Write a C++ program to ask the number of item types, then create an array list to obtain input from the user and display the item type details using iterator loop
Write a C++ program to ask the number of item types, then create an array list to obtain input from the user and display the item type details using iterator loop
Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement.
The class named ItemType has the following private member variables.
Data Type | Variable Name |
string | name |
double | deposit |
double | costPerDay |
Define the following member function inside the class ItemTypeBO.
Function Name | Description |
void display (list<ItemType> item) | This function is used to display the item type details in the following format, "ItemName", "ItemDeposit", "CostPerDay") print the header "Item type details are:" inside the display function. |
In the main method, obtain item type details from the user in the console and store them in a list. Display the ItemType details in a tabular form by calling the display method. If the number of item types entered is less than or equal to zero print 'Invalid number'.
Problem constraints:
Use < iterator> containers to display the values.
Use the insert() method to insert the values.
Input and Output format:
Refer sample input and output for formatting specifications.
Display the item deposit and cost per day with respect to two decimal places.
[All text in bold corresponds to input and the rest corresponds to output]
Enter the number of item type:
2
Enter details of item type 1
Enter the item type name:
Electronics
Enter the deposit:
20000
Enter cost per day:
200
Enter details of item type 2
Enter the item type name:
Furniture
Enter the deposit:
30000
Enter cost per day:
300
Item type details are:
ItemName ItemDeposit CostPerDay
Electronics 20000.00 200.00
Furniture 30000.00 300.00
Sample Input and Output 2:
Enter the number of itemtypes:
-5
Invalid number
--------------STRICTLY USE BELOW TEMPLATE WHILE MAKING SOLUTION-------
MAIN.CPP
#include <iostream>
|
ItemtypeBo.cpp
#include <iostream> #include<string.h> #include<list> #include<stdio.h> #include"ItemType.cpp" using namespace std; class ItemTypeBO { public: void display(list<ItemType> item){ // fill the code } }; |
ItemType.cpp
#ifndef ITEMTYPE_H #define ITEMTYPE_H #include <iostream> #include<string.h> #include<list> #include<stdio.h> using namespace std; class ItemType { private: string name; double deposit,costPerDay; public : ItemType() { } ItemType(string name,double deposit,double costPerDay) { this->name=name; this->deposit=deposit; this->costPerDay=costPerDay; } void setName(string name) { this->name=name; } void setDeposit(double deposit) { this->deposit=deposit; } void setCostPerDay(double costperday1) { this->costPerDay=costperday1; } string getItemName() { return name; } double getItemDeposit() { return deposit; } double getCostPerDay() { return costPerDay; } }; #endif |

Step by step
Solved in 2 steps

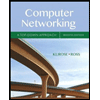
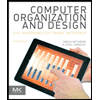
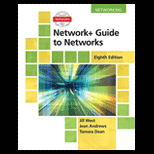
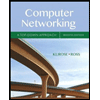
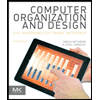
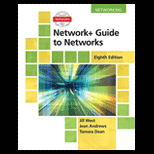
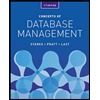
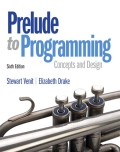
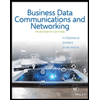