: '))
WHAT CAN I USE TO SORT WITHOUT USING PANDAS, LAMBDAS OR ITEMGETTER?
import csv
manufacturerList = []
priceList = []
serviceList = []
with open('ManufacturerList.csv', 'r') as man_list:
ml = csv.reader(man_list, delimiter=',')
for row in ml:
manufacturerList.append(row)
print(row)
with open('PriceList.csv', 'r') as price_list:
pl = csv.reader(price_list, delimiter=',')
for row in pl:
priceList.append(row)
print(row)
with open('ManufacturerList.csv', 'r') as service_list:
sl = csv.reader(service_list, delimiter=',')
for row in sl:
serviceList.append(row)
print(row)
new_mfl = (sorted(manufacturerList, key='id'))
new_prl = (sorted(priceList, key='None'))
new_sdl = (sorted(serviceList, key='None'))
for x in range(0, len(new_mfl)):
new_mfl[x].append(priceList[x][1])
for x in range(0, len(new_mfl)):
new_mfl[x].append(serviceList[x][1])
new_list = new_mfl
inventoryList = (sorted(list, key=1))
with open('FullInventory.csv', 'w') as inventory_file:
csv_writer = csv.writer(inventory_file)
for i in range(0, len(inventoryList)):
csv_writer.writerow(inventoryList[i])
item_type = inventoryList
tower_list = []
laptop_list = []
phone_list = []
for i in range(0, len(item_type)):
if item_type[i][2] == 'tower':
tower_list.append(item_type[i])
elif item_type[i][2] == 'phone':
phone_list.append(item_type[i])
elif item_type[i][2] == 'laptop':
laptop_list.append(item_type[i])
with open('LaptopInventory.csv', 'w') as new_file1:
laptop_writer = csv.writer(new_file1)
for i in range(0, len(laptop_list)):
laptop_writer.writerow(laptop_list[i])
with open('PhoneInventory.csv', 'w') as new_file2:
phone_writer = csv.writer(new_file2)
for i in range(0, len(phone_list)):
phone_writer.writerow(phone_list[i])
with open('TowerInventory.csv', 'w') as new_file3:
tower_writer = csv.writer(new_file3)
for i in range(0, len(tower_list)):
tower_writer.writerow(tower_list[i])
damaged_list = []
for i in range(0, len(item_type)):
if item_type[i][3] == 'damaged':
damaged_list.append(item_type[i])
damaged_list = (sorted(damaged_list, key=row[3], reverse=True))
with open('DamagedInventory.csv', 'w') as new_file4:
damaged_writer = csv.writer(new_file4)
for i in range(0, len(damaged_list)):
damaged_writer.writerow(damaged_list[i])
user_man = str(input('Enter manufacturer name: '))
user_type = str(input('Enter your item type: '))
user_item = []
while user_man != 'q':
for i in range(0, len(new_list)):
if user_man in new_list[i] and user_type in new_list[i]:
user_item.append(new_list[i])
if len(user_item) != 0:
user_item = sorted(user_item, key=row[4], reverse=True)
print('Your item is ', user_item[0])
else:
print('No such item in inventory')
user_man = str(input('Enter your manufacturer or q to exit: '))
user_type = str(input('Enter your item type: '))

Step by step
Solved in 2 steps

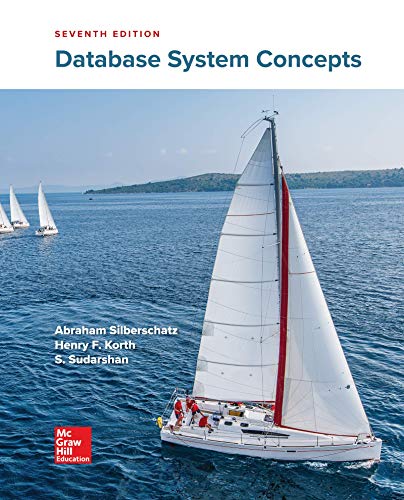
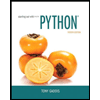
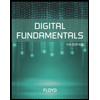
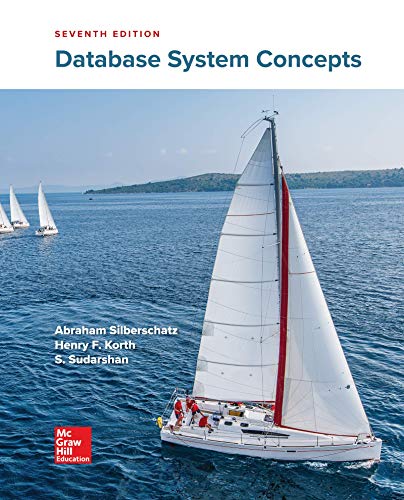
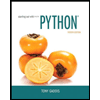
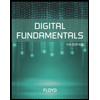
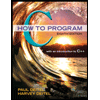
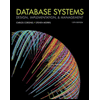
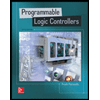