Write a program Java to convert an integer to a string containing its hex representation, then print the result. Hex, short for Hexadecimal, is the base 16 representation, using all the digits 0-9, and in addition the letters A (10), B (11), C (12), D (13), E (14), and F (15). So numbers less than 10 are written as usual, numbers 10-15 are written with the corresponding letter, 16 is written "10", 17 (which is 16+1) is written "11", 26 (16+10) is written "1A", 27 is "1B", 32 is "20", 33 is "21", and so on. Your program must include a recursive method public static String intToHex(int n) intToHex must return the hex representation of its given integer, and must have a recursive implementation similar to the intToString example in the lecture material. For example, intToHex(1) must return "1", intToHex(12) must return "C", intToHex(123) must return "7B", intToHex(1234) must return "4D2", intToHex(12345) must return "3039", intToHex(123456) must return "1E240", and of course intToHex(65535) must return "FFFF". Your program must repeatedly prompt until the user enters a negative number, at which point your program must end. enter a number: 65535 FFFF enter a number: 255 FF enter a number: 256 100 enter a number: -1
Write a
Hex, short for Hexadecimal, is the base 16 representation, using all the digits 0-9, and in addition the letters A (10), B (11), C (12), D (13), E (14), and F (15). So numbers less than 10 are written as usual, numbers 10-15 are written with the corresponding letter, 16 is written "10", 17 (which is 16+1) is written "11", 26 (16+10) is written "1A", 27 is "1B", 32 is "20", 33 is "21", and so on.
Your program must include a recursive method
public static String intToHex(int n)
intToHex must return the hex representation of its given integer, and must have a recursive implementation similar to the intToString example in the lecture material.
For example, intToHex(1) must return "1", intToHex(12) must return "C", intToHex(123) must return "7B", intToHex(1234) must return "4D2", intToHex(12345) must return "3039", intToHex(123456) must return "1E240", and of course intToHex(65535) must return "FFFF".
Your program must repeatedly prompt until the user enters a negative number, at which point your program must end.
enter a number: 65535
FFFF
enter a number: 255
FF
enter a number: 256
100
enter a number: -1

Step by step
Solved in 4 steps with 3 images

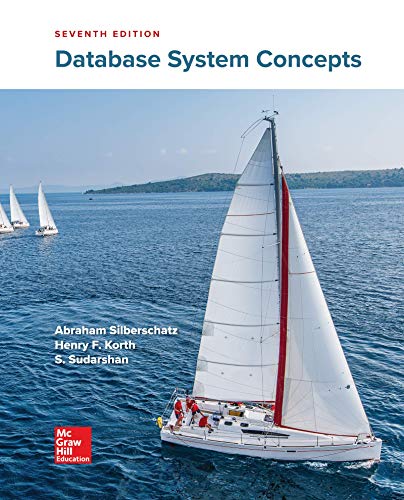
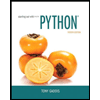
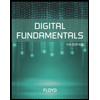
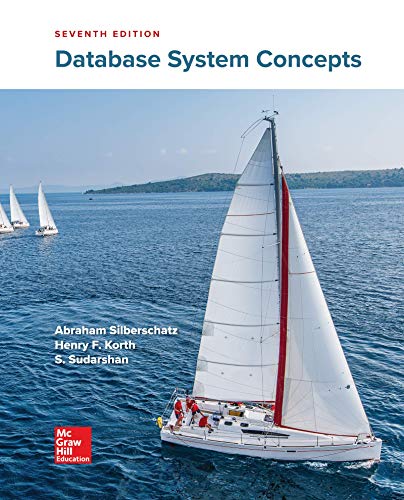
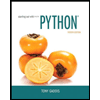
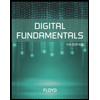
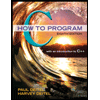
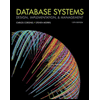
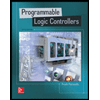